Your system's best friend, the Watchdog Timer, tirelessly monitors and resets your device when it detects abnormal behavior, guaranteeing consistent performance.
A
A
Hardware Overview
How does it work?
Watchdog Click is based on the TPS3430, a standalone watchdog timer with a programmable watchdog window and reset delay from Texas Instruments. This high-accuracy programmable timer with the disable feature achieves 15% watchdog timing accuracy over the extended temperature range. A window watchdog is typically employed in safety-critical applications where a traditional watchdog timer is inadequate. With a traditional timer, there is a maximum time in which a pulse must be issued to prevent the reset from occurring. However, in a window watchdog, the pulse must be issued between a maximum lower window time and the minimum upper window time set by the programmable timeout pin and two logic input pins. This Click board™ communicates with MCU using several GPIO pins and offers programmable watchdog timeout and reset delay. The two pins that this
Click board™ uses represent a watchdog input and output, with two additional logic inputs with whom the user can select the watchdog window ratios timeouts and turn off the watchdog timer. The watchdog input pin labeled as WDI routed on the PWM pin of the mikroBUS™ socket is ignored for the watchdog reset delay upon Startup. After Startup, the watchdog input signal must arrive within the watchdog window to prevent a watchdog reset whose delay duration may be configured with the CRST SEL on-board jumper. The user has two options: leaving the CRST pin pulled high with a pull-up resistor or connecting the CRST to a capacitor connected to GND. Similarly to the watchdog reset delay, the user can configure the watchdog timeout using the S0 and S1 pins, routed on the RST and CS pins of the mikroBUS™ socket and CWD SEL on-board jumper. This jumper can connect the CRST pin
with a pull-up resistor or a capacitor connected to GND. When a watchdog fault occurs due to an incorrectly timed watchdog input signal, the WDO pin activates and performs the transition to logic low state for the watchdog reset delay, indicated with a red LED labeled WDT FLT. When the delay expires, the WDO pin deactivates and returns to a logic high state. When the watchdog is disabled using S0 and S1 pins, the watchdog input is ignored, and the WDO pin is in a Hi-Z and remains logic-high due to the R5 external pull-up resistor. This Click board™ can operate with either 3.3V or 5V logic voltage levels selected via the VCC SEL jumper. This way, both 3.3V and 5V capable MCUs can use the communication lines properly. Also, this Click board™ comes equipped with a library containing easy-to-use functions and an example code that can be used as a reference for further development.
Features overview
Development board
EasyPIC v8 is a development board specially designed for the needs of rapid development of embedded applications. It supports many high pin count 8-bit PIC microcontrollers from Microchip, regardless of their number of pins, and a broad set of unique functions, such as the first-ever embedded debugger/programmer. The development board is well organized and designed so that the end-user has all the necessary elements, such as switches, buttons, indicators, connectors, and others, in one place. Thanks to innovative manufacturing technology, EasyPIC v8 provides a fluid and immersive working experience, allowing access anywhere and under any
circumstances at any time. Each part of the EasyPIC v8 development board contains the components necessary for the most efficient operation of the same board. In addition to the advanced integrated CODEGRIP programmer/debugger module, which offers many valuable programming/debugging options and seamless integration with the Mikroe software environment, the board also includes a clean and regulated power supply module for the development board. It can use a wide range of external power sources, including a battery, an external 12V power supply, and a power source via the USB Type-C (USB-C) connector.
Communication options such as USB-UART, USB DEVICE, and CAN are also included, including the well-established mikroBUS™ standard, two display options (graphical and character-based LCD), and several different DIP sockets. These sockets cover a wide range of 8-bit PIC MCUs, from the smallest PIC MCU devices with only eight up to forty pins. EasyPIC v8 is an integral part of the Mikroe ecosystem for rapid development. Natively supported by Mikroe software tools, it covers many aspects of prototyping and development thanks to a considerable number of different Click boards™ (over a thousand boards), the number of which is growing every day.
Microcontroller Overview
MCU Card / MCU
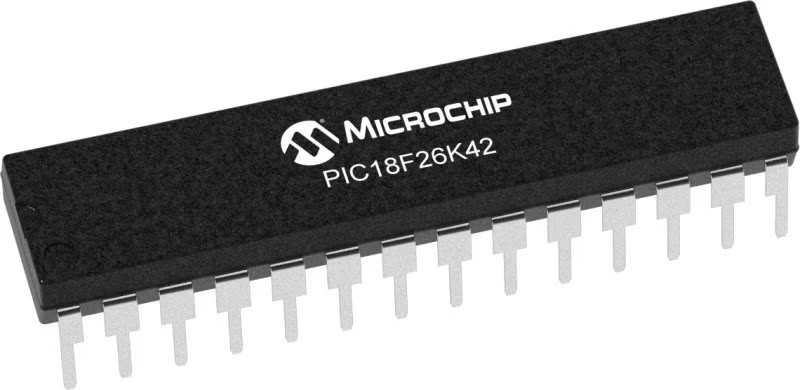
Architecture
PIC
MCU Memory (KB)
64
Silicon Vendor
Microchip
Pin count
28
RAM (Bytes)
4096
Used MCU Pins
mikroBUS™ mapper
Take a closer look
Click board™ Schematic
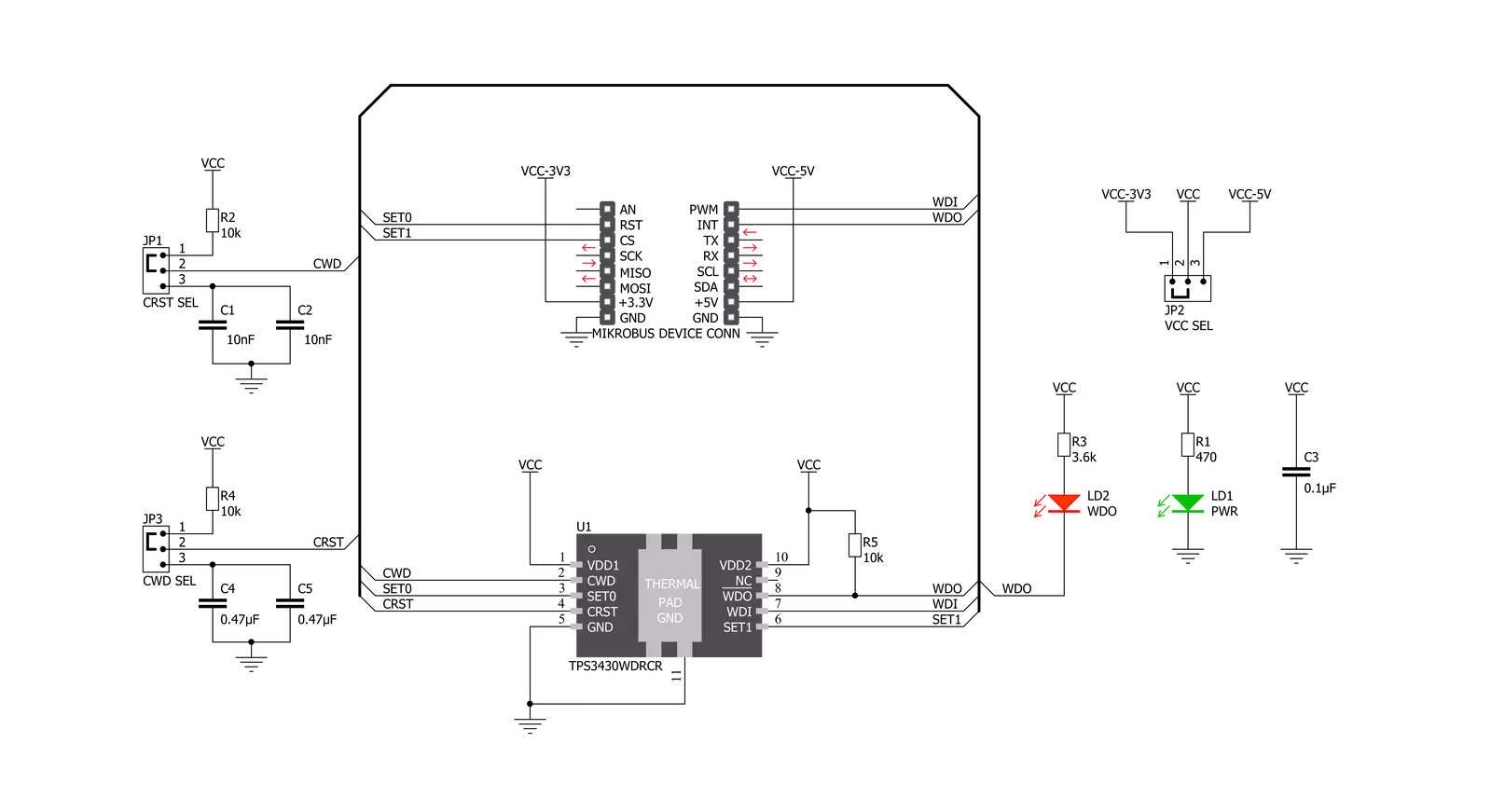
Step by step
Project assembly
Track your results in real time
Application Output
1. Application Output - In Debug mode, the 'Application Output' window enables real-time data monitoring, offering direct insight into execution results. Ensure proper data display by configuring the environment correctly using the provided tutorial.
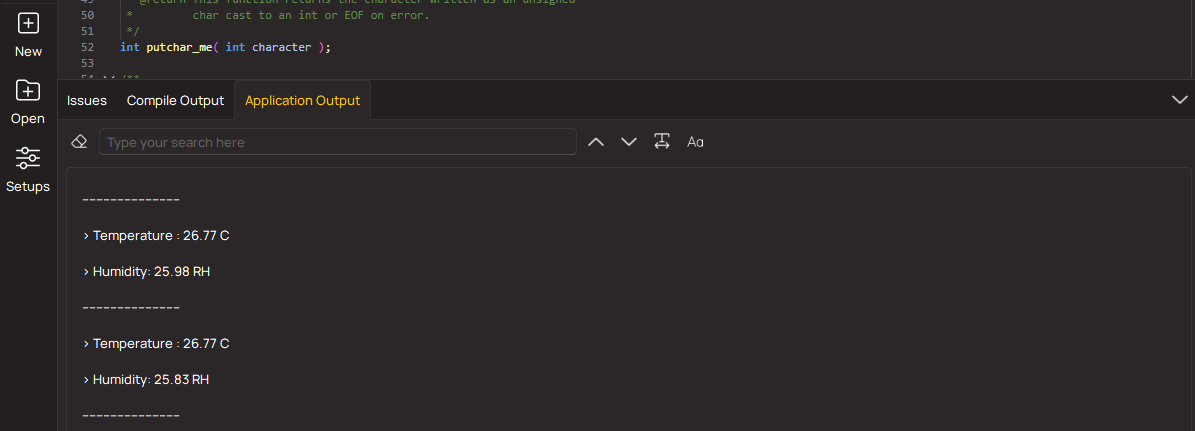
2. UART Terminal - Use the UART Terminal to monitor data transmission via a USB to UART converter, allowing direct communication between the Click board™ and your development system. Configure the baud rate and other serial settings according to your project's requirements to ensure proper functionality. For step-by-step setup instructions, refer to the provided tutorial.
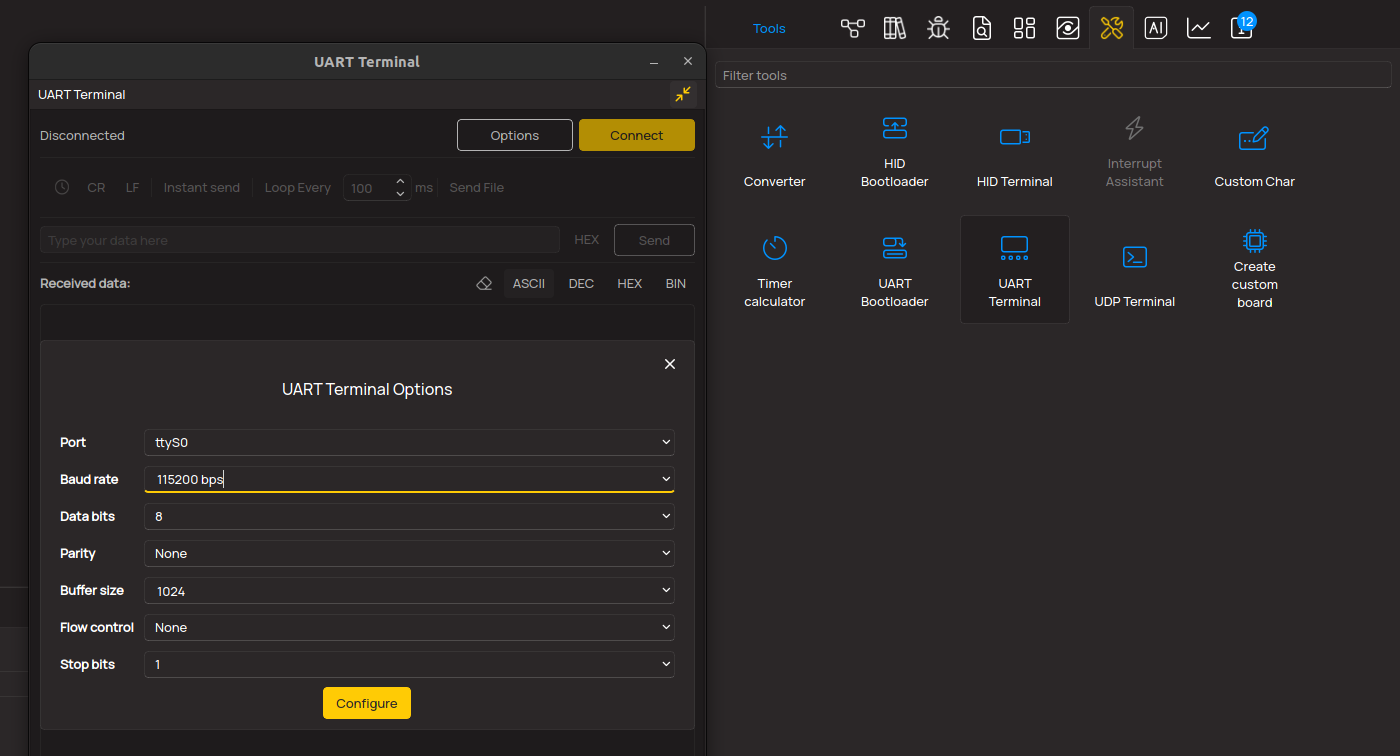
3. Plot Output - The Plot feature offers a powerful way to visualize real-time sensor data, enabling trend analysis, debugging, and comparison of multiple data points. To set it up correctly, follow the provided tutorial, which includes a step-by-step example of using the Plot feature to display Click board™ readings. To use the Plot feature in your code, use the function: plot(*insert_graph_name*, variable_name);. This is a general format, and it is up to the user to replace 'insert_graph_name' with the actual graph name and 'variable_name' with the parameter to be displayed.
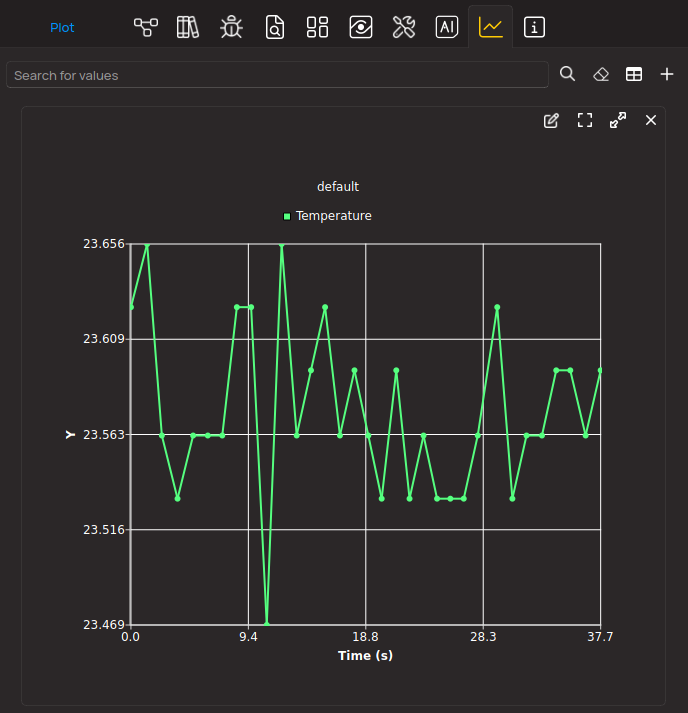
Software Support
Library Description
This library contains API for Watchdog Click driver.
Key functions:
watchdog_set_set0
- Set S0 ( RST ) pin state function.watchdog_get_wdo
- Get WDO ( INT ) pin state function.watchdog_send_pulse
- Send pulse function.
Open Source
Code example
The complete application code and a ready-to-use project are available through the NECTO Studio Package Manager for direct installation in the NECTO Studio. The application code can also be found on the MIKROE GitHub account.
/*!
* @file main.c
* @brief Watchdog Click Example.
*
* # Description
* This is an example that demonstrates the use of the Watchdog click board.
*
* The demo application is composed of two sections :
*
* ## Application Init
* Initialization driver enables - GPIO, configure the watchdog window,
* enable watchdog, also write log.
*
* ## Application Task
* In the first part of the example,
* we send pulses in a valid time window (Correct Operation).
* The second part of the example sends pulses outside the valid time window
* and then the watchdog detects a fault condition, display "Fault",
* performs the reset and turn on the LED ( WDT FLT ) on the Watchdog click board.
* Results are being sent to the Usart Terminal where you can track their changes.
*
*
* @author Stefan Ilic
*
*/
#include "board.h"
#include "log.h"
#include "watchdog.h"
static watchdog_t watchdog; /**< Watchdog Click driver object. */
static log_t logger; /**< Logger object. */
void application_init ( void )
{
log_cfg_t log_cfg; /**< Logger config object. */
watchdog_cfg_t watchdog_cfg; /**< Click config object. */
/**
* Logger initialization.
* Default baud rate: 115200
* Default log level: LOG_LEVEL_DEBUG
* @note If USB_UART_RX and USB_UART_TX
* are defined as HAL_PIN_NC, you will
* need to define them manually for log to work.
* See @b LOG_MAP_USB_UART macro definition for detailed explanation.
*/
LOG_MAP_USB_UART( log_cfg );
log_init( &logger, &log_cfg );
log_info( &logger, " Application Init " );
// Click initialization.
watchdog_cfg_setup( &watchdog_cfg );
WATCHDOG_MAP_MIKROBUS( watchdog_cfg, MIKROBUS_1 );
if ( DIGITAL_OUT_UNSUPPORTED_PIN == watchdog_init( &watchdog, &watchdog_cfg ) ) {
log_error( &logger, " Application Init Error. " );
log_info( &logger, " Please, run program again... " );
for ( ; ; );
}
watchdog_default_cfg ( &watchdog );
log_printf( &logger, "---------------------\r\n" );
log_printf( &logger, " Configure of the \r\n" );
log_printf( &logger, " watchdog window \r\n" );
watchdog_setup_time( &watchdog, WATCHDOG_SETUP_TIME_MODE_2 );
Delay_ms( 1000 );
log_printf( &logger, "---------------------\r\n" );
log_printf( &logger, " Watchdog enabled \r\n" );
log_printf( &logger, "---------------------\r\n" );
Delay_ms( 1000 );
log_info( &logger, " Application Task " );
}
void application_task ( void )
{
log_printf( &logger, " Correct Operation \r\n" );
uint8_t n_cnt = 40;
while ( n_cnt > 0 ) {
watchdog_send_pulse( &watchdog, 1 );
Delay_ms( 50 );
n_cnt--;
}
log_printf( &logger, "---------------------\r\n" );
log_printf( &logger, " Fault \r\n" );
n_cnt = 8;
while ( n_cnt > 0 ) {
watchdog_send_pulse( &watchdog, 1 );
Delay_ms( 250 );
n_cnt--;
}
log_printf( &logger, "---------------------\r\n" );
}
void main ( void )
{
application_init( );
for ( ; ; ) {
application_task( );
}
}
// ------------------------------------------------------------------------ END