Elevate the performance of your automation projects with our multi-port I/O expander, ensuring seamless and synchronized data exchange among various input and output devices
A
A
Hardware Overview
How does it work?
Expand 13 Click is based on the PI4IOE5V96248, a 48-bit low-voltage translating general-purpose remote I/O expander from Diodes Incorporated. This port expander is a simple solution when additional I/Os are needed while keeping interconnections to a minimum. It is particularly great for system monitoring applications, industrial controllers, and portable equipment. The PI4IOE5V96248 comes in a 6-channel configuration with a built-in level shifting feature that makes it highly flexible in power supply systems where communication between incompatible I/O voltages is required. The PI4IOE5V96248 has low current consumption and includes latched outputs with high current drive capability for directly driving LEDs. All ports of the PI4IOE5V96248 are entirely independent and can
be used as input or output ports without using a control signal for data directions. Input data is transferred from the ports to the MCU in the Read mode, while output data is transmitted to the ports in the Write mode, where every data transmission must consist of a multiple of six bytes. Expand 13 Click communicates with MCU using the standard I2C 2-Wire interface to read data and configure settings with a maximum frequency of 1MHz. Besides, it also allows the choice of the least significant bit of its I2C slave address by positioning the SMD jumpers labeled ADDR SEL to an appropriate position marked as 0 and 1. This way, the PI4IOE5V96248 provides the opportunity of the 64 possible different I2C addresses by positioning the SMD jumper to an appropriate position. In addition to I2C
communication, two GPIO pins connected to the mikroBUS™ socket pins are also used. The Reset pin routed to the RST pin of the mikroBUS™ socket, is used to place the PI4IOE5V96248 registers in their default state, while the interrupt, routed to the INT pin of the mikroBUS™ socket, may be configured as an interrupt to notify the host MCU of incoming data on any port without having to communicate via the I2C-bus. This Click board™ can operate with either 3.3V or 5V logic voltage levels selected via the VCC SEL jumper. This way, both 3.3V and 5V capable MCUs can use the communication lines properly. Also, this Click board™ comes equipped with a library containing easy-to-use functions and an example code that can be used as a reference for further development.
Features overview
Development board
PIC18F57Q43 Curiosity Nano evaluation kit is a cutting-edge hardware platform designed to evaluate microcontrollers within the PIC18-Q43 family. Central to its design is the inclusion of the powerful PIC18F57Q43 microcontroller (MCU), offering advanced functionalities and robust performance. Key features of this evaluation kit include a yellow user LED and a responsive
mechanical user switch, providing seamless interaction and testing. The provision for a 32.768kHz crystal footprint ensures precision timing capabilities. With an onboard debugger boasting a green power and status LED, programming and debugging become intuitive and efficient. Further enhancing its utility is the Virtual serial port (CDC) and a debug GPIO channel (DGI
GPIO), offering extensive connectivity options. Powered via USB, this kit boasts an adjustable target voltage feature facilitated by the MIC5353 LDO regulator, ensuring stable operation with an output voltage ranging from 1.8V to 5.1V, with a maximum output current of 500mA, subject to ambient temperature and voltage constraints.
Microcontroller Overview
MCU Card / MCU
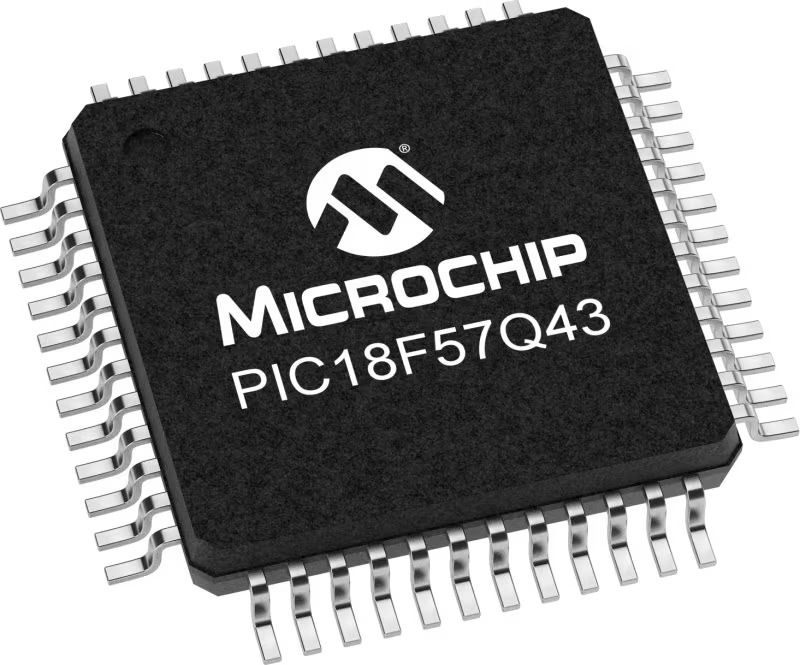
Architecture
PIC
MCU Memory (KB)
128
Silicon Vendor
Microchip
Pin count
48
RAM (Bytes)
8196
You complete me!
Accessories
Curiosity Nano Base for Click boards is a versatile hardware extension platform created to streamline the integration between Curiosity Nano kits and extension boards, tailored explicitly for the mikroBUS™-standardized Click boards and Xplained Pro extension boards. This innovative base board (shield) offers seamless connectivity and expansion possibilities, simplifying experimentation and development. Key features include USB power compatibility from the Curiosity Nano kit, alongside an alternative external power input option for enhanced flexibility. The onboard Li-Ion/LiPo charger and management circuit ensure smooth operation for battery-powered applications, simplifying usage and management. Moreover, the base incorporates a fixed 3.3V PSU dedicated to target and mikroBUS™ power rails, alongside a fixed 5.0V boost converter catering to 5V power rails of mikroBUS™ sockets, providing stable power delivery for various connected devices.
Used MCU Pins
mikroBUS™ mapper
Take a closer look
Schematic
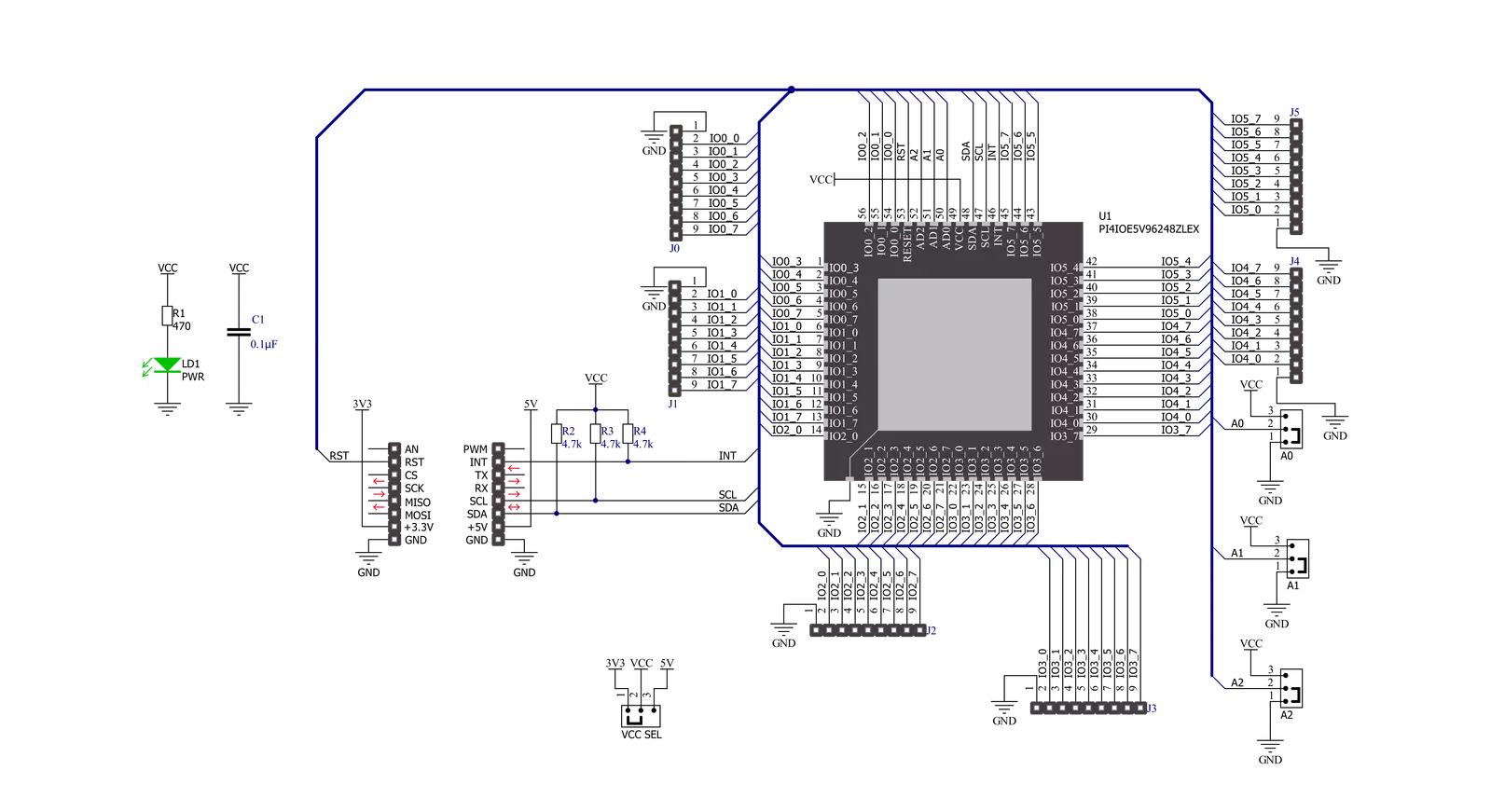
Step by step
Project assembly
Track your results in real time
Application Output via Debug Mode
1. Once the code example is loaded, pressing the "DEBUG" button initiates the build process, programs it on the created setup, and enters Debug mode.
2. After the programming is completed, a header with buttons for various actions within the IDE becomes visible. Clicking the green "PLAY" button starts reading the results achieved with the Click board™. The achieved results are displayed in the Application Output tab.
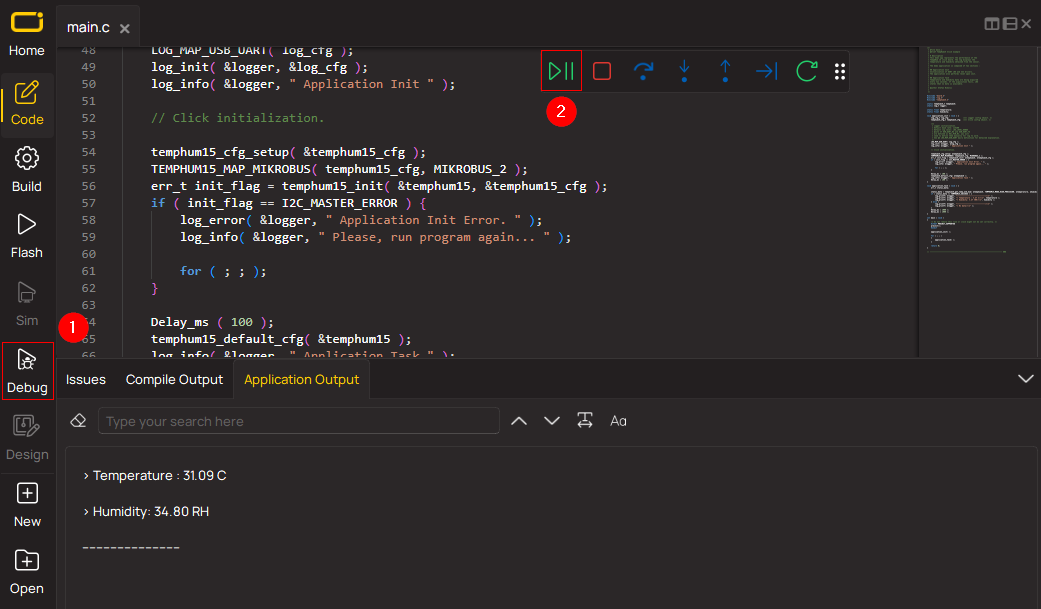
Software Support
Library Description
This library contains API for Expand 13 Click driver.
Key functions:
expand13_enable_device
- This function enables the device by setting the RST pin to high logic stateexpand13_write_all_ports
- This function writes a desired data to all 6 portsexpand13_read_all_ports
- This function reads the state of all 6 ports
Open Source
Code example
This example can be found in NECTO Studio. Feel free to download the code, or you can copy the code below.
/*!
* @file main.c
* @brief Expand13 Click example
*
* # Description
* This example demonstrates the use of Expand 13 click board,
* by writing data to all six ports and then reading back the status of the ports.
*
* The demo application is composed of two sections :
*
* ## Application Init
* Initializes the driver and enables the click board.
*
* ## Application Task
* Sets the pins of all ports and then reads and displays their status on the
* USB UART approximately once per second.
*
* @author Stefan Filipovic
*
*/
#include "board.h"
#include "log.h"
#include "expand13.h"
static expand13_t expand13;
static log_t logger;
void application_init ( void )
{
log_cfg_t log_cfg; /**< Logger config object. */
expand13_cfg_t expand13_cfg; /**< Click config object. */
/**
* Logger initialization.
* Default baud rate: 115200
* Default log level: LOG_LEVEL_DEBUG
* @note If USB_UART_RX and USB_UART_TX
* are defined as HAL_PIN_NC, you will
* need to define them manually for log to work.
* See @b LOG_MAP_USB_UART macro definition for detailed explanation.
*/
LOG_MAP_USB_UART( log_cfg );
log_init( &logger, &log_cfg );
log_info( &logger, " Application Init " );
// Click initialization.
expand13_cfg_setup( &expand13_cfg );
EXPAND13_MAP_MIKROBUS( expand13_cfg, MIKROBUS_1 );
if ( I2C_MASTER_ERROR == expand13_init( &expand13, &expand13_cfg ) )
{
log_error( &logger, " Communication init." );
for ( ; ; );
}
expand13_enable_device ( &expand13 );
log_info( &logger, " Application Task " );
}
void application_task ( void )
{
uint8_t port_value[ 6 ] = { 0 };
uint16_t pin_num = 0;
for ( pin_num = EXPAND13_PIN_0_MASK; pin_num <= EXPAND13_PIN_7_MASK; pin_num <<= 1 )
{
if ( !expand13_get_int_pin ( &expand13 ) )
{
log_printf( &logger, " External input has occurred.\r\n" );
}
memset ( port_value, pin_num, 6 );
expand13_write_all_ports( &expand13, port_value );
expand13_read_all_ports( &expand13, port_value );
for ( uint8_t cnt = EXPAND13_PORT_0; cnt <= EXPAND13_PORT_5; cnt++ )
{
log_printf( &logger, " Status port %d : 0x%.2X\r\n", ( uint16_t ) cnt, ( uint16_t ) port_value[ cnt ] );
}
log_printf( &logger, "\n" );
Delay_ms( 1000 );
}
}
void main ( void )
{
application_init( );
for ( ; ; )
{
application_task( );
}
}
// ------------------------------------------------------------------------ END