Maximize space and functionality by choosing our 16-in-1 button integration solution for your control needs
A
A
Hardware Overview
How does it work?
4x4 Key Click is based on 16 buttons with debounce circuits and two MC74HC165A, 8-bit parallel-in/serial-out shift registers from ON Semiconductor. The rightmost column of the keyboard is marked with letters from A to D, while the other 12 buttons are marked like a telephone keypad, so it is easy to implement this 4x4 Click board to any design. The 16-button output lines go straight to the parallel data inputs of the two shift registers connected in a serial (daisy) chain, thus
occupying fewer pins on the host MCU. The shift registers allow you to press all 16 buttons simultaneously, and each will be registered. The 4X4 Click board uses an SPI serial interface to communicate with the host MCU over the mikroBUS™ socket. In this case, the SPI interface saves as many IO pins of the MCU as possible from 16 buttons using shift registers. The Clock Enable pins of the shift registers are not user-configurable and are tied LOW; thus, shift registers are always
enabled. This Click board™ can operate with either 3.3V or 5V logic voltage levels selected via the PWR SEL jumper. This way, both 3.3V and 5V capable MCUs can use the communication lines properly. Also, this Click board™ comes equipped with a library containing easy-to-use functions and an example code that can be used as a reference for further development.
Features overview
Development board
EasyPIC v8 is a development board specially designed for the needs of rapid development of embedded applications. It supports many high pin count 8-bit PIC microcontrollers from Microchip, regardless of their number of pins, and a broad set of unique functions, such as the first-ever embedded debugger/programmer. The development board is well organized and designed so that the end-user has all the necessary elements, such as switches, buttons, indicators, connectors, and others, in one place. Thanks to innovative manufacturing technology, EasyPIC v8 provides a fluid and immersive working experience, allowing access anywhere and under any
circumstances at any time. Each part of the EasyPIC v8 development board contains the components necessary for the most efficient operation of the same board. In addition to the advanced integrated CODEGRIP programmer/debugger module, which offers many valuable programming/debugging options and seamless integration with the Mikroe software environment, the board also includes a clean and regulated power supply module for the development board. It can use a wide range of external power sources, including a battery, an external 12V power supply, and a power source via the USB Type-C (USB-C) connector.
Communication options such as USB-UART, USB DEVICE, and CAN are also included, including the well-established mikroBUS™ standard, two display options (graphical and character-based LCD), and several different DIP sockets. These sockets cover a wide range of 8-bit PIC MCUs, from the smallest PIC MCU devices with only eight up to forty pins. EasyPIC v8 is an integral part of the Mikroe ecosystem for rapid development. Natively supported by Mikroe software tools, it covers many aspects of prototyping and development thanks to a considerable number of different Click boards™ (over a thousand boards), the number of which is growing every day.
Microcontroller Overview
MCU Card / MCU
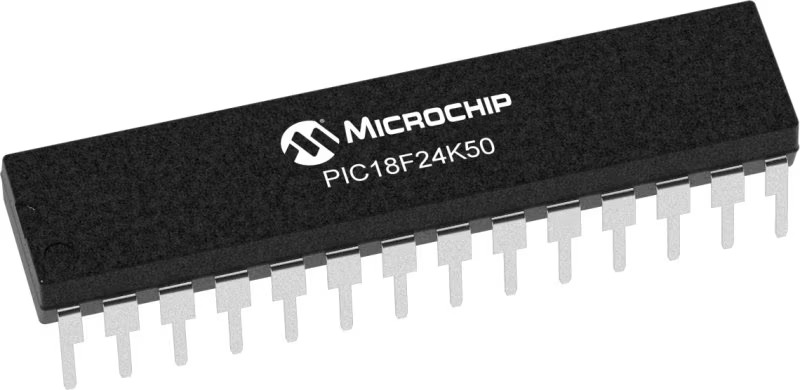
Architecture
PIC
MCU Memory (KB)
16
Silicon Vendor
Microchip
Pin count
28
RAM (Bytes)
2048
Used MCU Pins
mikroBUS™ mapper
Take a closer look
Click board™ Schematic
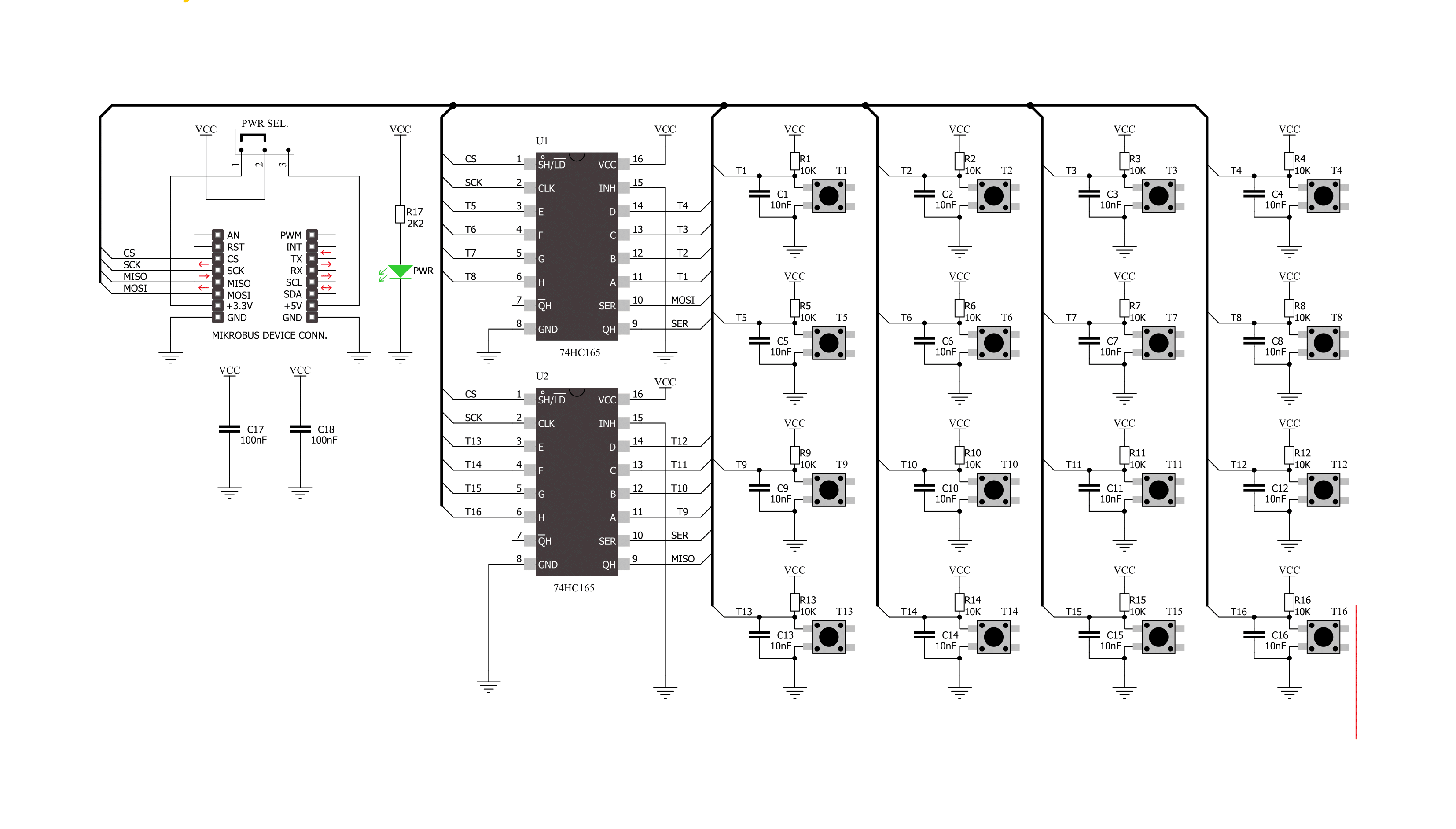
Step by step
Project assembly
Track your results in real time
Application Output
1. Application Output - In Debug mode, the 'Application Output' window enables real-time data monitoring, offering direct insight into execution results. Ensure proper data display by configuring the environment correctly using the provided tutorial.
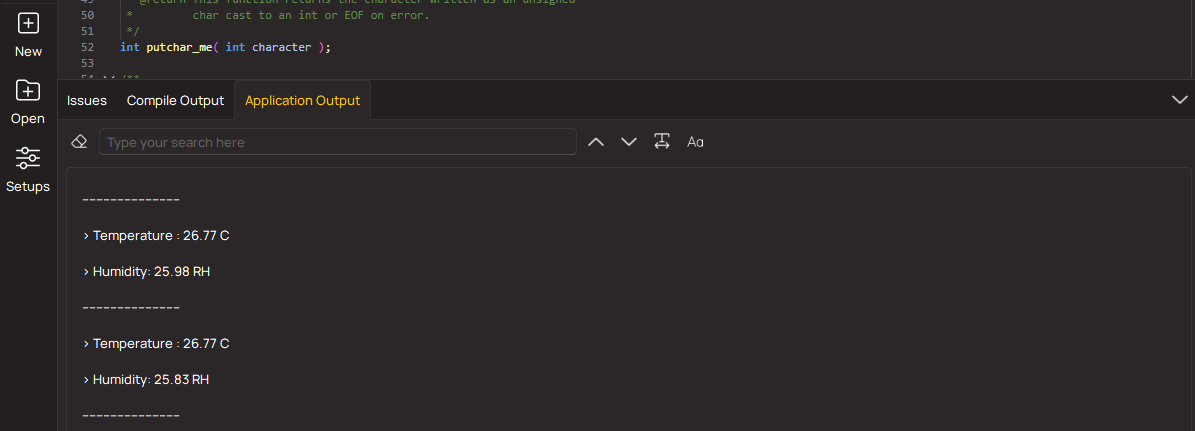
2. UART Terminal - Use the UART Terminal to monitor data transmission via a USB to UART converter, allowing direct communication between the Click board™ and your development system. Configure the baud rate and other serial settings according to your project's requirements to ensure proper functionality. For step-by-step setup instructions, refer to the provided tutorial.
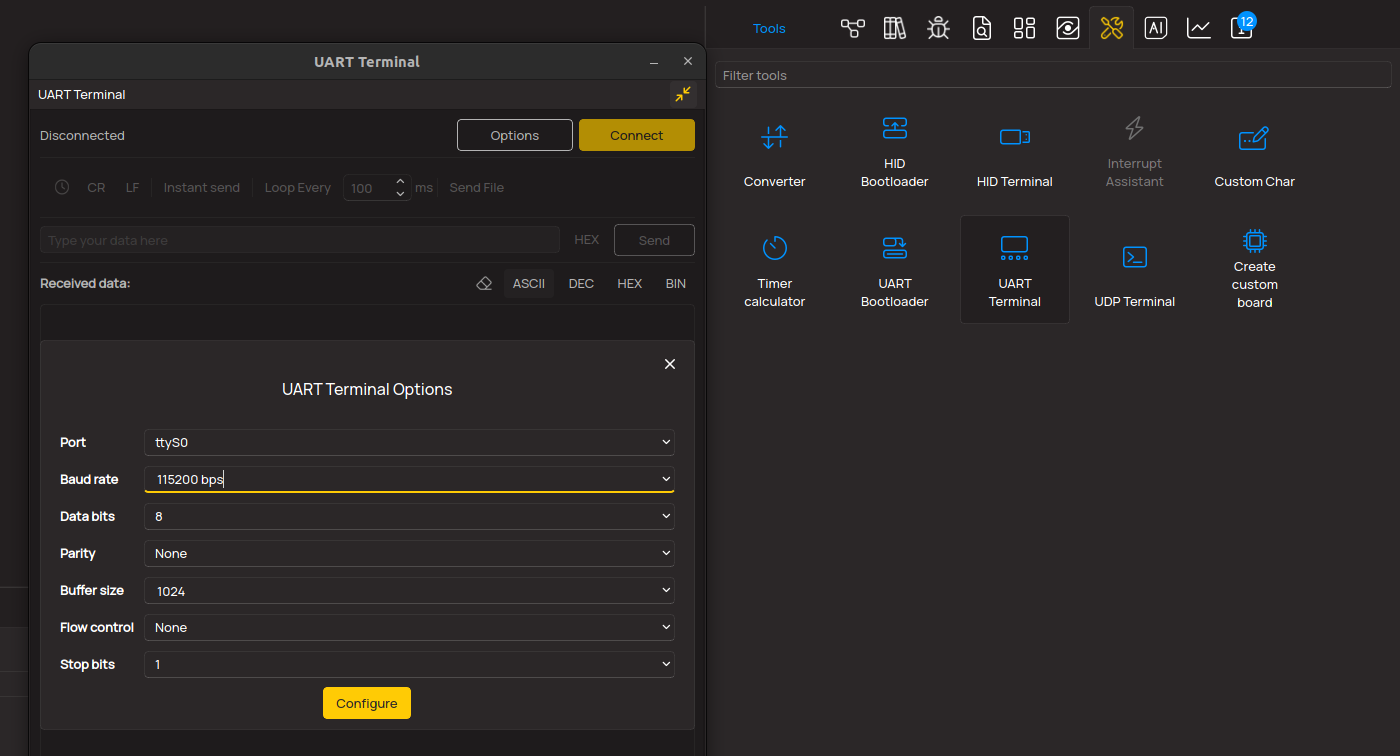
3. Plot Output - The Plot feature offers a powerful way to visualize real-time sensor data, enabling trend analysis, debugging, and comparison of multiple data points. To set it up correctly, follow the provided tutorial, which includes a step-by-step example of using the Plot feature to display Click board™ readings. To use the Plot feature in your code, use the function: plot(*insert_graph_name*, variable_name);. This is a general format, and it is up to the user to replace 'insert_graph_name' with the actual graph name and 'variable_name' with the parameter to be displayed.
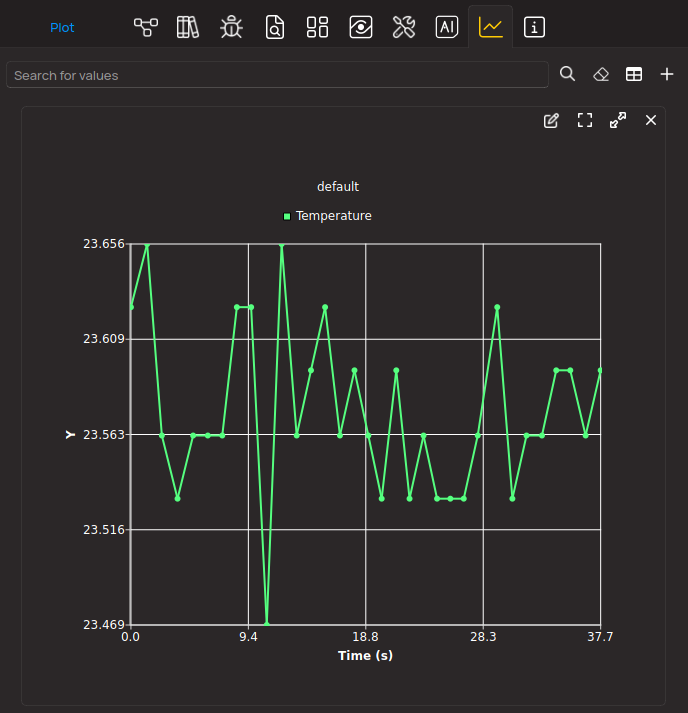
Software Support
Library Description
This library contains API for 4x4 Key Click driver.
Key functions:
c4x4key_get_data
- Get 16-bit data function.c4x4key_get_btn_position
- Get position pressed button function.
Open Source
Code example
The complete application code and a ready-to-use project are available through the NECTO Studio Package Manager for direct installation in the NECTO Studio. The application code can also be found on the MIKROE GitHub account.
/*!
* \file
* \brief 4x4Key Click example
*
* # Description
* The library covers all the necessary functions to control the 4x4 Key Click.
* 4x4 Key Click communicates with the target board via SPI interface.
* This library contains drivers for reading data from a sensor and get
* the position of the pressed button.
*
* The demo application is composed of two sections :
*
* ## Application Init
* Configuring Clicks and log objects.
*
* ## Application Task
* This is a example which demonstrates the use of 4x4 Key Click board.
* Detects and logs whether any of the buttons is pressed.
* Results are being sent to the Usart Terminal
* where you can track their changes.
* All data logs on usb uart when the button is triggered.
*
* \author Nenad Filipovic
*
*/
// ------------------------------------------------------------------- INCLUDES
#include "board.h"
#include "log.h"
#include "c4x4key.h"
// ------------------------------------------------------------------ VARIABLES
static c4x4key_t c4x4key;
static log_t logger;
static uint16_t btn_data_old;
// ------------------------------------------------------ APPLICATION FUNCTIONS
void application_init ( void )
{
log_cfg_t log_cfg;
c4x4key_cfg_t cfg;
/**
* Logger initialization.
* Default baud rate: 115200
* Default log level: LOG_LEVEL_DEBUG
* @note If USB_UART_RX and USB_UART_TX
* are defined as HAL_PIN_NC, you will
* need to define them manually for log to work.
* See @b LOG_MAP_USB_UART macro definition for detailed explanation.
*/
LOG_MAP_USB_UART( log_cfg );
log_init( &logger, &log_cfg );
log_info( &logger, "---- Application Init ----" );
// Click initialization.
c4x4key_cfg_setup( &cfg );
C4X4KEY_MAP_MIKROBUS( cfg, MIKROBUS_1 );
c4x4key_init( &c4x4key, &cfg );
btn_data_old = 0;
log_printf( &logger, " 4x4 Key Click\r\n" );
log_printf( &logger, "--------------------\r\n" );
log_printf( &logger, " Press any button\r\n" );
log_printf( &logger, "--------------------\r\n" );
}
void application_task ( void )
{
uint16_t btn_data;
btn_data = c4x4key_get_data( &c4x4key );
if ( btn_data_old != btn_data )
{
if ( btn_data == C4X4KEY_BUTTON_0 )
{
log_printf( &logger, " 0\r\n" );
}
if ( btn_data == C4X4KEY_BUTTON_1 )
{
log_printf( &logger, " 1\r\n" );
}
if ( btn_data == C4X4KEY_BUTTON_2 )
{
log_printf( &logger, " 2\r\n" );
}
if ( btn_data == C4X4KEY_BUTTON_3 )
{
log_printf( &logger, " 3\r\n" );
}
if ( btn_data == C4X4KEY_BUTTON_4 )
{
log_printf( &logger, " 4\r\n" );
}
if ( btn_data == C4X4KEY_BUTTON_5 )
{
log_printf( &logger, " 5\r\n" );
}
if ( btn_data == C4X4KEY_BUTTON_6 )
{
log_printf( &logger, " 6\r\n" );
}
if ( btn_data == C4X4KEY_BUTTON_7 )
{
log_printf( &logger, " 7\r\n" );
}
if ( btn_data == C4X4KEY_BUTTON_8 )
{
log_printf( &logger, " 8\r\n" );
}
if ( btn_data == C4X4KEY_BUTTON_9 )
{
log_printf( &logger, " 9\r\n" );
}
if ( btn_data == C4X4KEY_BUTTON_A )
{
log_printf( &logger, " A\r\n" );
}
if ( btn_data == C4X4KEY_BUTTON_B )
{
log_printf( &logger, " B\r\n" );
}
if ( btn_data == C4X4KEY_BUTTON_C )
{
log_printf( &logger, " C\r\n" );
}
if ( btn_data == C4X4KEY_BUTTON_D )
{
log_printf( &logger, " D\r\n" );
}
if ( btn_data == C4X4KEY_BUTTON_STAR )
{
log_printf( &logger, " *\r\n" );
}
if ( btn_data == C4X4KEY_BUTTON_HASH )
{
log_printf( &logger, " #\r\n" );
}
btn_data_old = btn_data;
}
Delay_10ms();
}
int main ( void )
{
/* Do not remove this line or clock might not be set correctly. */
#ifdef PREINIT_SUPPORTED
preinit();
#endif
application_init( );
for ( ; ; )
{
application_task( );
}
return 0;
}
// ------------------------------------------------------------------------ END
Additional Support
Resources
Category:Pushbutton/Switches