Achieve unparalleled data accuracy in your designs with our top-of-the-line ADC
A
A
Hardware Overview
How does it work?
ADC 3 Click is based on the MCP3428, a differential multi-channel low-power, 16-bit ΔΣ A/D converter from Microchip. The MCP3428 contains an input channel selection multiplexer (CH1, CH2, CH3, or CH4), an onboard voltage reference, and an internal oscillator. It performs conversions at rates of 15, 60, or 240 samples per second (12, 14, or 16 bits), depending on user-controllable configuration bit settings, and has a programmable gain amplifier (x1, x2, x4, or x8) which makes it an ideal choice for monitoring extremely low-voltage sensors. All four channels have differential inputs monitoring a full-scale range of 4.096VDC or ±2.048V differentially. The MCP3428 has two conversion modes: Continuous and One-Shot mode. In the Continuous
Conversion mode, the ADC converts the inputs continuously, while in the One-Shot Conversion mode, the MCP3428 converts the input once and stays in the low-power Standby mode until it receives another command for a new conversion. The standby mode reduces current consumption significantly during idle periods. This ADDC can be used for various high-accuracy analog-to-digital data conversion applications where ease of use and low power consumption are significant considerations. ADC 3 Click communicates with an MCU using the standard I2C 2-Wire interface to read data and configure settings, supporting High-Speed Mode up to 3.4MHz. It also has a 7-bit slave address with the first four MSBs fixed to 1101. The address pins, ADR0 and ADR1, are
programmed by the user and determine the value of the last three LSBs of the slave address, allowing up to 8 devices to operate on the same bus segment. The value of these address pins can be set by positioning onboard SMD jumpers labeled as I2C ADR to an appropriate position marked as 0 or 1. This Click board™ can operate with both 3.3V and 5V logic voltage levels selected via the PWR SEL jumper. This way, it is allowed for both 3.3V and 5V capable MCUs to use the communication lines properly. However, the Click board™ comes equipped with a library containing easy-to-use functions and an example code that can be used, as a reference, for further development.
Features overview
Development board
EasyAVR v7 is the seventh generation of AVR development boards specially designed for the needs of rapid development of embedded applications. It supports a wide range of 16-bit AVR microcontrollers from Microchip and has a broad set of unique functions, such as a powerful onboard mikroProg programmer and In-Circuit debugger over USB. The development board is well organized and designed so that the end-user has all the necessary elements in one place, such as switches, buttons, indicators, connectors, and others. With four different connectors for each port, EasyAVR v7 allows you to connect accessory boards, sensors, and custom electronics more
efficiently than ever. Each part of the EasyAVR v7 development board contains the components necessary for the most efficient operation of the same board. An integrated mikroProg, a fast USB 2.0 programmer with mikroICD hardware In-Circuit Debugger, offers many valuable programming/debugging options and seamless integration with the Mikroe software environment. Besides it also includes a clean and regulated power supply block for the development board. It can use a wide range of external power sources, including an external 12V power supply, 7-12V AC or 9-15V DC via DC connector/screw terminals, and a power source via the USB Type-B (USB-B)
connector. Communication options such as USB-UART and RS-232 are also included, alongside the well-established mikroBUS™ standard, three display options (7-segment, graphical, and character-based LCD), and several different DIP sockets which cover a wide range of 16-bit AVR MCUs. EasyAVR v7 is an integral part of the Mikroe ecosystem for rapid development. Natively supported by Mikroe software tools, it covers many aspects of prototyping and development thanks to a considerable number of different Click boards™ (over a thousand boards), the number of which is growing every day.
Microcontroller Overview
MCU Card / MCU
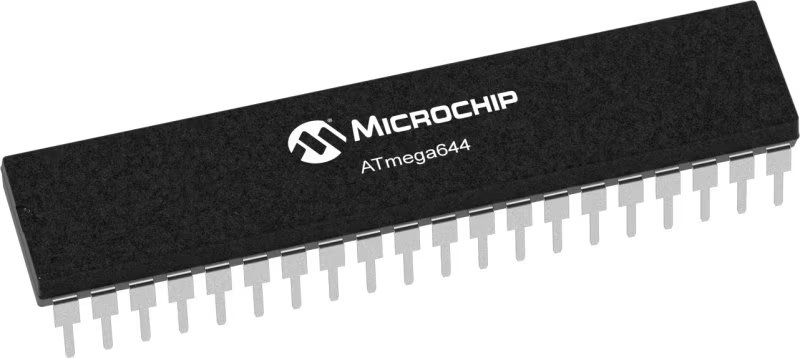
Architecture
AVR
MCU Memory (KB)
64
Silicon Vendor
Microchip
Pin count
40
RAM (Bytes)
4096
Used MCU Pins
mikroBUS™ mapper
Take a closer look
Click board™ Schematic
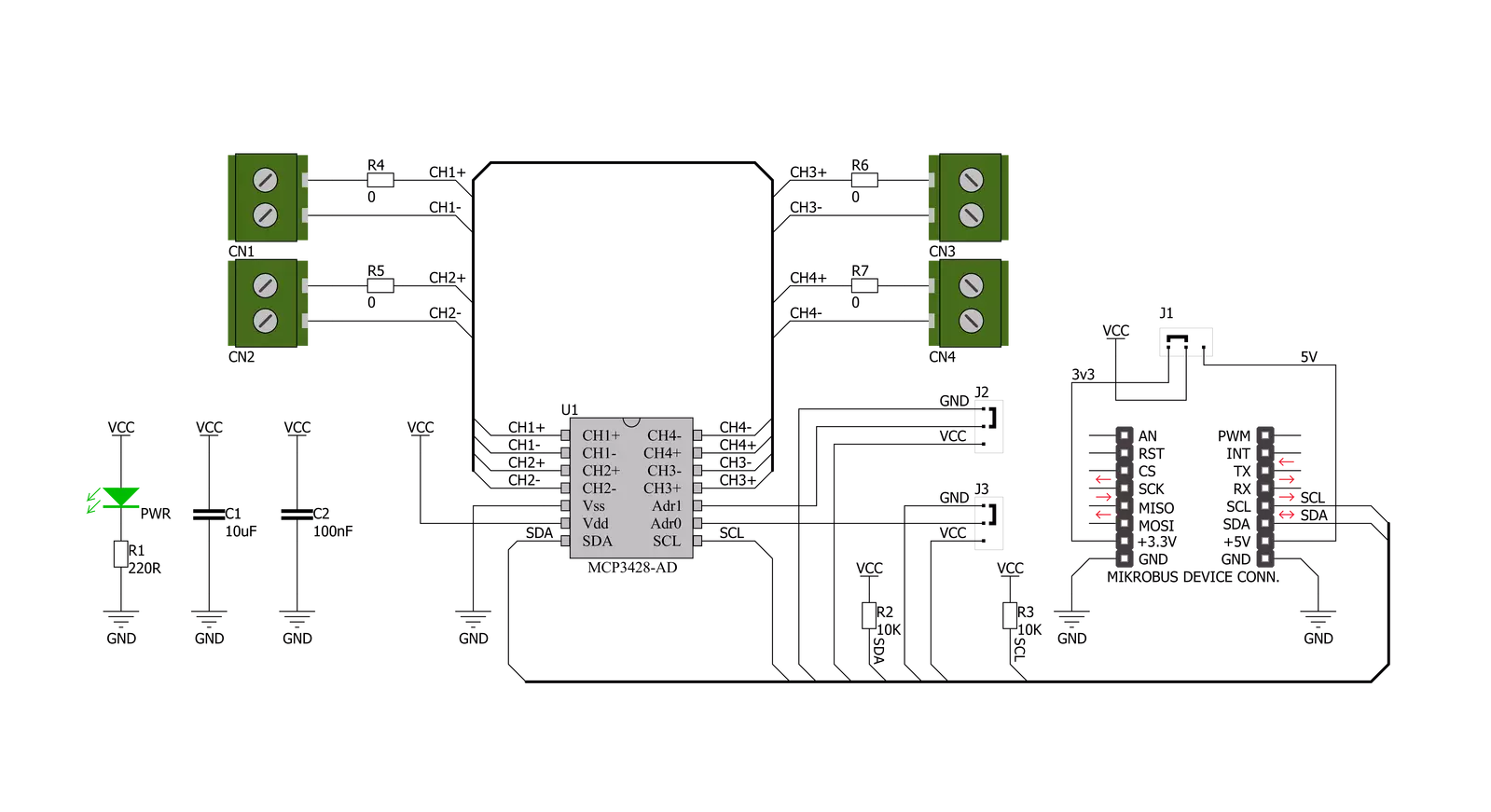
Step by step
Project assembly
Track your results in real time
Application Output
1. Application Output - In Debug mode, the 'Application Output' window enables real-time data monitoring, offering direct insight into execution results. Ensure proper data display by configuring the environment correctly using the provided tutorial.
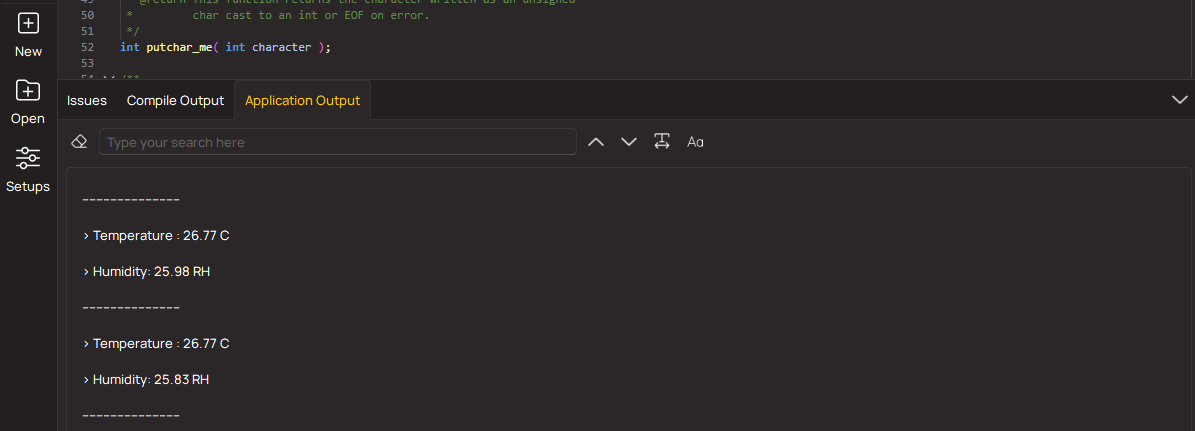
2. UART Terminal - Use the UART Terminal to monitor data transmission via a USB to UART converter, allowing direct communication between the Click board™ and your development system. Configure the baud rate and other serial settings according to your project's requirements to ensure proper functionality. For step-by-step setup instructions, refer to the provided tutorial.
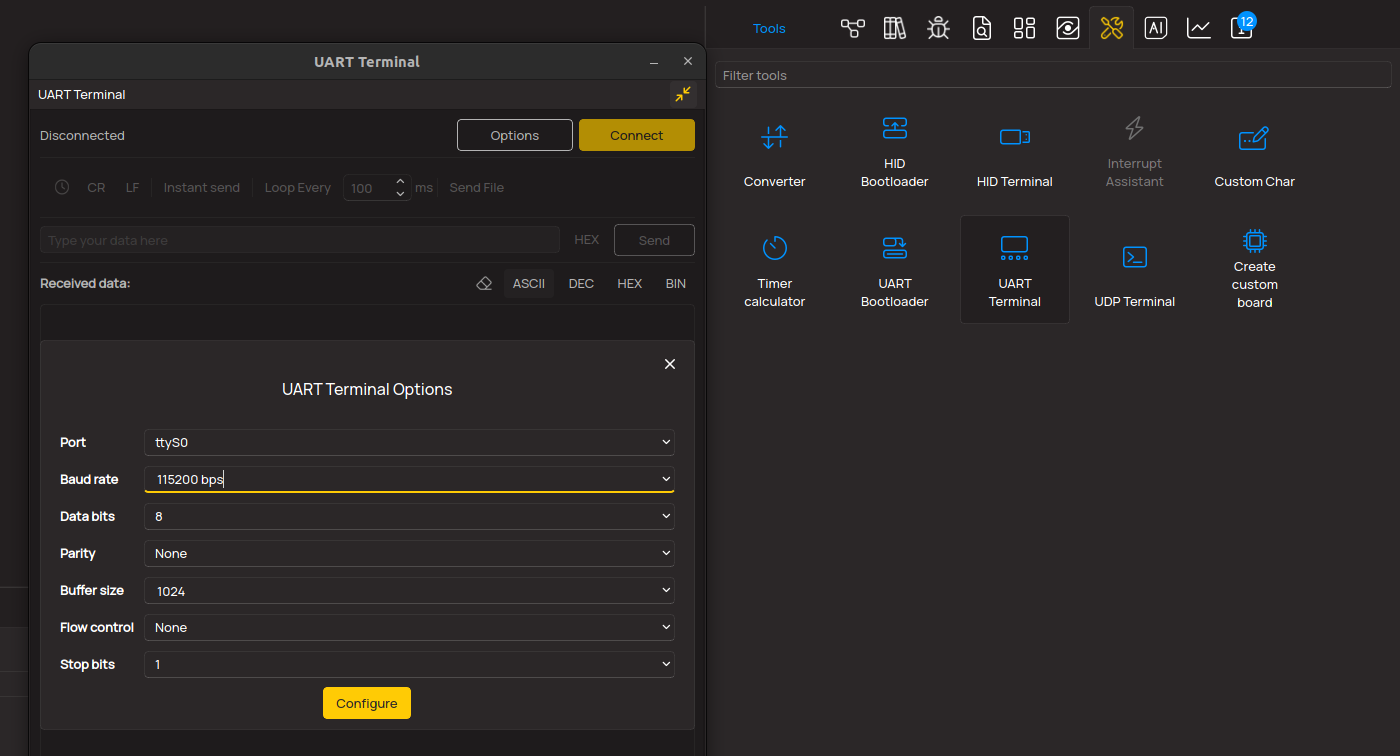
3. Plot Output - The Plot feature offers a powerful way to visualize real-time sensor data, enabling trend analysis, debugging, and comparison of multiple data points. To set it up correctly, follow the provided tutorial, which includes a step-by-step example of using the Plot feature to display Click board™ readings. To use the Plot feature in your code, use the function: plot(*insert_graph_name*, variable_name);. This is a general format, and it is up to the user to replace 'insert_graph_name' with the actual graph name and 'variable_name' with the parameter to be displayed.
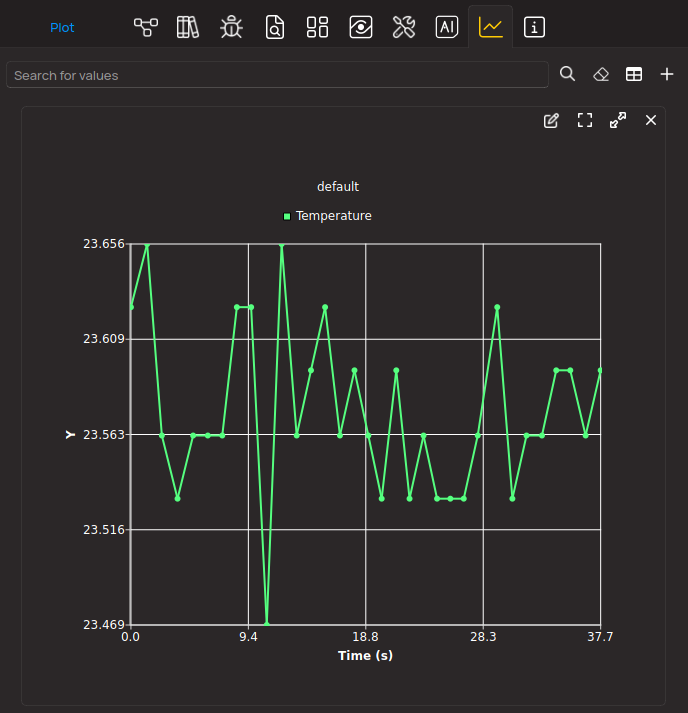
Software Support
Library Description
This library contains API for ADC 3 Click driver.
Key functions:
adc3_default_cfg
- This function is used to initiate general call reset.adc3_read_voltage
- This function is used to read ADC value and calculate voltage.
Open Source
Code example
The complete application code and a ready-to-use project are available through the NECTO Studio Package Manager for direct installation in the NECTO Studio. The application code can also be found on the MIKROE GitHub account.
/*!
* \file
* \brief Adc3 Click example
*
* # Description
* ADC 3 Click represent 16-bit multichannel analog-to-digital converter.
* The Click has four pairs of screw terminals onboard, letting you access the chip’s four differential input channels.
*
* The demo application is composed of two sections :
*
* ## Application Init
* Application Init performs Logger and Click initialization.
*
* ## Application Task
* This is an example that shows the capabilities of the ADC 3 Click
* by taking voltage measurements from all four channel. Results are being sent to the UART Terminal
* where you can track their changes. All data logs write on USB UART and changes for every 1 sec.
*
* \author Mihajlo Djordjevic
*
*/
// ------------------------------------------------------------------- INCLUDES
#include "board.h"
#include "log.h"
#include "adc3.h"
// ------------------------------------------------------------------ VARIABLES
static adc3_t adc3;
static log_t logger;
static float read_volt;
// ------------------------------------------------------ APPLICATION FUNCTIONS
void application_init ( void )
{
log_cfg_t log_cfg;
adc3_cfg_t cfg;
/**
* Logger initialization.
* Default baud rate: 115200
* Default log level: LOG_LEVEL_DEBUG
* @note If USB_UART_RX and USB_UART_TX
* are defined as HAL_PIN_NC, you will
* need to define them manually for log to work.
* See @b LOG_MAP_USB_UART macro definition for detailed explanation.
*/
LOG_MAP_USB_UART( log_cfg );
log_init( &logger, &log_cfg );
log_info( &logger, "---- Application Init ----" );
Delay_ms ( 100 );
// Click initialization.
adc3_cfg_setup( &cfg );
ADC3_MAP_MIKROBUS( cfg, MIKROBUS_1 );
adc3_init( &adc3, &cfg );
log_printf( &logger, "--------------------------\r\n" );
log_printf( &logger, " ------ ADC 3 Click ----- \r\n" );
log_printf( &logger, "--------------------------\r\n" );
Delay_ms ( 1000 );
adc3_default_cfg( &adc3 );
Delay_ms ( 100 );
log_printf( &logger, " -- Initialization done --\r\n" );
log_printf( &logger, "--------------------------\r\n" );
Delay_ms ( 1000 );
}
void application_task ( void )
{
read_volt = adc3_read_voltage( &adc3, ADC3_SELECT_CHANNEL_1, ADC3_SAMPLE_RATE_12, ADC3_PGA_GAIN_X1 );
Delay_ms ( 100 );
log_printf( &logger, "Channel 1 : %0.2f V \r\n", read_volt );
read_volt = adc3_read_voltage( &adc3, ADC3_SELECT_CHANNEL_2, ADC3_SAMPLE_RATE_12, ADC3_PGA_GAIN_X1 );
Delay_ms ( 100 );
log_printf( &logger, "Channel 2 : %0.2f V \r\n", read_volt );
read_volt = adc3_read_voltage( &adc3, ADC3_SELECT_CHANNEL_3, ADC3_SAMPLE_RATE_12, ADC3_PGA_GAIN_X1 );
Delay_ms ( 100 );
log_printf( &logger, "Channel 3 : %0.2f V \r\n", read_volt );
read_volt = adc3_read_voltage( &adc3, ADC3_SELECT_CHANNEL_4, ADC3_SAMPLE_RATE_12, ADC3_PGA_GAIN_X1 );
Delay_ms ( 100 );
log_printf( &logger, "Channel 4 : %0.2f V \r\n", read_volt );
log_printf( &logger, "--------------------------\r\n" );
Delay_ms ( 1000 );
}
int main ( void )
{
/* Do not remove this line or clock might not be set correctly. */
#ifdef PREINIT_SUPPORTED
preinit();
#endif
application_init( );
for ( ; ; )
{
application_task( );
}
return 0;
}
// ------------------------------------------------------------------------ END