Unlock new levels of connectivity and control as our port expander technology provides you with the tools to expand your I/O capabilities, effortlessly manage data flow, and improve the efficiency of your electronic systems
A
A
Hardware Overview
How does it work?
Expand 4 Click is based on the TPIC6A595, a monolithic, high-voltage, high-current power logic 8-bit shift register from Texas Instruments. The TPIC6A595 contains a built-in voltage clamp on the outputs for inductive transient protection. Each output is a low-side, open-drain DMOS transistor with output ratings of 50V and a 350mA continuous sink current capability, featuring an independent chopping current-limiting circuit to prevent damage in the case of a short circuit. This Click board™ is designed for use in systems that require relatively high load power, such as relays, solenoids, and other medium-current or high-voltage loads. This TPIC6A595 contains an 8-bit serial-in, parallel-out shift register that feeds an 8-bit, D-type storage register. Data transfers through the shift and storage register on the rising edge
of the shift register clock (SPI clock pin on the mikroBUS™ socket) and the register clock (CS clock pin on the mikroBUS™ socket), respectively. The storage register transfers data to the output buffer when the shift register clear pin is set to a high logic state. This function can be done via the existing CLR jumper by placing it in the appropriate VCC or CLR position. In this way, it is possible to permanently bind this function so that the storage register always transfers data to the output buffer by setting the jumper to the VCC position or controlled digitally by setting the jumper to the CLR position. This way, controlling the shift-register-clear via the RST pin of the mikroBUS™ socket marked as CLR is possible. The input shift register is cleared when CLR is in a low logic state. In the same way, it is possible to
manage the outputs of the port expander, eight pins above the mikroBUS™ socket (D0-D7), using EN jumper, more precisely define the output management mode, constantly ON or digital control over them through PWM pin of the mikroBUS™ socket marked as EN. When the EN pin is held in a high logic state, all data in the output buffers is kept low, and all drain outputs are OFF. When EN is LOW, data from the storage register is transparent to the output buffers. This Click board™ can be operated only with a 5V logic voltage level. The board must perform appropriate logic voltage level conversion before using MCUs with different logic levels. Also, it comes equipped with a library containing functions and an example code that can be used as a reference for further development.
Features overview
Development board
PIC32MZ Clicker is a compact starter development board that brings the flexibility of add-on Click boards™ to your favorite microcontroller, making it a perfect starter kit for implementing your ideas. It comes with an onboard 32-bit PIC32MZ microcontroller with FPU from Microchip, a USB connector, LED indicators, buttons, a mikroProg connector, and a header for interfacing with external electronics. Thanks to its compact design with clear and easy-recognizable silkscreen markings, it provides a fluid and immersive working experience, allowing access anywhere and under
any circumstances. Each part of the PIC32MZ Clicker development kit contains the components necessary for the most efficient operation of the same board. In addition to the possibility of choosing the PIC32MZ Clicker programming method, using USB HID mikroBootloader, or through an external mikroProg connector for PIC, dsPIC, or PIC32 programmer, the Clicker board also includes a clean and regulated power supply module for the development kit. The USB Micro-B connection can provide up to 500mA of current, which is more than enough to operate all onboard
and additional modules. All communication methods that mikroBUS™ itself supports are on this board, including the well-established mikroBUS™ socket, reset button, and several buttons and LED indicators. PIC32MZ Clicker is an integral part of the Mikroe ecosystem, allowing you to create a new application in minutes. Natively supported by Mikroe software tools, it covers many aspects of prototyping thanks to a considerable number of different Click boards™ (over a thousand boards), the number of which is growing every day.
Microcontroller Overview
MCU Card / MCU
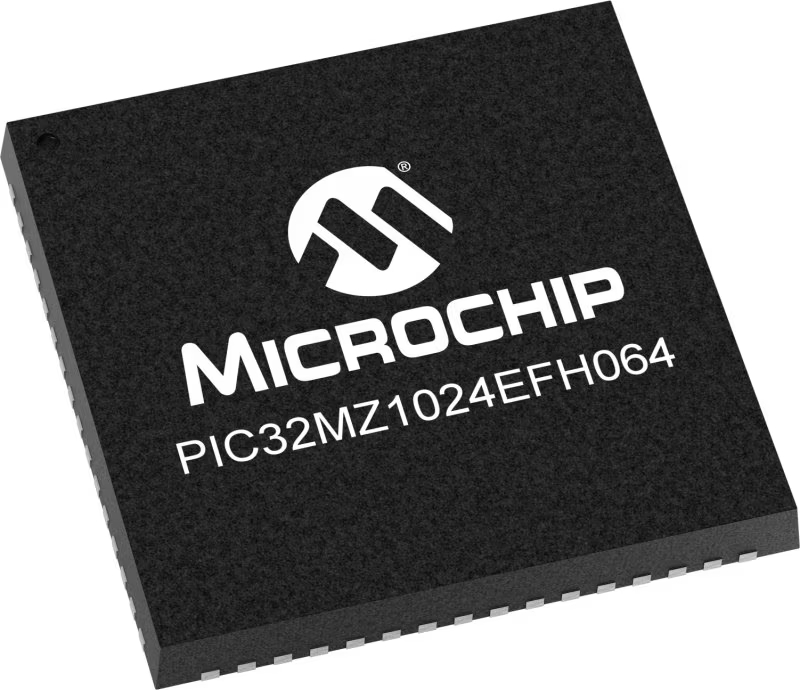
Architecture
PIC32
MCU Memory (KB)
1024
Silicon Vendor
Microchip
Pin count
64
RAM (Bytes)
524288
Used MCU Pins
mikroBUS™ mapper
Take a closer look
Click board™ Schematic
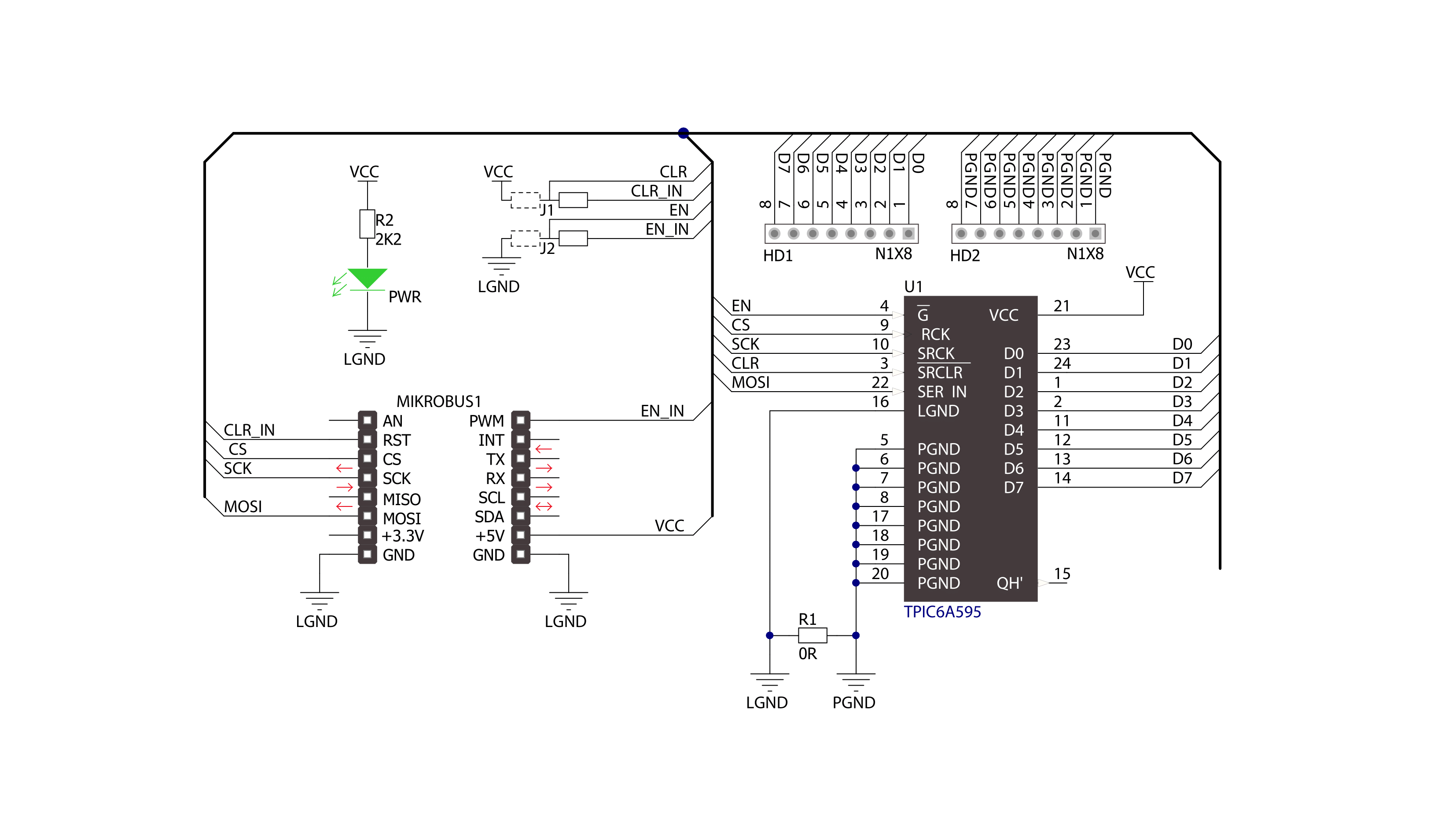
Step by step
Project assembly
Track your results in real time
Application Output via Debug Mode
1. Once the code example is loaded, pressing the "DEBUG" button initiates the build process, programs it on the created setup, and enters Debug mode.
2. After the programming is completed, a header with buttons for various actions within the IDE becomes visible. Clicking the green "PLAY" button starts reading the results achieved with the Click board™. The achieved results are displayed in the Application Output tab.
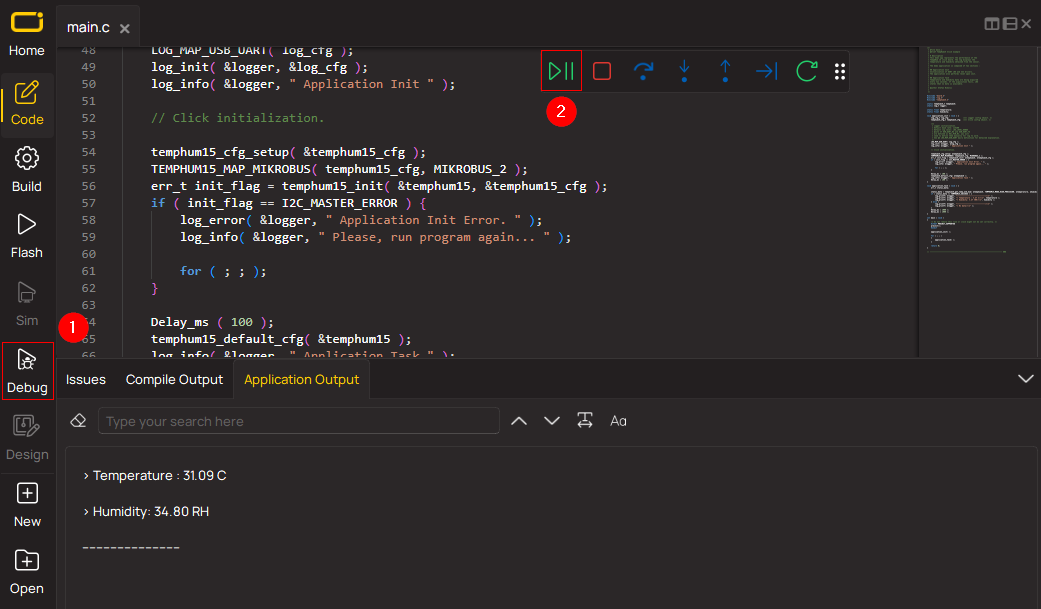
Software Support
Library Description
This library contains API for EXPAND 4 Click driver.
Key functions:
expand4_write_data
- Function write 8-bit data function to TPIC6A595 shift registerexpand4_enable_output
- Function turn on output buffers - set PWM pin lowexpand4_reset
- Function clear input TPIC6A595 shift register.
Open Source
Code example
The complete application code and a ready-to-use project are available through the NECTO Studio Package Manager for direct installation in the NECTO Studio. The application code can also be found on the MIKROE GitHub account.
/*!
* \file
* \brief Expand4 Click example
*
* # Description
* Example demonstrates use of Expand 4 Click board.
*
* The demo application is composed of two sections :
*
* ## Application Init
* Initialization driver enable's - Clear TPIC6A595 register and start write log.
*
* ## Application Task
* This is a example which demonstrates the use of Expand 4 Click board.
* In this example, the LED pin mask is transferred via SPI bus,
* LEDs connected to D0-D7 pins are lit accordingly by turning ON LEDs from D0 to D7 for 3 sec.
* Results are being sent to the Usart Terminal where you can track their changes.
* All data logs on usb uart for aproximetly every 3 sec. when the change pin who is connected.
*
*
* \author MikroE Team
*
*/
// ------------------------------------------------------------------- INCLUDES
#include "board.h"
#include "log.h"
#include "expand4.h"
// ------------------------------------------------------------------ VARIABLES
static expand4_t expand4;
static log_t logger;
// ------------------------------------------------------ APPLICATION FUNCTIONS
void application_init ( void )
{
log_cfg_t log_cfg;
expand4_cfg_t cfg;
/**
* Logger initialization.
* Default baud rate: 115200
* Default log level: LOG_LEVEL_DEBUG
* @note If USB_UART_RX and USB_UART_TX
* are defined as HAL_PIN_NC, you will
* need to define them manually for log to work.
* See @b LOG_MAP_USB_UART macro definition for detailed explanation.
*/
LOG_MAP_USB_UART( log_cfg );
log_init( &logger, &log_cfg );
log_info( &logger, "---- Application Init ----" );
// Click initialization.
expand4_cfg_setup( &cfg );
EXPAND4_MAP_MIKROBUS( cfg, MIKROBUS_1 );
expand4_init( &expand4, &cfg );
expand4_reset( &expand4 );
}
void application_task ( void )
{
uint8_t pin_position;
for ( pin_position = 0; pin_position < 8; pin_position++ )
{
expand4_disable_output( &expand4 );
Delay_ms( 100 );
expand4_turn_on_by_position( &expand4, pin_position );
Delay_ms( 100 );
log_printf( &logger, " D%d", pin_position );
expand4_enable_output( &expand4 );
Delay_ms( 3000 );
}
log_printf( &logger, "\n----------------------------------\n");
}
void main ( void )
{
application_init( );
for ( ; ; )
{
application_task( );
}
}
// ------------------------------------------------------------------------ END