We empower your lifestyle with smart temperature monitoring. Our solution is designed to seamlessly integrate into your daily routine, giving you the insights needed to adapt, thrive, and enjoy your environment to the fullest.
A
A
Hardware Overview
How does it work?
Thermo 30 Click is based on the STS32-DIS-10KS, a third-generation, high-reliability, certified digital temperature sensor from Sensirion. Every sensor has its unique serial number and is supplied with an ISO17025-accredited calibration certificate, which comprises three temperatures: −30°C, 5°C, and 70°C. The calibration is performed on each sensor and granted by the Swiss Accreditation Service. The calibration data and the serial number are stored in the onboard memory. Temperature data can be acquired both in Single Shot and Periodic Data mode as a 16-bit temperature value. The sensor is also equipped with an internal heater that can be used for plausibility checking
only and can be switched on and off through software. The Thermo 30 Click uses a standard 2-Wire I2C interface to communicate with the host MCU with fast-mode support and frequencies up to 1MHz. The I2C address can be selected via the ADDR SEL jumper (0 set by default). The STS32-DIS-10KS can be reset via the RST pin of the mikroBUS™ socket, through a general call, or software as a soft reset. Resetting over the RST pin or through a general call (according to I2C specifications) achieves a full reset, while with the soft reset, the sensor resets the system controller and reloads calibration data from memory. In addition, the Thermo 30 Click comes with an
alert function, a feature that can interrupt the host MCU over the ALR pin of the mikroBUS™ socket if there is an alarm condition. The red ALARM LED stands as a visual presentation of this feature. This Click board™ can operate with either 3.3V or 5V logic voltage levels selected via the VCC SEL jumper. This way, both 3.3V and 5V capable MCUs can use the communication lines properly. Also, this Click board™ comes equipped with a library containing easy-to-use functions and an example code that can be used as a reference for further development.
Features overview
Development board
Nucleo 32 with STM32F031K6 MCU board provides an affordable and flexible platform for experimenting with STM32 microcontrollers in 32-pin packages. Featuring Arduino™ Nano connectivity, it allows easy expansion with specialized shields, while being mbed-enabled for seamless integration with online resources. The
board includes an on-board ST-LINK/V2-1 debugger/programmer, supporting USB reenumeration with three interfaces: Virtual Com port, mass storage, and debug port. It offers a flexible power supply through either USB VBUS or an external source. Additionally, it includes three LEDs (LD1 for USB communication, LD2 for power,
and LD3 as a user LED) and a reset push button. The STM32 Nucleo-32 board is supported by various Integrated Development Environments (IDEs) such as IAR™, Keil®, and GCC-based IDEs like AC6 SW4STM32, making it a versatile tool for developers.
Microcontroller Overview
MCU Card / MCU
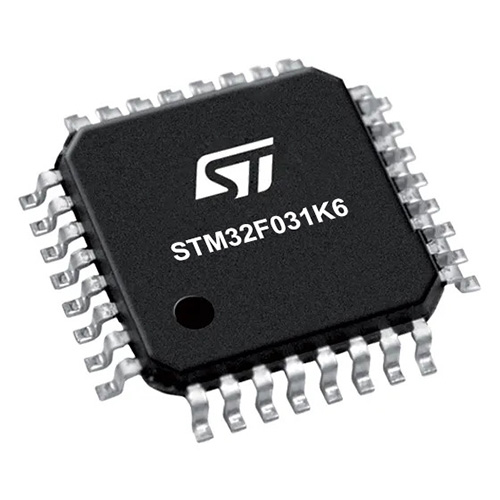
Architecture
ARM Cortex-M0
MCU Memory (KB)
32
Silicon Vendor
STMicroelectronics
Pin count
32
RAM (Bytes)
4096
You complete me!
Accessories
Click Shield for Nucleo-32 is the perfect way to expand your development board's functionalities with STM32 Nucleo-32 pinout. The Click Shield for Nucleo-32 provides two mikroBUS™ sockets to add any functionality from our ever-growing range of Click boards™. We are fully stocked with everything, from sensors and WiFi transceivers to motor control and audio amplifiers. The Click Shield for Nucleo-32 is compatible with the STM32 Nucleo-32 board, providing an affordable and flexible way for users to try out new ideas and quickly create prototypes with any STM32 microcontrollers, choosing from the various combinations of performance, power consumption, and features. The STM32 Nucleo-32 boards do not require any separate probe as they integrate the ST-LINK/V2-1 debugger/programmer and come with the STM32 comprehensive software HAL library and various packaged software examples. This development platform provides users with an effortless and common way to combine the STM32 Nucleo-32 footprint compatible board with their favorite Click boards™ in their upcoming projects.
Used MCU Pins
mikroBUS™ mapper
Take a closer look
Click board™ Schematic
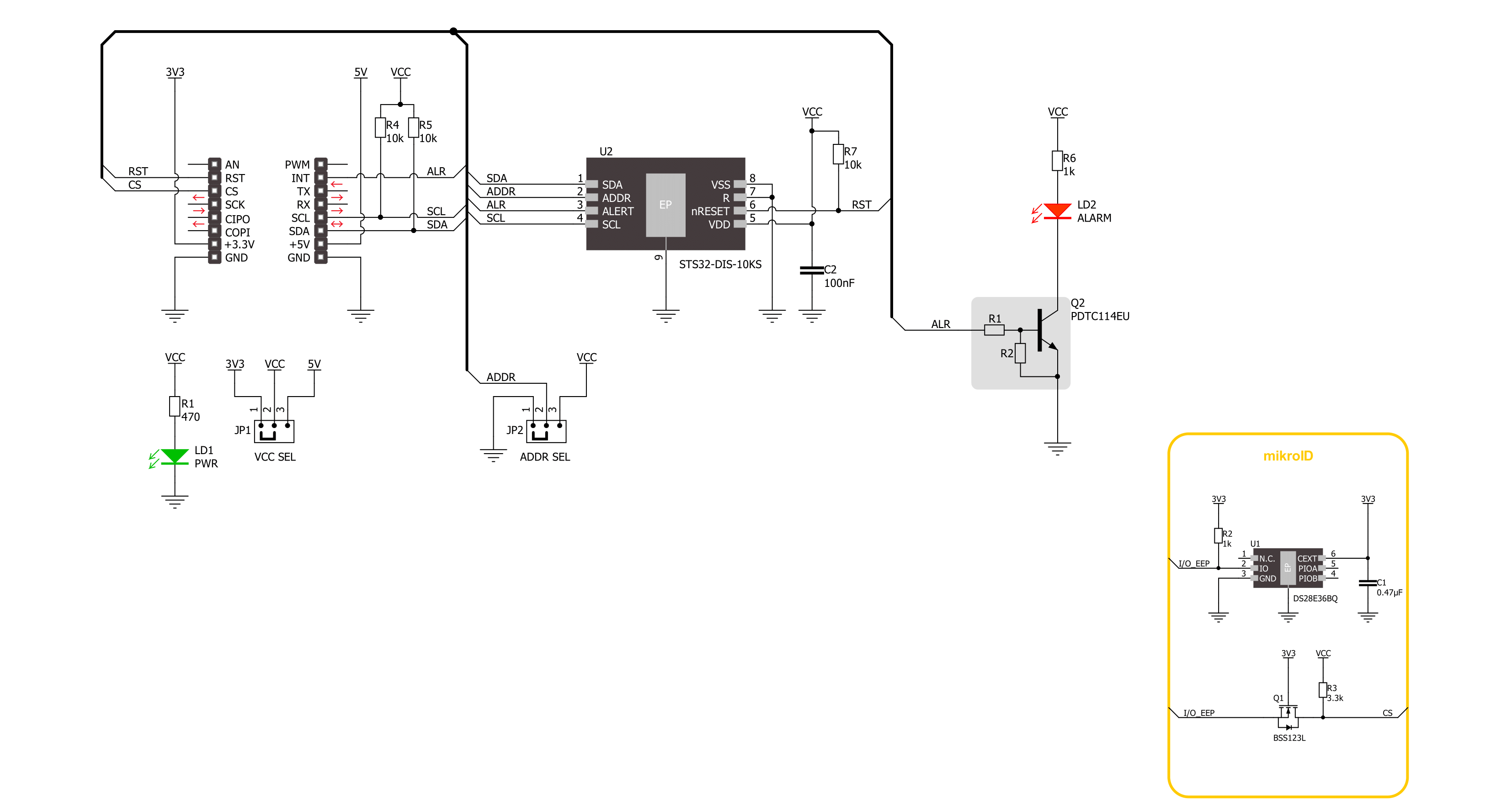
Step by step
Project assembly
Track your results in real time
Application Output
1. Application Output - In Debug mode, the 'Application Output' window enables real-time data monitoring, offering direct insight into execution results. Ensure proper data display by configuring the environment correctly using the provided tutorial.
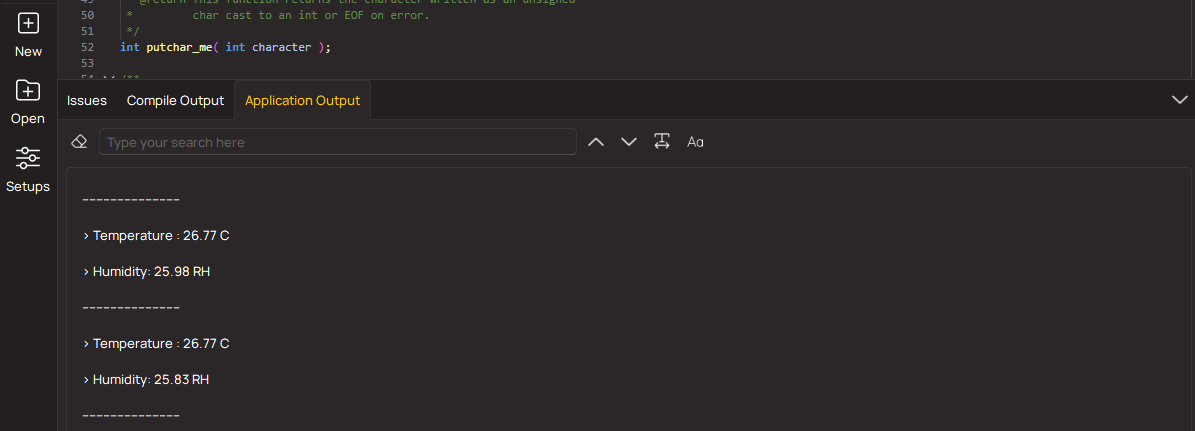
2. UART Terminal - Use the UART Terminal to monitor data transmission via a USB to UART converter, allowing direct communication between the Click board™ and your development system. Configure the baud rate and other serial settings according to your project's requirements to ensure proper functionality. For step-by-step setup instructions, refer to the provided tutorial.
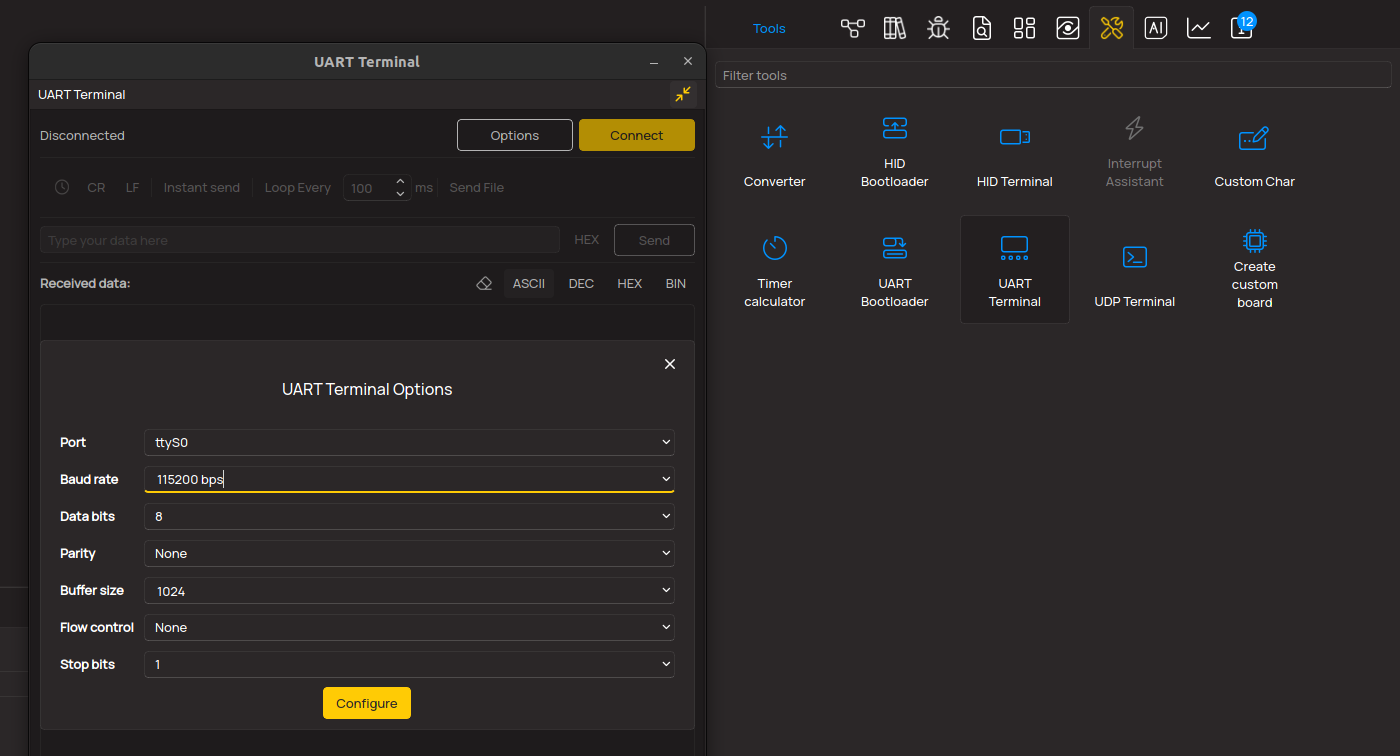
3. Plot Output - The Plot feature offers a powerful way to visualize real-time sensor data, enabling trend analysis, debugging, and comparison of multiple data points. To set it up correctly, follow the provided tutorial, which includes a step-by-step example of using the Plot feature to display Click board™ readings. To use the Plot feature in your code, use the function: plot(*insert_graph_name*, variable_name);. This is a general format, and it is up to the user to replace 'insert_graph_name' with the actual graph name and 'variable_name' with the parameter to be displayed.
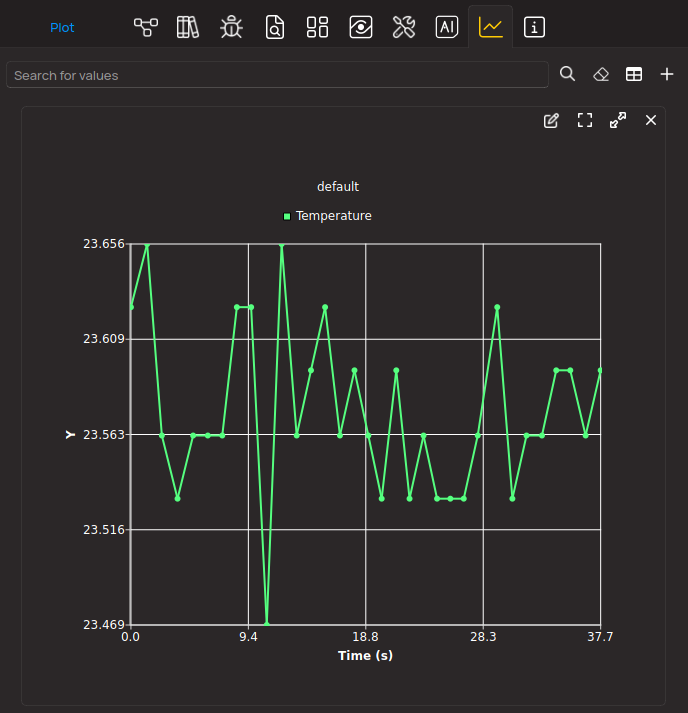
Software Support
Library Description
This library contains API for Thermo 30 Click driver.
Key functions:
thermo30_hw_reset
- Thermo 30 hw reset device function.thermo30_start_measurement
- Thermo 30 start measurement function.thermo30_read_temperature
- Thermo 30 read temperature function.
Open Source
Code example
The complete application code and a ready-to-use project are available through the NECTO Studio Package Manager for direct installation in the NECTO Studio. The application code can also be found on the MIKROE GitHub account.
/*!
* @file main.c
* @brief Thermo 30 Click example
*
* # Description
* This example demonstrates the use of Thermo 30 click board by reading and displaying
* the temperature measurements.
*
* The demo application is composed of two sections :
*
* ## Application Init
* Initializes the driver and resets the device, and
* starts the periodic measurements at 1 mps with high repeatability.
*
* ## Application Task
* Reads the temperature measurement in degrees Celsius and displays the results on the USB UART
* approximately once per second.
*
* @author Stefan Ilic
*
*/
#include "board.h"
#include "log.h"
#include "thermo30.h"
static thermo30_t thermo30;
static log_t logger;
void application_init ( void )
{
log_cfg_t log_cfg; /**< Logger config object. */
thermo30_cfg_t thermo30_cfg; /**< Click config object. */
/**
* Logger initialization.
* Default baud rate: 115200
* Default log level: LOG_LEVEL_DEBUG
* @note If USB_UART_RX and USB_UART_TX
* are defined as HAL_PIN_NC, you will
* need to define them manually for log to work.
* See @b LOG_MAP_USB_UART macro definition for detailed explanation.
*/
LOG_MAP_USB_UART( log_cfg );
log_init( &logger, &log_cfg );
log_info( &logger, " Application Init " );
// Click initialization.
thermo30_cfg_setup( &thermo30_cfg );
THERMO30_MAP_MIKROBUS( thermo30_cfg, MIKROBUS_1 );
if ( I2C_MASTER_ERROR == thermo30_init( &thermo30, &thermo30_cfg ) )
{
log_error( &logger, " Communication init." );
for ( ; ; );
}
if ( THERMO30_ERROR == thermo30_default_cfg( &thermo30 ) )
{
log_error( &logger, " Default configuration." );
for ( ; ; );
}
log_info( &logger, " Application Task " );
}
void application_task ( void )
{
float temperature;
if ( THERMO30_OK == thermo30_read_temperature( &thermo30, &temperature ) )
{
log_printf( &logger, " Temperature: %.2f deg C\r\n\n", temperature );
}
Delay_ms ( 1000 );
}
void main ( void )
{
application_init( );
for ( ; ; )
{
application_task( );
}
}
// ------------------------------------------------------------------------ END