Allow devices that traditionally communicate over I2C to be connected and interact over a 1-Wire interface
A
A
Hardware Overview
How does it work?
1-Wire I2C Click is based on the DS28E17, a 1-Wire-to-I2C master bridge from Analog Devices. The bridge supports 15Kbps and 77Kbps 1-Wire protocol with packetized I2C data payloads. The factory-programmed unique 64-bit 1-Wire ROM ID provides an unalterable serial number to the end equipment, thus allowing multiple DS8E17 devices to coexist with other devices in a 1-Wire network and be accessed individually without affecting other devices. The 1-Wire I2C Click allows
communication with complex I2C devices, such as displays, ADCs, DACs, sensors, and more. The bridge provides 1-Wire communication with only one I2C device. 1-Wire I2C Click uses the 1-Wire interface as a bridge to the standard 2-Wire I2C interface to communicate with the host MCU. You can choose a One-Wire input pin over the OW SEL jumper, where the OW1 is routed to an analog pin of the mikroBUS™ socket and is set by default. You can also reset the bridge over the RST pin. The I2C
device can be connected over a 4-pin screw terminal. This Click board™ can be operated only with a 3.3V logic voltage level. The board must perform appropriate logic voltage level conversion before using MCUs with different logic levels. Also, this Click board™ comes equipped with a library containing functions and an example code that can be used as a reference for further development.
Features overview
Development board
Flip&Click PIC32MZ is a compact development board designed as a complete solution that brings the flexibility of add-on Click boards™ to your favorite microcontroller, making it a perfect starter kit for implementing your ideas. It comes with an onboard 32-bit PIC32MZ microcontroller, the PIC32MZ2048EFH100 from Microchip, four mikroBUS™ sockets for Click board™ connectivity, two USB connectors, LED indicators, buttons, debugger/programmer connectors, and two headers compatible with Arduino-UNO pinout. Thanks to innovative manufacturing technology,
it allows you to build gadgets with unique functionalities and features quickly. Each part of the Flip&Click PIC32MZ development kit contains the components necessary for the most efficient operation of the same board. In addition, there is the possibility of choosing the Flip&Click PIC32MZ programming method, using the chipKIT bootloader (Arduino-style development environment) or our USB HID bootloader using mikroC, mikroBasic, and mikroPascal for PIC32. This kit includes a clean and regulated power supply block through the USB Type-C (USB-C) connector. All communication
methods that mikroBUS™ itself supports are on this board, including the well-established mikroBUS™ socket, user-configurable buttons, and LED indicators. Flip&Click PIC32MZ development kit allows you to create a new application in minutes. Natively supported by Mikroe software tools, it covers many aspects of prototyping thanks to a considerable number of different Click boards™ (over a thousand boards), the number of which is growing every day.
Microcontroller Overview
MCU Card / MCU
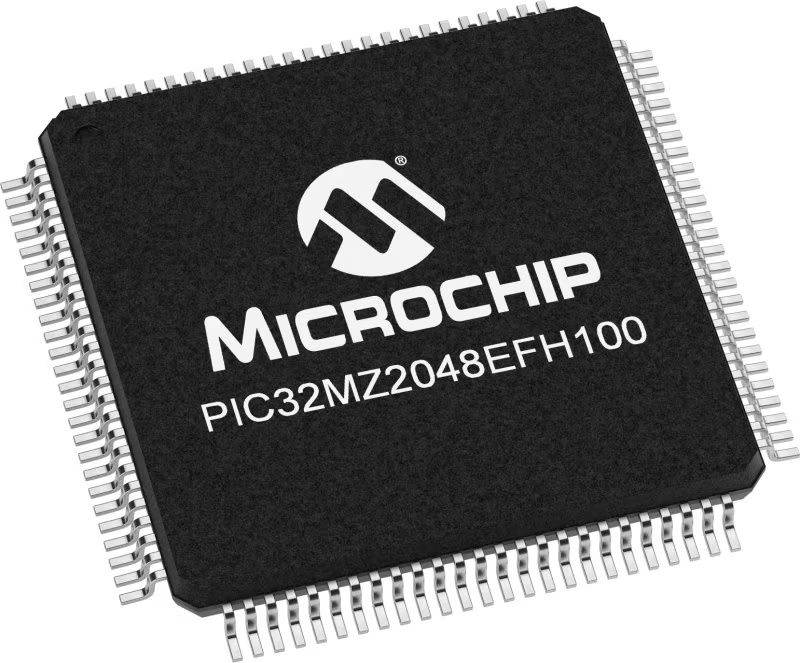
Architecture
PIC32
MCU Memory (KB)
2048
Silicon Vendor
Microchip
Pin count
100
RAM (Bytes)
524288
Used MCU Pins
mikroBUS™ mapper
Take a closer look
Click board™ Schematic
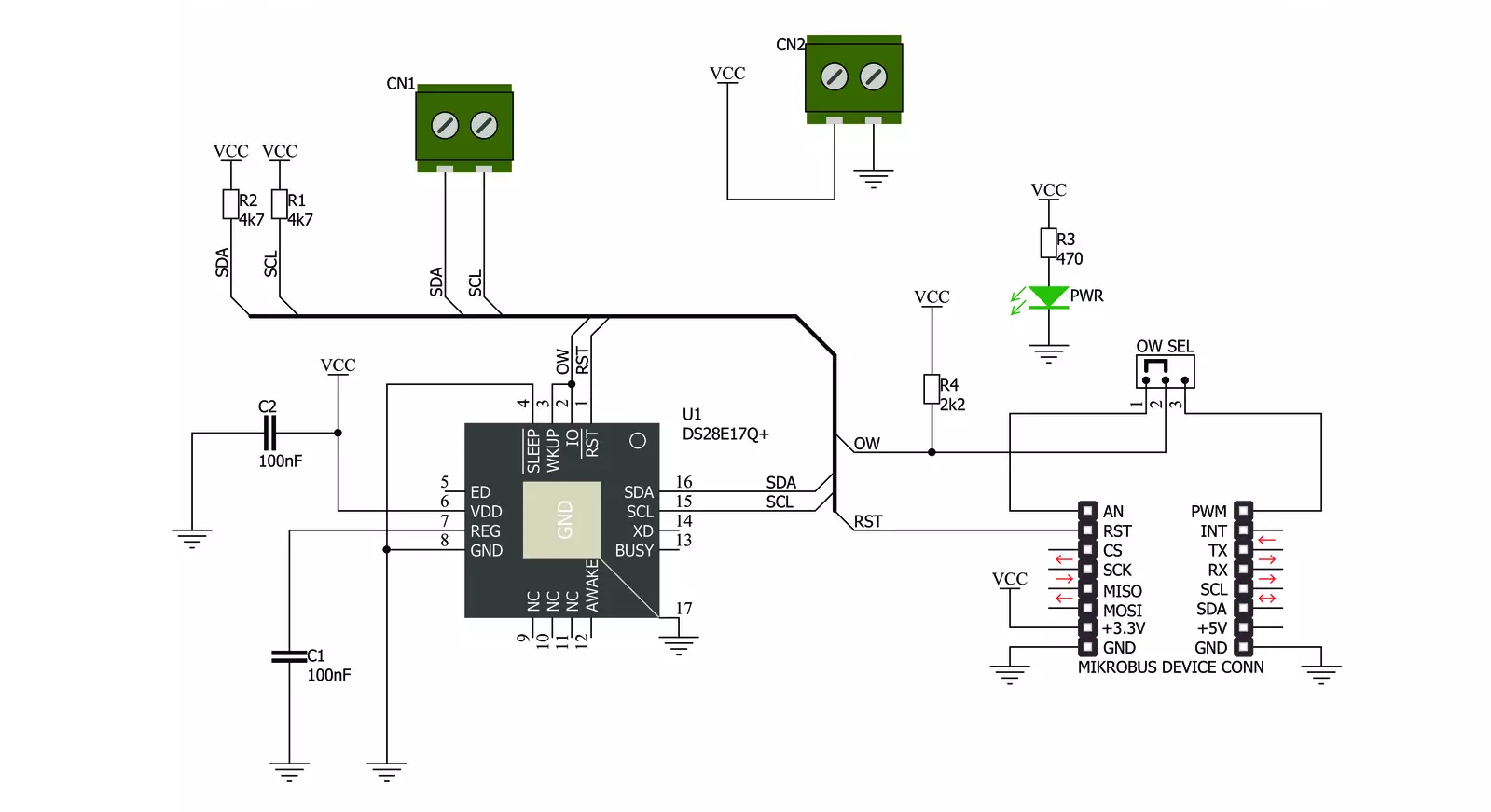
Step by step
Project assembly
Track your results in real time
Application Output via Debug Mode
1. Once the code example is loaded, pressing the "DEBUG" button initiates the build process, programs it on the created setup, and enters Debug mode.
2. After the programming is completed, a header with buttons for various actions within the IDE becomes visible. Clicking the green "PLAY" button starts reading the results achieved with the Click board™. The achieved results are displayed in the Application Output tab.
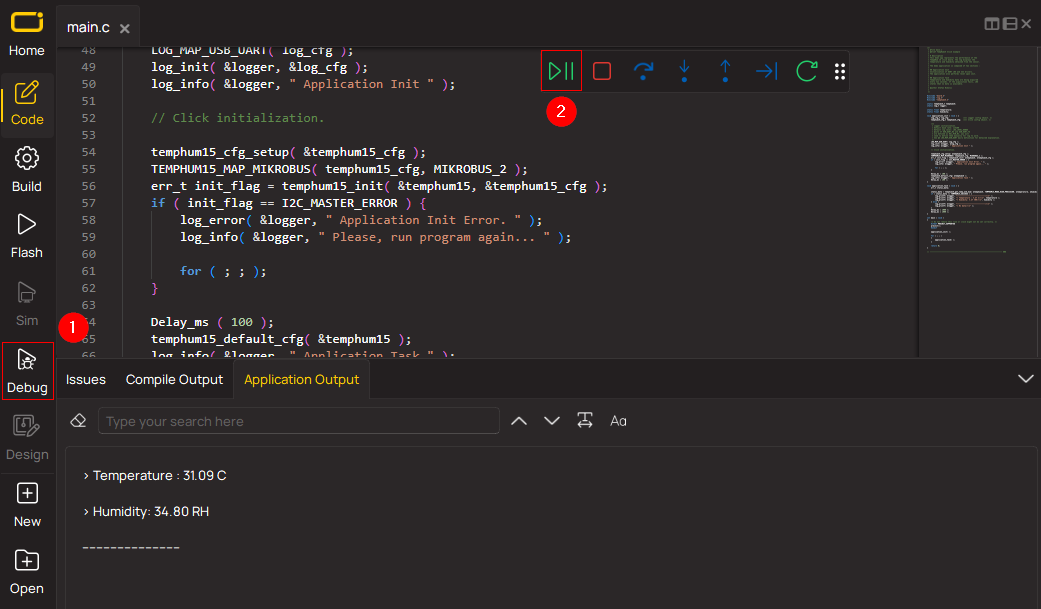
Software Support
Library Description
This library contains API for 1-Wire I2C Click driver.
Key functions:
c1wirei2c_reset_device
- This function resets the device by toggling the RST pin statec1wirei2c_write_data
- This function addresses and writes 1-255 bytes to an I2C slave without completing the transaction with a stopc1wirei2c_read_data_stop
- This function is used to address and read 1-255 bytes from an I2C slave in one transaction
Open Source
Code example
This example can be found in NECTO Studio. Feel free to download the code, or you can copy the code below.
/*!
* @file main.c
* @brief 1-Wire I2C Click Example.
*
* # Description
* This example demonstrates the use of 1-Wire I2C click board by reading
* the temperature measurement from connected Thermo 4 click board.
*
* The demo application is composed of two sections :
*
* ## Application Init
* Initializes the driver and performs the click default configuration.
*
* ## Application Task
* Reads the temperature measurement from connected Thermo 4 click board and
* displays the results on the USB UART once per second.
*
* @author Stefan Filipovic
*
*/
#include "board.h"
#include "log.h"
#include "c1wirei2c.h"
// Thermo 4 device settings
#define DEVICE_NAME "Thermo 4 click"
#define DEVICE_SLAVE_ADDRESS 0x48
#define DEVICE_REG_TEMPERATURE 0x00
#define DEVICE_TEMPERATURE_RES 0.125f
static c1wirei2c_t c1wirei2c;
static log_t logger;
void application_init ( void )
{
log_cfg_t log_cfg; /**< Logger config object. */
c1wirei2c_cfg_t c1wirei2c_cfg; /**< Click config object. */
/**
* Logger initialization.
* Default baud rate: 115200
* Default log level: LOG_LEVEL_DEBUG
* @note If USB_UART_RX and USB_UART_TX
* are defined as HAL_PIN_NC, you will
* need to define them manually for log to work.
* See @b LOG_MAP_USB_UART macro definition for detailed explanation.
*/
LOG_MAP_USB_UART( log_cfg );
log_init( &logger, &log_cfg );
log_info( &logger, " Application Init " );
// Click initialization.
c1wirei2c_cfg_setup( &c1wirei2c_cfg );
C1WIREI2C_MAP_MIKROBUS( c1wirei2c_cfg, MIKROBUS_1 );
if ( ONE_WIRE_ERROR == c1wirei2c_init( &c1wirei2c, &c1wirei2c_cfg ) )
{
log_error( &logger, " Communication init." );
for ( ; ; );
}
if ( C1WIREI2C_ERROR == c1wirei2c_default_cfg ( &c1wirei2c ) )
{
log_error( &logger, " Default configuration." );
for ( ; ; );
}
log_info( &logger, " Application Task " );
}
void application_task ( void )
{
float temperature = 0;
uint8_t reg_data[ 2 ] = { 0 };
uint8_t reg_addr = DEVICE_REG_TEMPERATURE;
if ( ( C1WIREI2C_OK == c1wirei2c_write_data ( &c1wirei2c, DEVICE_SLAVE_ADDRESS, ®_addr, 1 ) ) &&
( C1WIREI2C_OK == c1wirei2c_read_data_stop ( &c1wirei2c, DEVICE_SLAVE_ADDRESS, reg_data, 2 ) ) )
{
temperature = ( ( ( int16_t ) ( ( ( uint16_t ) reg_data[ 0 ] << 8 ) |
reg_data[ 1 ] ) ) >> 5 ) * DEVICE_TEMPERATURE_RES;
log_printf( &logger, "\r\n%s - Temperature: %.3f degC\r\n", ( char * ) DEVICE_NAME, temperature );
}
else
{
log_error( &logger, "%s - no communication!\r\n", ( char * ) DEVICE_NAME );
}
Delay_ms( 1000 );
}
int main ( void )
{
application_init( );
for ( ; ; )
{
application_task( );
}
return 0;
}
// ------------------------------------------------------------------------ END