Protect your health and well-being by detecting harmful organic gases with our cutting-edge sensing technology
A
A
Hardware Overview
How does it work?
Pollution Click is based on the WSP2110, a VOC gas sensor from Winsen. The MQ-135 can detect the presence and concentration of toxic gases in the air, such as toluene, benzene, methanal, and alcohol. It consists of a heater and metal oxide semiconductor material on the ceramic substrate of subminiature Al2O3, which are fetched out by electrode down-lead and encapsulated in a metal socket and cap. Besides its high sensitivity, the WSP2110 is also characterized by a detection
range from 1 to 50 ppm for toluene, benzene, methanal, and alcohol. The WSP2110 provides an analog representation of polluted concentration in the air sent directly to an analog pin of the mikroBUS™ socket labeled OUT. The analog output voltage the sensor provides varies in proportion to the toxic gas concentration; the higher the toxic gas concentration in the air, the higher the output voltage. Also, this Click board™ has a built-in potentiometer that allows users to
adjust the load resistance of the MQ-135 circuit for optimum performance. The ENA pin can be used to enable the gas sensor. This Click board™ can be operated only with a 5V logic voltage level. The board must perform appropriate logic voltage level conversion before using MCUs with different logic levels. Also, it comes equipped with a library containing functions and an example code that can be used as a reference for further development.
Features overview
Development board
Clicker 2 for Kinetis is a compact starter development board that brings the flexibility of add-on Click boards™ to your favorite microcontroller, making it a perfect starter kit for implementing your ideas. It comes with an onboard 32-bit ARM Cortex-M4F microcontroller, the MK64FN1M0VDC12 from NXP Semiconductors, two mikroBUS™ sockets for Click board™ connectivity, a USB connector, LED indicators, buttons, a JTAG programmer connector, and two 26-pin headers for interfacing with external electronics. Its compact design with clear and easily recognizable silkscreen markings allows you to build gadgets with unique functionalities and
features quickly. Each part of the Clicker 2 for Kinetis development kit contains the components necessary for the most efficient operation of the same board. In addition to the possibility of choosing the Clicker 2 for Kinetis programming method, using a USB HID mikroBootloader or an external mikroProg connector for Kinetis programmer, the Clicker 2 board also includes a clean and regulated power supply module for the development kit. It provides two ways of board-powering; through the USB Micro-B cable, where onboard voltage regulators provide the appropriate voltage levels to each component on the board, or
using a Li-Polymer battery via an onboard battery connector. All communication methods that mikroBUS™ itself supports are on this board, including the well-established mikroBUS™ socket, reset button, and several user-configurable buttons and LED indicators. Clicker 2 for Kinetis is an integral part of the Mikroe ecosystem, allowing you to create a new application in minutes. Natively supported by Mikroe software tools, it covers many aspects of prototyping thanks to a considerable number of different Click boards™ (over a thousand boards), the number of which is growing every day.
Microcontroller Overview
MCU Card / MCU
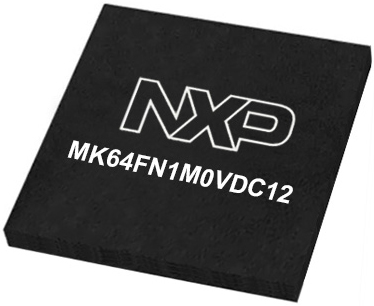
Architecture
ARM Cortex-M4
MCU Memory (KB)
1024
Silicon Vendor
NXP
Pin count
121
RAM (Bytes)
262144
Used MCU Pins
mikroBUS™ mapper
Take a closer look
Click board™ Schematic
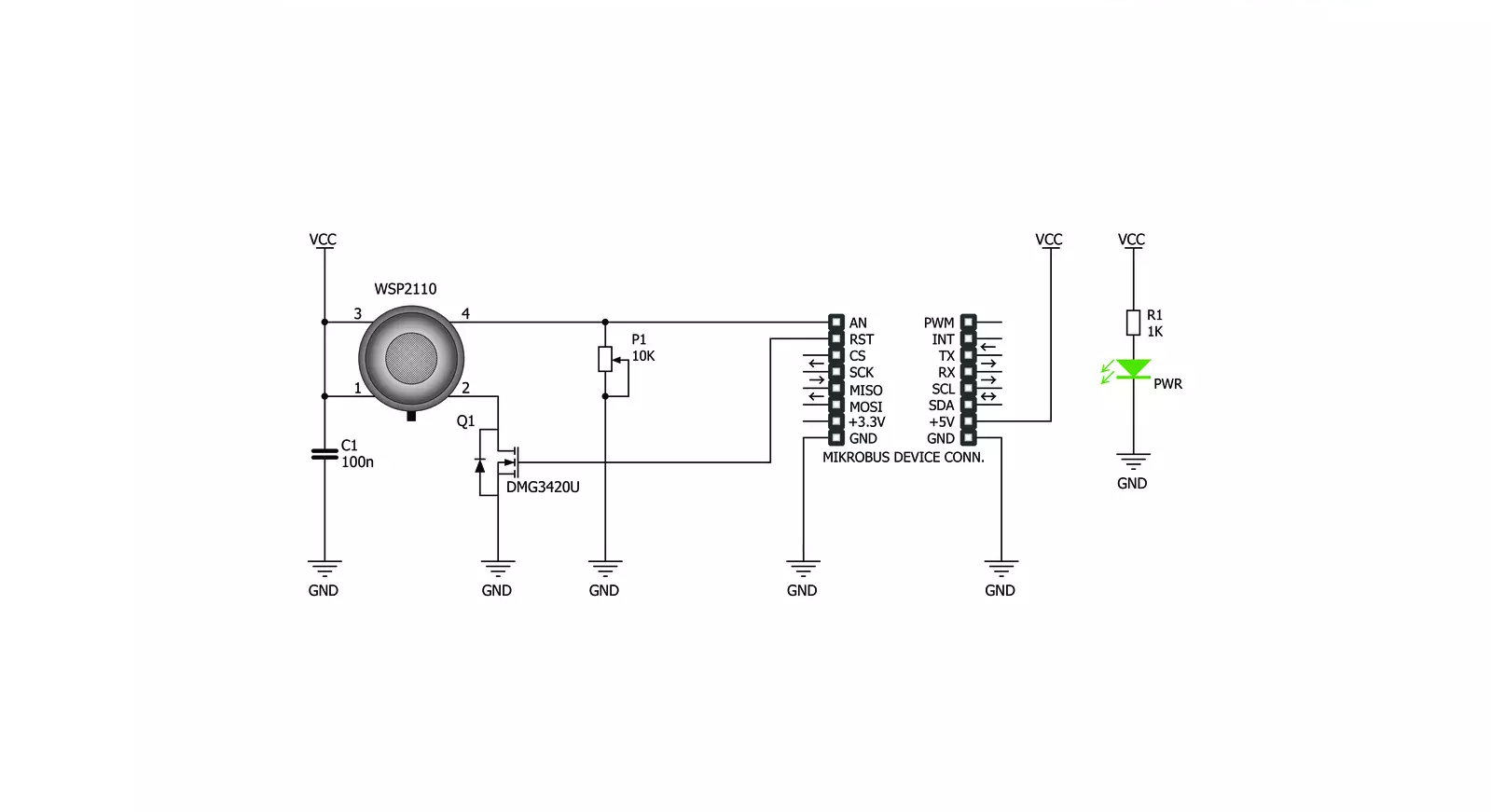
Step by step
Project assembly
Track your results in real time
Application Output
1. Application Output - In Debug mode, the 'Application Output' window enables real-time data monitoring, offering direct insight into execution results. Ensure proper data display by configuring the environment correctly using the provided tutorial.
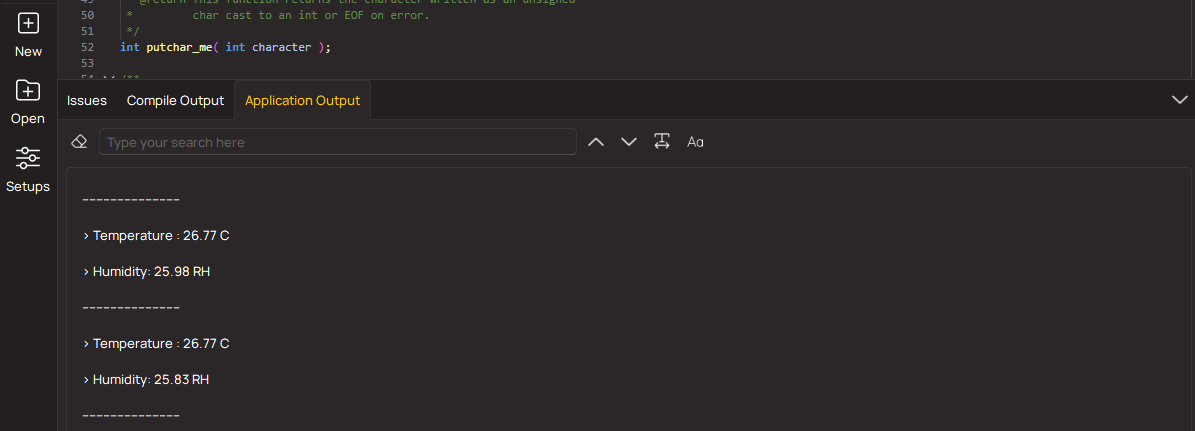
2. UART Terminal - Use the UART Terminal to monitor data transmission via a USB to UART converter, allowing direct communication between the Click board™ and your development system. Configure the baud rate and other serial settings according to your project's requirements to ensure proper functionality. For step-by-step setup instructions, refer to the provided tutorial.
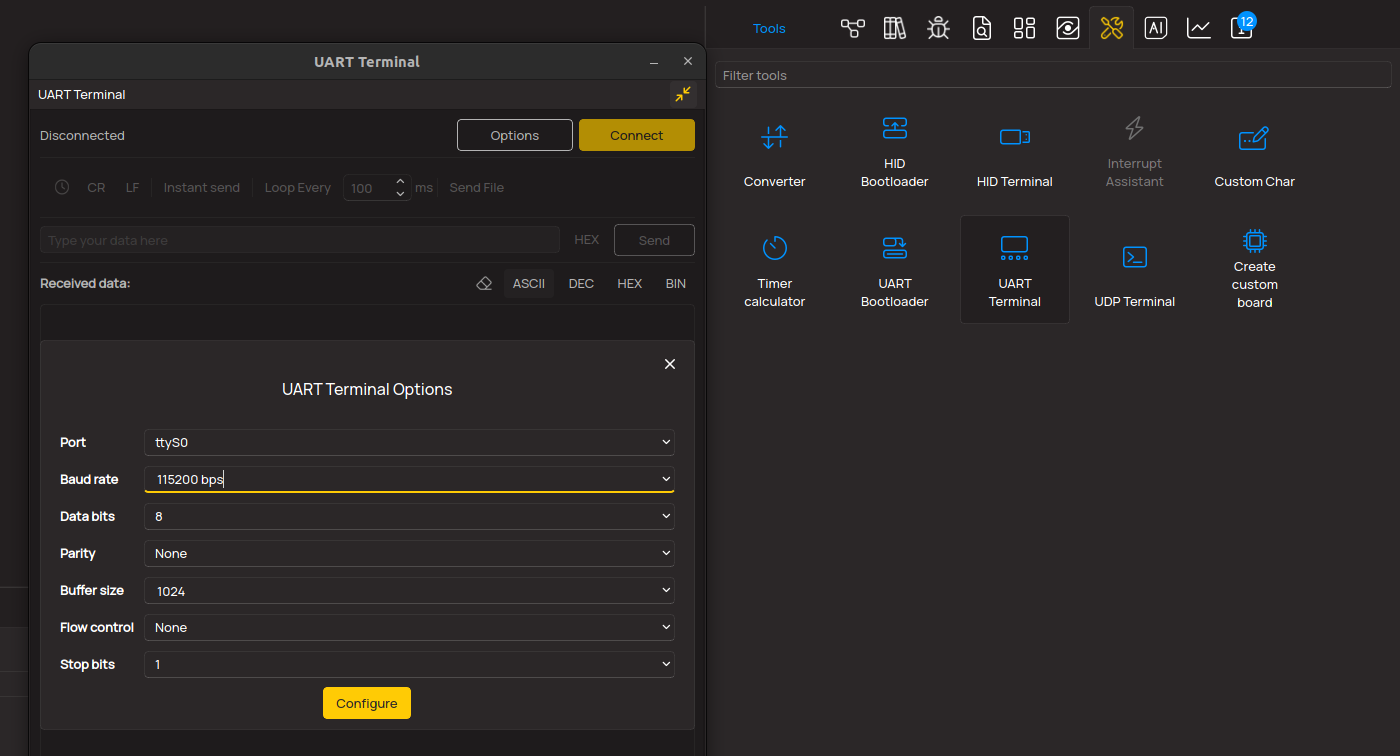
3. Plot Output - The Plot feature offers a powerful way to visualize real-time sensor data, enabling trend analysis, debugging, and comparison of multiple data points. To set it up correctly, follow the provided tutorial, which includes a step-by-step example of using the Plot feature to display Click board™ readings. To use the Plot feature in your code, use the function: plot(*insert_graph_name*, variable_name);. This is a general format, and it is up to the user to replace 'insert_graph_name' with the actual graph name and 'variable_name' with the parameter to be displayed.
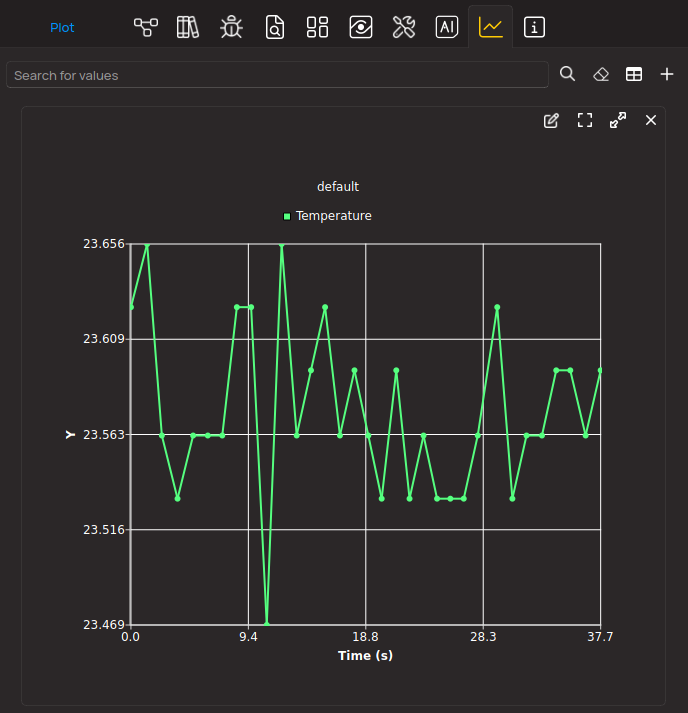
Software Support
Library Description
This library contains API for Pollution Click driver.
Key functions:
pollution_generic_read
- This function read ADC datapollution_measure_load_voltage
- This function gets load voltage from read ADC valuepollution_get_corrected_resistance
- This function gets the corrected resistance of the sensor
Open Source
Code example
The complete application code and a ready-to-use project are available through the NECTO Studio Package Manager for direct installation in the NECTO Studio. The application code can also be found on the MIKROE GitHub account.
/*!
* \file
* \brief Pollution Click example
*
* # Description
* Pollution Click carries the VOC gas sensor and has high sensitivity to organic gases
* such as methanal (also known as formaldehyde), benzene, alcohol, toluene, etc.
*
* The demo application is composed of two sections :
*
* ## Application Init
* Application Init performs Logger and Click initialization.
*
* ## Application Task
* This is an example which demonstrates the usage of Pollution Click board.
* Pollution Click reads ADC value, load voltage from ADC value, and reads corrected
* resistance of the sensor where results are being sent to the UART terminal
* where you can track changes.
*
* \author Mihajlo Djordjevic
*
*/
// ------------------------------------------------------------------- INCLUDES
#include "board.h"
#include "log.h"
#include "pollution.h"
float value_volt;
float value_res;
// ------------------------------------------------------------------ VARIABLES
static pollution_t pollution;
static log_t logger;
// ------------------------------------------------------ APPLICATION FUNCTIONS
void application_init ( void )
{
log_cfg_t log_cfg;
pollution_cfg_t cfg;
/**
* Logger initialization.
* Default baud rate: 115200
* Default log level: LOG_LEVEL_DEBUG
* @note If USB_UART_RX and USB_UART_TX
* are defined as HAL_PIN_NC, you will
* need to define them manually for log to work.
* See @b LOG_MAP_USB_UART macro definition for detailed explanation.
*/
LOG_MAP_USB_UART( log_cfg );
log_init( &logger, &log_cfg );
log_info( &logger, "---- Application Init ----" );
Delay_ms ( 1000 );
// Click initialization.
pollution_cfg_setup( &cfg );
POLLUTION_MAP_MIKROBUS( cfg, MIKROBUS_1 );
pollution_init( &pollution, &cfg );
log_printf( &logger, "-------------------------------------\r\n" );
log_printf( &logger, "---------- Pollution Click ----------\r\n" );
log_printf( &logger, "-------------------------------------\r\n" );
Delay_ms ( 1000 );
pollution_default_cfg( &pollution );
Delay_ms ( 1000 );
log_printf( &logger, "--------- ADC Initializated ---------\r\n" );
log_printf( &logger, "-------------------------------------\r\n" );
Delay_ms ( 1000 );
}
void application_task ( void )
{
pollution_data_t tmp;
tmp = pollution_generic_read( &pollution );
log_printf( &logger, " ADC value : %u ppm\r\n", tmp );
Delay_ms ( 1000 );
value_volt = pollution_measure_load_voltage( &pollution );
log_printf( &logger, " Load voltage : %.2f V\r\n", value_volt );
Delay_ms ( 1000 );
value_res = pollution_get_corrected_resistance( &pollution );
log_printf( &logger, " Corrected resistance : %.2f kOhm\r\n", value_res );
log_printf( &logger, "-------------------------------------\r\n" );
Delay_ms ( 1000 );
}
int main ( void )
{
/* Do not remove this line or clock might not be set correctly. */
#ifdef PREINIT_SUPPORTED
preinit();
#endif
application_init( );
for ( ; ; )
{
application_task( );
}
return 0;
}
// ------------------------------------------------------------------------ END