Completely isolated I2C interface
A
A
Hardware Overview
How does it work?
I2C Isolator 4 Click is based on the MAX14937, a two-channel, 5kVRMS I2C digital isolator from Analog Devices. The MAX14937 bidirectionally buffers the two I2C signals across the isolation barrier and supports I2C clock-stretching while providing 5kVrms of galvanic isolation. It transfers digital signals between circuits with different power domains at ambient temperatures and offers glitch-free operation, excellent reliability,
and very long operational life. The wide temperature range and high isolation voltage make the device ideal for harsh industrial environments. This Click board™ also possesses two terminals labeled as VIN and SDA/SCL at the bottom of the Click board™, where VIN represents the B-side power supply of the isolator, while the other corresponds to the isolated bidirectional logic-bus terminal.
This Click board™ can operate with either 3.3V or 5V logic voltage levels selected via the VCC SEL jumper. This way, both 3.3V and 5V capable MCUs can use the communication lines properly. However, the Click board™ comes equipped with a library containing easy-to-use functions and an example code that can be used, as a reference, for further development.
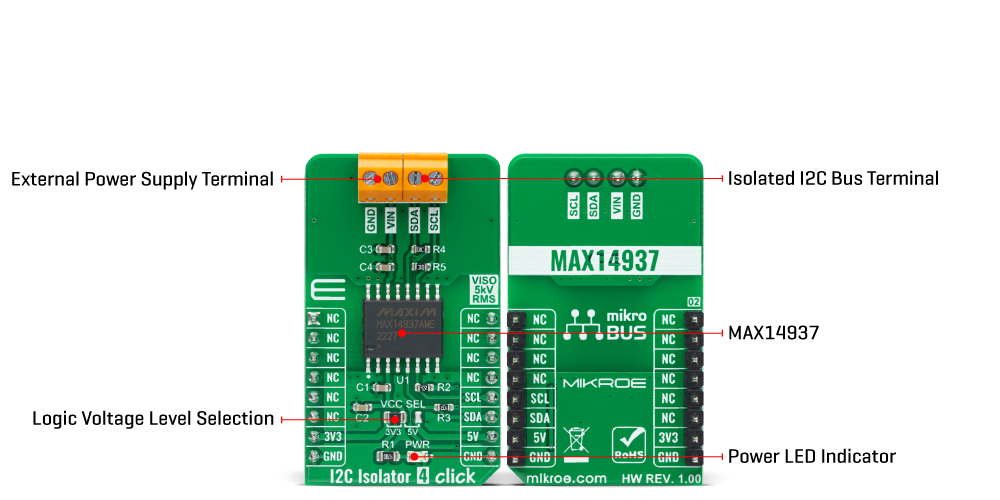
Features overview
Development board
Fusion for TIVA v8 is a development board specially designed for the needs of rapid development of embedded applications. It supports a wide range of microcontrollers, such as different 32-bit ARM® Cortex®-M based MCUs from Texas Instruments, regardless of their number of pins, and a broad set of unique functions, such as the first-ever embedded debugger/programmer over a WiFi network. The development board is well organized and designed so that the end-user has all the necessary elements, such as switches, buttons, indicators, connectors, and others, in one place. Thanks to innovative manufacturing technology, Fusion for TIVA v8 provides a fluid and immersive working experience, allowing access
anywhere and under any circumstances at any time. Each part of the Fusion for TIVA v8 development board contains the components necessary for the most efficient operation of the same board. An advanced integrated CODEGRIP programmer/debugger module offers many valuable programming/debugging options, including support for JTAG, SWD, and SWO Trace (Single Wire Output)), and seamless integration with the Mikroe software environment. Besides, it also includes a clean and regulated power supply module for the development board. It can use a wide range of external power sources, including a battery, an external 12V power supply, and a power source via the USB Type-C (USB-C) connector.
Communication options such as USB-UART, USB HOST/DEVICE, CAN (on the MCU card, if supported), and Ethernet is also included. In addition, it also has the well-established mikroBUS™ standard, a standardized socket for the MCU card (SiBRAIN standard), and two display options for the TFT board line of products and character-based LCD. Fusion for TIVA v8 is an integral part of the Mikroe ecosystem for rapid development. Natively supported by Mikroe software tools, it covers many aspects of prototyping and development thanks to a considerable number of different Click boards™ (over a thousand boards), the number of which is growing every day.
Microcontroller Overview
MCU Card / MCU
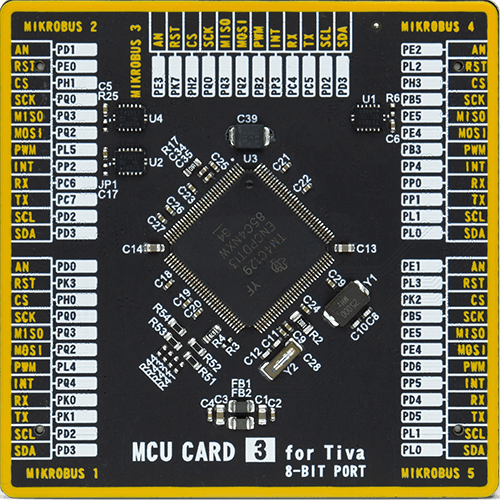
Type
8th Generation
Architecture
ARM Cortex-M4
MCU Memory (KB)
1024
Silicon Vendor
Texas Instruments
Pin count
128
RAM (Bytes)
262144
Used MCU Pins
mikroBUS™ mapper
Take a closer look
Schematic
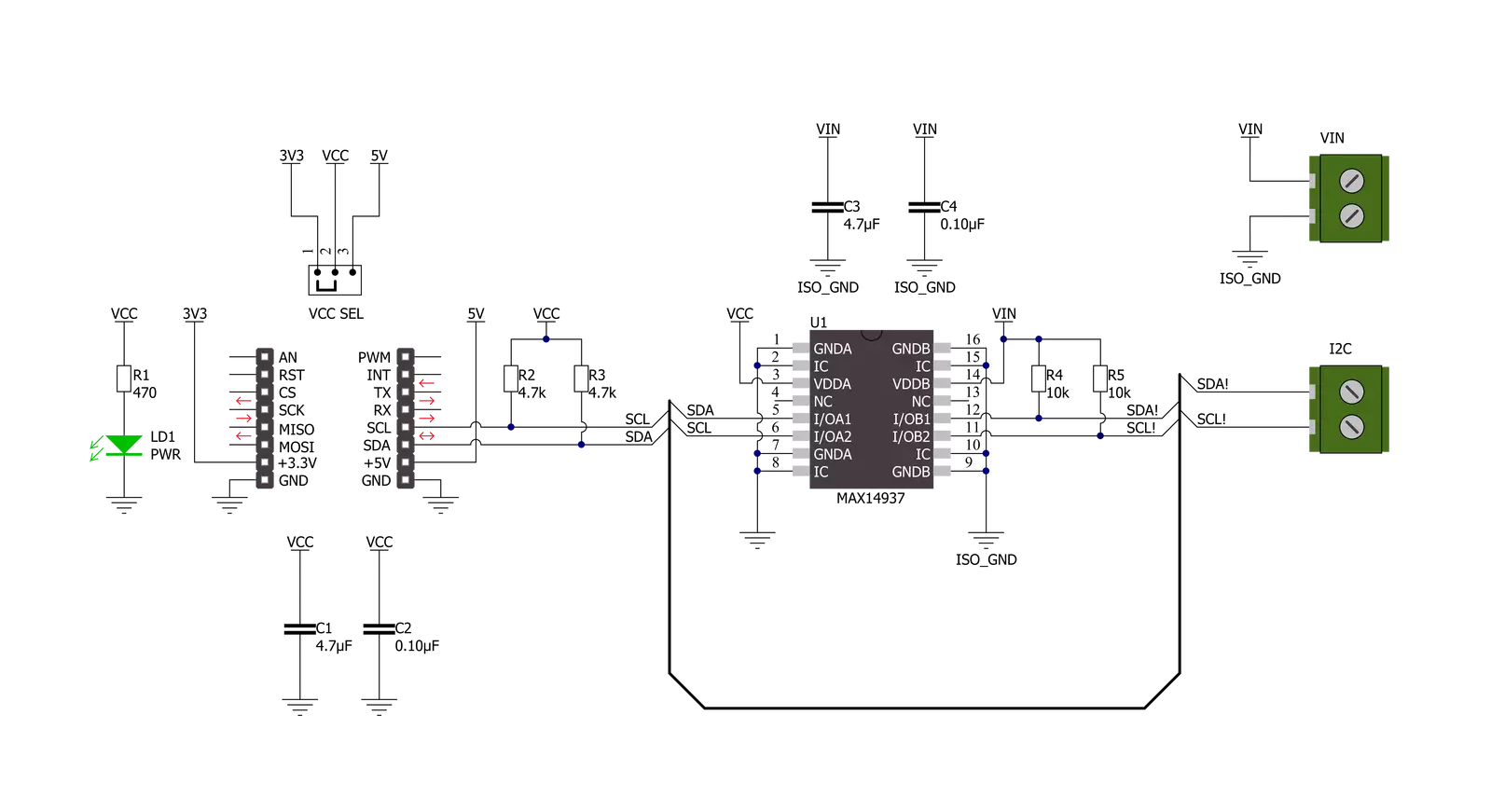
Step by step
Project assembly
Track your results in real time
Application Output via UART Mode
1. Once the code example is loaded, pressing the "FLASH" button initiates the build process, and programs it on the created setup.
2. After the programming is completed, click on the Tools icon in the upper-right panel, and select the UART Terminal.
3. After opening the UART Terminal tab, first check the baud rate setting in the Options menu (default is 115200). If this parameter is correct, activate the terminal by clicking the "CONNECT" button.
4. Now terminal status changes from Disconnected to Connected in green, and the data is displayed in the Received data field.
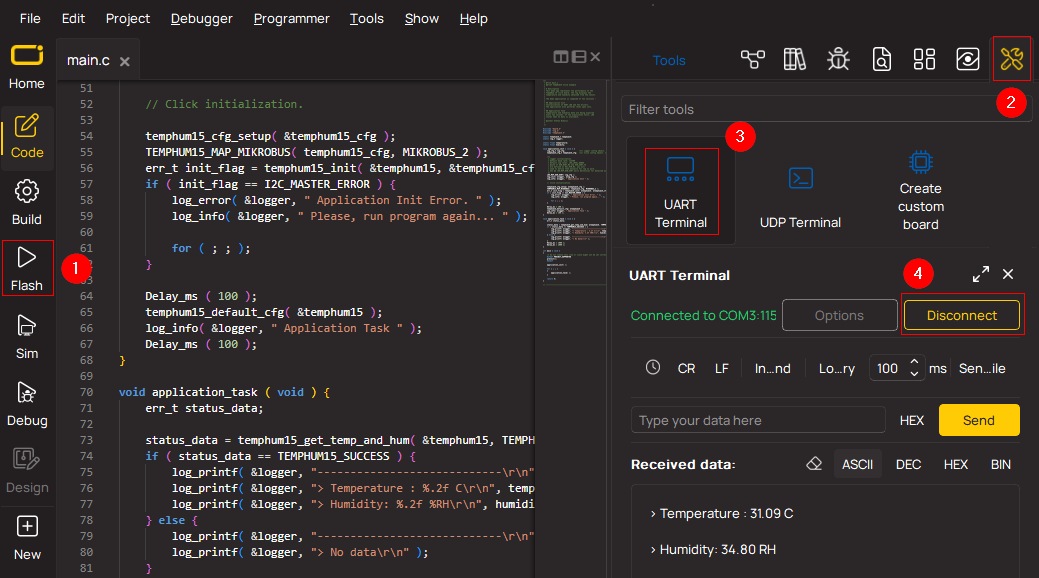
Software Support
Library Description
This library contains API for I2C Isolator 4 Click driver.
Key functions:
i2cisolator4_generic_write
I2C Isolator 4 I2C writing function.i2cisolator4_generic_read
I2C Isolator 4 I2C reading function.i2cisolator4_set_slave_address
I2C Isolator 4 set I2C Slave address function.
Open Source
Code example
This example can be found in NECTO Studio. Feel free to download the code, or you can copy the code below.
/*!
* @file main.c
* @brief I2cIsolator4 Click example
*
* # Description
* This library contains API for the I2C Isolator 4 click driver.
* This demo application shows an example of an I2C Isolator 4 click
* wired to the VAV Press click for reading
* differential pressure and temperature measurement.
*
* The demo application is composed of two sections :
*
* ## Application Init
* Initialization of I2C module and log UART.
* After driver initialization and default settings,
* the app set VAV Press click I2C slave address ( 0x5C )
* and enable device.
*
* ## Application Task
* This is an example that shows the use of an I2C Isolator 4 click board™.
* Logs pressure difference [ Pa ] and temperature [ degree Celsius ] values
* of the VAV Press click wired to the I2C Isolator 4 click board™.
* Results are being sent to the Usart Terminal where you can track their changes.
*
* @note
* void get_dif_press_and_temp ( void ) - Get differential pressure and temperature function.
*
* @author Nenad Filipovic
*
*/
#include "board.h"
#include "log.h"
#include "i2cisolator4.h"
#define I2CISOLATOR4_VAV_PRESS_DEV_ADDR 0x5C
#define I2CISOLATOR4_VAV_PRESS_CMD_START_PRESSURE_CONVERSION 0x21
#define I2CISOLATOR4_VAV_PRESS_PRESS_SCALE_FACTOR 1200
#define I2CISOLATOR4_VAV_PRESS_TEMP_SCALE_FACTOR 72
#define I2CISOLATOR4_VAV_PRESS_READOUT_AT_KNOWN_TEMPERATURE 105
#define I2CISOLATOR4_VAV_PRESS_KNOWN_TEMPERATURE_C 23.1
static i2cisolator4_t i2cisolator4;
static log_t logger;
static float diff_press;
static float temperature;
void get_dif_press_and_temp ( void ) {
uint8_t rx_buf[ 4 ];
int16_t readout_data;
i2cisolator4_generic_read( &i2cisolator4, I2CISOLATOR4_VAV_PRESS_CMD_START_PRESSURE_CONVERSION, &rx_buf[ 0 ], 4 );
readout_data = rx_buf[ 1 ];
readout_data <<= 9;
readout_data |= rx_buf[ 0 ];
readout_data >>= 1;
diff_press = ( float ) readout_data;
diff_press /= I2CISOLATOR4_VAV_PRESS_PRESS_SCALE_FACTOR;
readout_data = rx_buf[ 3 ];
readout_data <<= 8;
readout_data |= rx_buf[ 2 ];
temperature = ( float ) readout_data;
temperature -= I2CISOLATOR4_VAV_PRESS_READOUT_AT_KNOWN_TEMPERATURE;
temperature /= I2CISOLATOR4_VAV_PRESS_TEMP_SCALE_FACTOR;
temperature += I2CISOLATOR4_VAV_PRESS_KNOWN_TEMPERATURE_C;
}
void application_init ( void ) {
log_cfg_t log_cfg; /**< Logger config object. */
i2cisolator4_cfg_t i2cisolator4_cfg; /**< Click config object. */
/**
* Logger initialization.
* Default baud rate: 115200
* Default log level: LOG_LEVEL_DEBUG
* @note If USB_UART_RX and USB_UART_TX
* are defined as HAL_PIN_NC, you will
* need to define them manually for log to work.
* See @b LOG_MAP_USB_UART macro definition for detailed explanation.
*/
LOG_MAP_USB_UART( log_cfg );
log_init( &logger, &log_cfg );
log_info( &logger, " Application Init " );
// Click initialization.
i2cisolator4_cfg_setup( &i2cisolator4_cfg );
I2CISOLATOR4_MAP_MIKROBUS( i2cisolator4_cfg, MIKROBUS_1 );
err_t init_flag = i2cisolator4_init( &i2cisolator4, &i2cisolator4_cfg );
if ( init_flag == I2C_MASTER_ERROR ) {
log_error( &logger, " Application Init Error. " );
log_info( &logger, " Please, run program again... " );
for ( ; ; );
}
log_info( &logger, " Application Task " );
Delay_ms( 100 );
log_printf( &logger, "--------------------------------\r\n" );
log_printf( &logger, " Set I2C Slave Address \r\n" );
i2cisolator4_set_slave_address ( &i2cisolator4, I2CISOLATOR4_VAV_PRESS_DEV_ADDR );
Delay_ms( 100 );
}
void application_task ( void ) {
get_dif_press_and_temp( );
log_printf( &logger, " Diff. Pressure : %.4f Pa\r\n", diff_press );
log_printf( &logger, " Temperature : %.4f C\r\n", temperature );
log_printf( &logger, "--------------------------------\r\n" );
Delay_ms( 2000 );
}
void main ( void ) {
application_init( );
for ( ; ; ) {
application_task( );
}
}
// ------------------------------------------------------------------------ END