Seamlessly bridge analog and digital realms using our digital potentiometer technology, harmonizing control and responsiveness for superior outcomes
A
A
Hardware Overview
How does it work?
DIGI POT 6 Click is based on the MCP41HV51, 8-bit dual power rails digital potentiometer with SPI serial interface and volatile memory from Microchip. It has a wide operating voltage range, analog from 10 to 36V and digital from 2.7 to 5.5V, or is implemented as a dual-rail (±18V) for systems requiring wide signal swing or high power-supply voltages. It supports resistor configurations of 255 resistors and 256 steps and high terminal/wiper current, including the ability to sink/source up to 25mA on all terminal pins for driving larger loads. The resistor network of the MCP41HV51 has an 8-bit resolution where each resistor network allows
Zero-Scale to Full-Scale connections. All these features, combined with an extended temperature range, make the MCP41HV51 well-suited for a broad range of high-voltage and high-temperature applications, including those in the industrial, automotive, and audio markets. DIGI POT 6 click communicates with MCU using the SPI serial interface with a maximum frequency 10MHz and supports the two most common SPI modes, 0 and 3. This Click board™ also has three terminals labeled P0A, P0B, and P0W, with an internal architecture comprising various resistances and switches. The resistance between
terminals A and B, RAB, commonly called the “end-to-end” resistance, provides RAB resistance options up to 100 kΩ. In contrast, the wiper terminal, P0W, is digitally programmable to access any 2n tap points on the resistor string. This Click board™ can operate with either 3.3V or 5V logic voltage levels selected via the VCC SEL jumper. This way, both 3.3V and 5V capable MCUs can use the communication lines properly. Also, this Click board™ comes equipped with a library containing easy-to-use functions and an example code that can be used, as a reference, for further development.
Features overview
Development board
Curiosity HPC, standing for Curiosity High Pin Count (HPC) development board, supports 28- and 40-pin 8-bit PIC MCUs specially designed by Microchip for the needs of rapid development of embedded applications. This board has two unique PDIP sockets, surrounded by dual-row expansion headers, allowing connectivity to all pins on the populated PIC MCUs. It also contains a powerful onboard PICkit™ (PKOB), eliminating the need for an external programming/debugging tool, two mikroBUS™ sockets for Click board™ connectivity, a USB connector, a set of indicator LEDs, push button switches and a variable potentiometer. All
these features allow you to combine the strength of Microchip and Mikroe and create custom electronic solutions more efficiently than ever. Each part of the Curiosity HPC development board contains the components necessary for the most efficient operation of the same board. An integrated onboard PICkit™ (PKOB) allows low-voltage programming and in-circuit debugging for all supported devices. When used with the MPLAB® X Integrated Development Environment (IDE, version 3.0 or higher) or MPLAB® Xpress IDE, in-circuit debugging allows users to run, modify, and troubleshoot their custom software and hardware
quickly without the need for additional debugging tools. Besides, it includes a clean and regulated power supply block for the development board via the USB Micro-B connector, alongside all communication methods that mikroBUS™ itself supports. Curiosity HPC development board allows you to create a new application in just a few steps. Natively supported by Microchip software tools, it covers many aspects of prototyping thanks to many number of different Click boards™ (over a thousand boards), the number of which is growing daily.
Microcontroller Overview
MCU Card / MCU
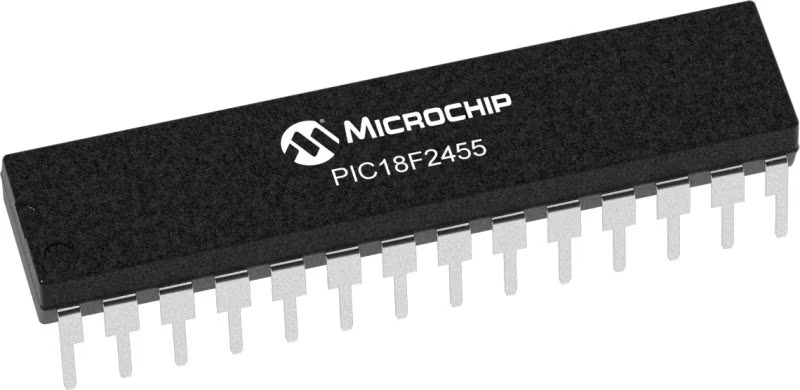
Architecture
PIC
MCU Memory (KB)
24
Silicon Vendor
Microchip
Pin count
28
RAM (Bytes)
2048
Used MCU Pins
mikroBUS™ mapper
Take a closer look
Click board™ Schematic
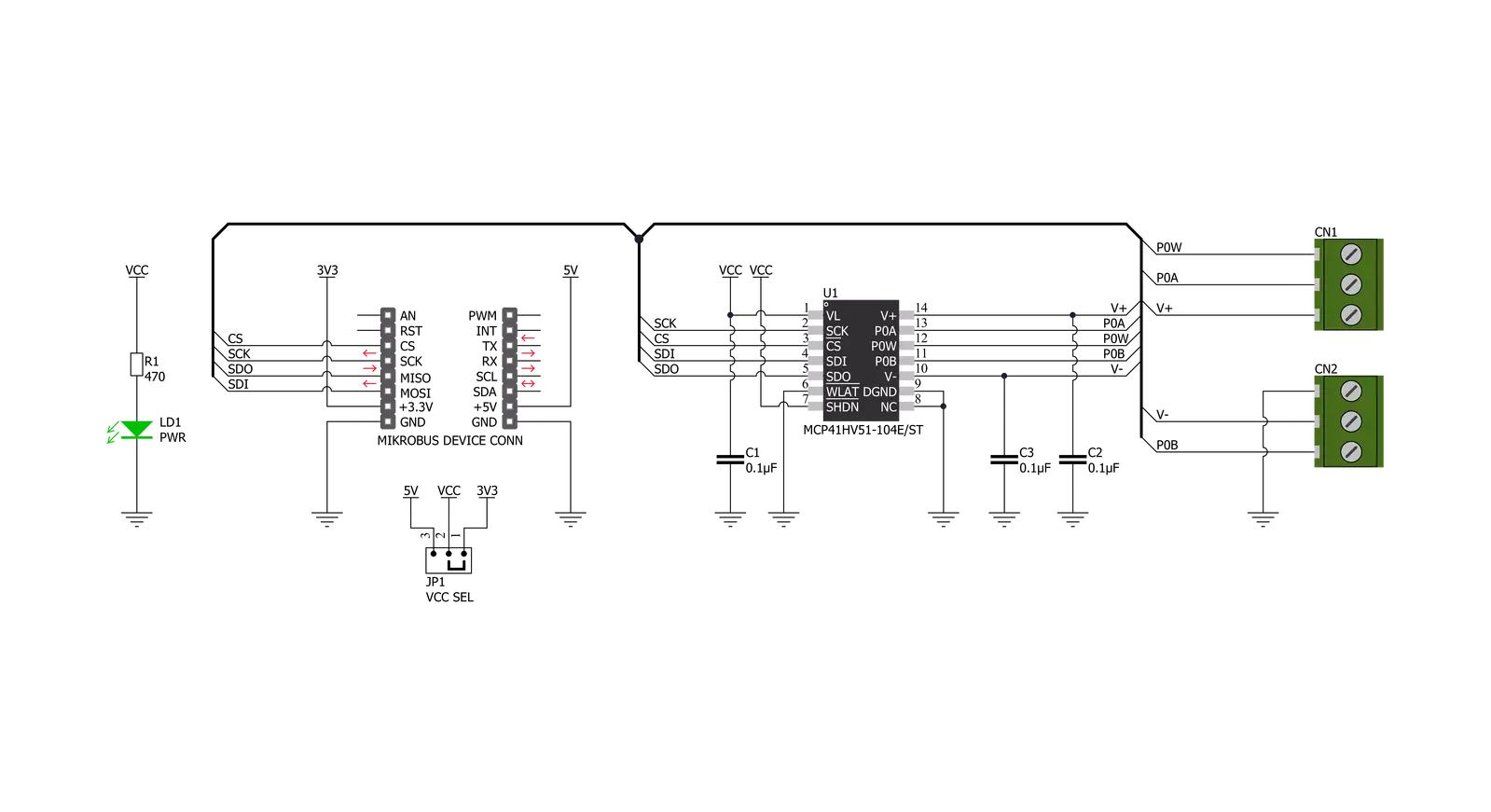
Step by step
Project assembly
Track your results in real time
Application Output via Debug Mode
1. Once the code example is loaded, pressing the "DEBUG" button initiates the build process, programs it on the created setup, and enters Debug mode.
2. After the programming is completed, a header with buttons for various actions within the IDE becomes visible. Clicking the green "PLAY" button starts reading the results achieved with the Click board™. The achieved results are displayed in the Application Output tab.
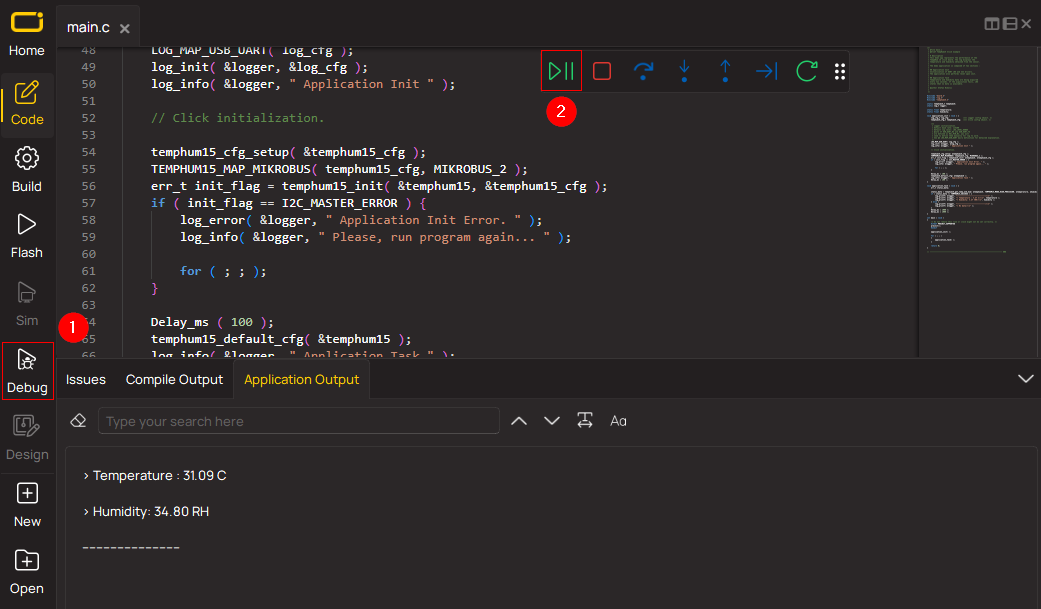
Software Support
Library Description
This library contains API for DIGI POT 6 Click driver.
Key functions:
digipot6_read_data
- This function reads data from the specified register addressdigipot6_write_wiper_cmd
- This function writes a wiper configuration command to the click moduledigipot6_set_resistor
- This function reads data from the specified register address
Open Source
Code example
The complete application code and a ready-to-use project are available through the NECTO Studio Package Manager for direct installation in the NECTO Studio. The application code can also be found on the MIKROE GitHub account.
/*!
* \file
* \brief DIGIPOT6 Click example
*
* # Description
* This example showcases how to initialize, configure and use the DIGI POT 6 click module. The
* click is a digital potentiometer. The potentiometer has a programmable wiper which controls
* the resistance between P0W-POA and POW-POB. An external power supply is required for this example.
*
* The demo application is composed of two sections :
*
* ## Application Init
* This function initializes and configures the logger and click modules. This function also sets
* the click default configuration.
*
* ## Application Task
* This function programs the wiper position and shows the current wiper position in the UART
* console every second.
*
* \author MikroE Team
*
*/
// ------------------------------------------------------------------- INCLUDES
#include "board.h"
#include "log.h"
#include "digipot6.h"
// ------------------------------------------------------------------ VARIABLES
static digipot6_t digipot6;
static log_t logger;
// ------------------------------------------------------ APPLICATION FUNCTIONS
void application_init ( void )
{
log_cfg_t log_cfg;
digipot6_cfg_t cfg;
/**
* Logger initialization.
* Default baud rate: 115200
* Default log level: LOG_LEVEL_DEBUG
* @note If USB_UART_RX and USB_UART_TX
* are defined as HAL_PIN_NC, you will
* need to define them manually for log to work.
* See @b LOG_MAP_USB_UART macro definition for detailed explanation.
*/
LOG_MAP_USB_UART( log_cfg );
log_init( &logger, &log_cfg );
log_info( &logger, "---- Application Init ----" );
// Click initialization.
digipot6_cfg_setup( &cfg );
DIGIPOT6_MAP_MIKROBUS( cfg, MIKROBUS_1 );
digipot6_init( &digipot6, &cfg );
Delay_100ms( );
digipot6_default_cfg( &digipot6 );
Delay_100ms( );
}
void application_task ( void )
{
uint8_t wiper;
uint16_t cnt;
for ( cnt = 0; cnt <= 255; cnt += 15 )
{
digipot6_write_data( &digipot6, DIGIPOT6_VOLATILE_WIPER_0, cnt );
Delay_ms( 10 );
wiper = digipot6_read_data( &digipot6, DIGIPOT6_VOLATILE_WIPER_0 );
log_printf( &logger, " * Wiper position: %u *\r\n", ( uint16_t ) wiper );
Delay_ms( 1000 );
}
}
void main ( void )
{
application_init( );
for ( ; ; )
{
application_task( );
}
}
// ------------------------------------------------------------------------ END