Experience intuitive interaction like never before by integrating responsive touch controls into your projects for enhanced user experiences and functionality
A
A
Hardware Overview
How does it work?
CapSense Click is based on the CY8C201A0, a multi-channel capacitive touch sensor from Infineon Technologies. The CY8C201A0 takes human body capacitance as an input and directly provides real-time sensor information via a serial interface. The user can also configure registers with parameters needed to adjust the operation and sensitivity of the CapSense touch buttons and slider and permanently store the settings. As mentioned earlier, this board contains a 5-segment capacitive sensing slider that can detect a slide in either the UP or DOWN direction, as well as two touch button pads which are the only elements on the top side of the board. Each of these touch button pads has a
LED indicator representing the activity in that field. If a touch event is detected on one of these onboard pads, the state of the corresponding LED will be changed, indicating an activated channel; more precisely, touch has been detected on that specific field. CapSense Click communicates with MCU using the standard I2C 2-Wire interface to read data and configure settings. The CY8C201A0 contains multiple operating modes: Active, Periodic Sleep, and Deep Sleep Mode, to meet different power consumption requirements. In the case of using some of the existing Sleep modes, the user is provided with the possibility of controlling these states via the GPO pin, routed to the AN pin of the
mikroBUS™ socket, or this pin can be set in software as an interrupt pin indicating when a specific interrupt event occurs (touch detection). Besides, a Reset pin, routed to the RST pin of the mikroBUS™ socket, causes all operations of the CY8C201A0s CPU and blocks to stop and return to a pre-defined state. This Click board™ can operate with either 3.3V or 5V logic voltage levels selected via the PWR SEL jumper. This way, both 3.3V and 5V capable MCUs can use the communication lines properly. However, the Click board™ comes equipped with a library containing easy-to-use functions and an example code that can be used, as a reference, for further development.
Features overview
Development board
PIC18F57Q43 Curiosity Nano evaluation kit is a cutting-edge hardware platform designed to evaluate microcontrollers within the PIC18-Q43 family. Central to its design is the inclusion of the powerful PIC18F57Q43 microcontroller (MCU), offering advanced functionalities and robust performance. Key features of this evaluation kit include a yellow user LED and a responsive
mechanical user switch, providing seamless interaction and testing. The provision for a 32.768kHz crystal footprint ensures precision timing capabilities. With an onboard debugger boasting a green power and status LED, programming and debugging become intuitive and efficient. Further enhancing its utility is the Virtual serial port (CDC) and a debug GPIO channel (DGI
GPIO), offering extensive connectivity options. Powered via USB, this kit boasts an adjustable target voltage feature facilitated by the MIC5353 LDO regulator, ensuring stable operation with an output voltage ranging from 1.8V to 5.1V, with a maximum output current of 500mA, subject to ambient temperature and voltage constraints.
Microcontroller Overview
MCU Card / MCU
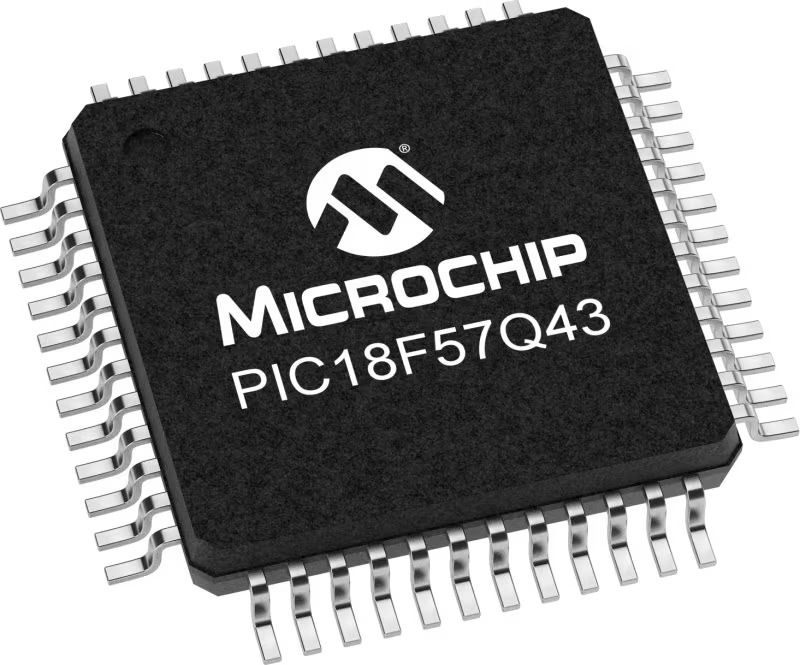
Architecture
PIC
MCU Memory (KB)
128
Silicon Vendor
Microchip
Pin count
48
RAM (Bytes)
8196
You complete me!
Accessories
Curiosity Nano Base for Click boards is a versatile hardware extension platform created to streamline the integration between Curiosity Nano kits and extension boards, tailored explicitly for the mikroBUS™-standardized Click boards and Xplained Pro extension boards. This innovative base board (shield) offers seamless connectivity and expansion possibilities, simplifying experimentation and development. Key features include USB power compatibility from the Curiosity Nano kit, alongside an alternative external power input option for enhanced flexibility. The onboard Li-Ion/LiPo charger and management circuit ensure smooth operation for battery-powered applications, simplifying usage and management. Moreover, the base incorporates a fixed 3.3V PSU dedicated to target and mikroBUS™ power rails, alongside a fixed 5.0V boost converter catering to 5V power rails of mikroBUS™ sockets, providing stable power delivery for various connected devices.
Used MCU Pins
mikroBUS™ mapper
Take a closer look
Schematic
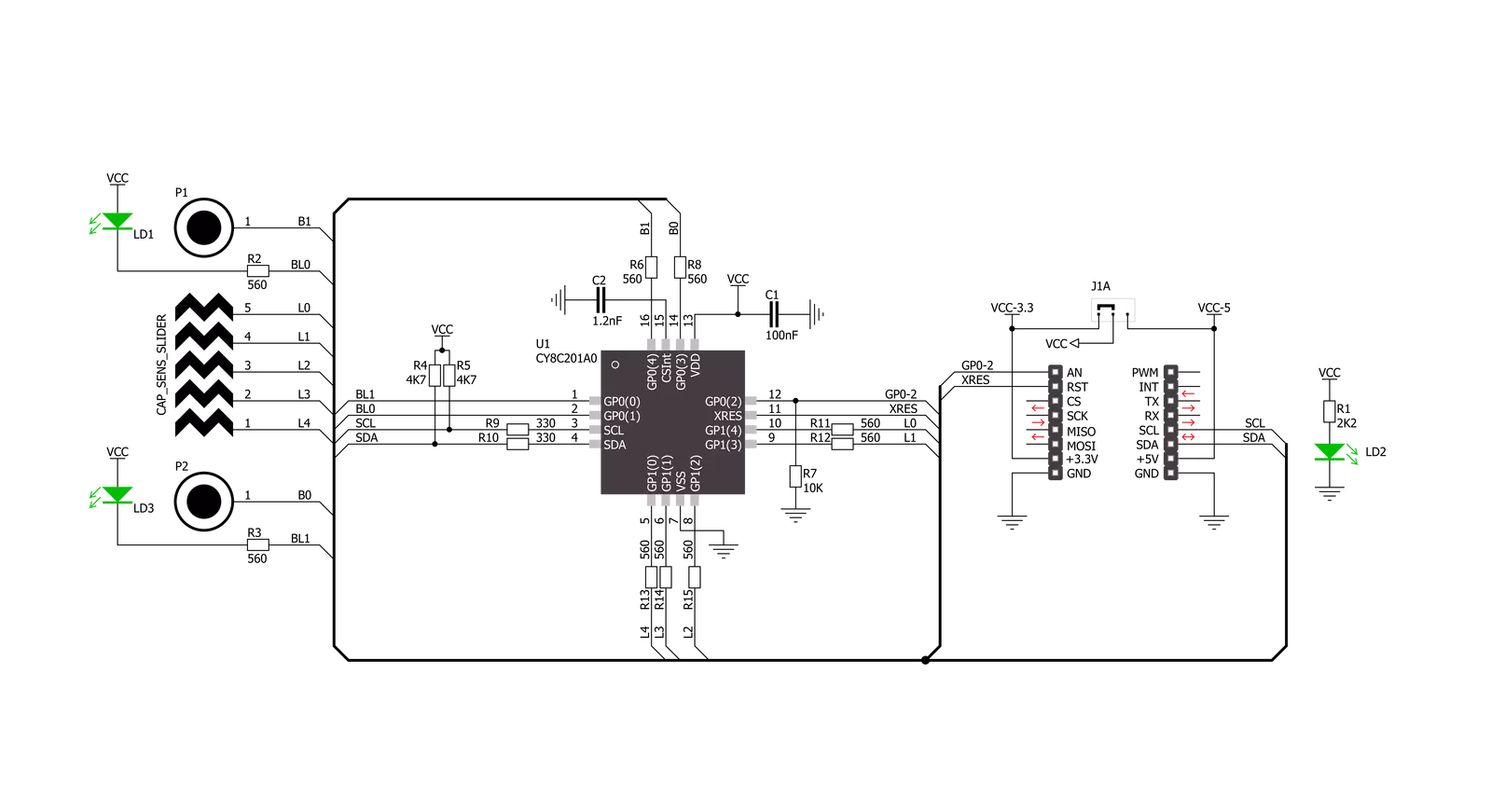
Step by step
Project assembly
Track your results in real time
Application Output via Debug Mode
1. Once the code example is loaded, pressing the "DEBUG" button initiates the build process, programs it on the created setup, and enters Debug mode.
2. After the programming is completed, a header with buttons for various actions within the IDE becomes visible. Clicking the green "PLAY" button starts reading the results achieved with the Click board™. The achieved results are displayed in the Application Output tab.
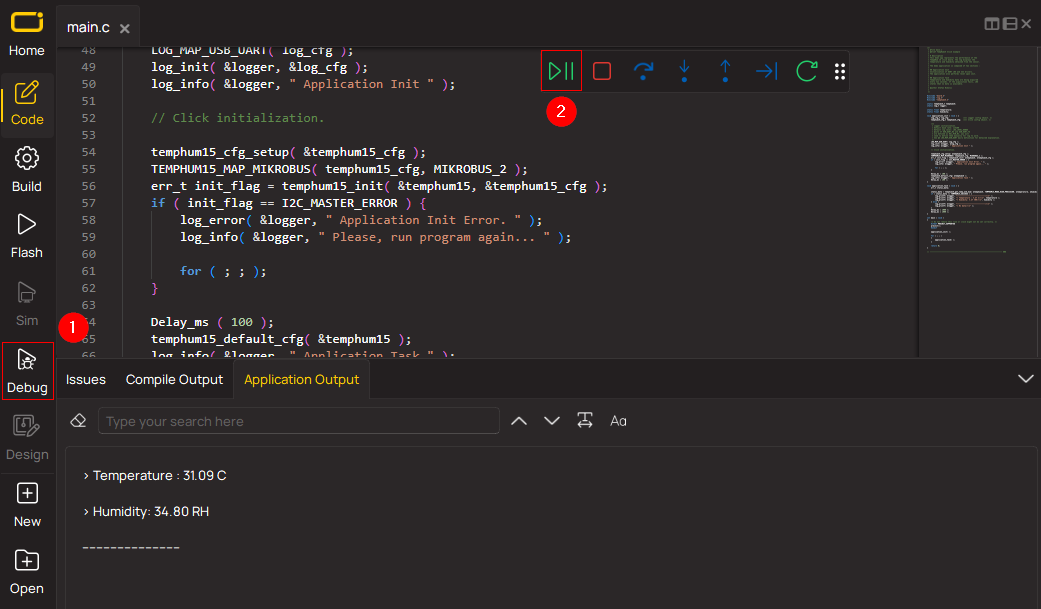
Software Support
Library Description
This library contains API for CapSense Click driver.
Key functions:
capsense_get_slider_lvl
- This function gets slider levelcapsense_read_data
- Read one byte from register addresscapsense_write_data
- Generic write data function
Open Source
Code example
This example can be found in NECTO Studio. Feel free to download the code, or you can copy the code below.
/*!
* \file
* \brief CapSense Click example
*
* # Description
* This demo example shows level of the slider on the terminal.
*
* The demo application is composed of two sections :
*
* ## Application Init
* Initializes device.
*
* ## Application Task
* Waits user to press top and bottom button to turn click's LEDs ON or OFF.
* User can swipe slider to send log to the UART where one can track their changes.
*
*
* \author MikroE Team
*
*/
// ------------------------------------------------------------------- INCLUDES
#include "board.h"
#include "log.h"
#include "capsense.h"
// ------------------------------------------------------------------ VARIABLES
static capsense_t capsense;
static log_t logger;
// ------------------------------------------------------- ADDITIONAL FUNCTIONS
void bits_to_str( uint8_t num, uint8_t *s )
{
uint8_t mask = 0x80;
while ( mask )
{
if ( num & mask )
{
*s++ = '1';
}
else
{
*s++ = '0';
}
mask >>= 1;
}
*s = '\0';
}
// ------------------------------------------------------ APPLICATION FUNCTIONS
void application_init ( void )
{
log_cfg_t log_cfg;
capsense_cfg_t cfg;
/**
* Logger initialization.
* Default baud rate: 115200
* Default log level: LOG_LEVEL_DEBUG
* @note If USB_UART_RX and USB_UART_TX
* are defined as HAL_PIN_NC, you will
* need to define them manually for log to work.
* See @b LOG_MAP_USB_UART macro definition for detailed explanation.
*/
LOG_MAP_USB_UART( log_cfg );
log_init( &logger, &log_cfg );
log_info( &logger, "---- Application Init ----" );
// Click initialization.
capsense_cfg_setup( &cfg );
CAPSENSE_MAP_MIKROBUS( cfg, MIKROBUS_1 );
capsense_init( &capsense, &cfg );
if ( CAPSENSE_ERROR == capsense_default_cfg ( &capsense ) )
{
log_error( &logger, " Default configuration." );
for ( ; ; );
}
log_info( &logger, " Application Task " );
}
void application_task ( void )
{
static uint8_t current_led_state = 0;
uint8_t output_lvl[ 10 ] = { 0 };
uint8_t button_select = 0;
uint8_t slider_lvl = 0;
capsense_read_data( &capsense, CAPSENSE_CS_READ_STATUS0, &button_select );
capsense_get_slider_lvl( &capsense, &slider_lvl );
capsense_write_data( &capsense, CAPSENSE_OUTPUT_PORT0, current_led_state );
Delay_ms( 100 );
if ( 8 == button_select )
{
current_led_state ^= 0x01;
log_printf( &logger, "Toggle LED1\r\n");
Delay_ms( 100 );
}
if ( 16 == button_select )
{
current_led_state ^= 0x02;
log_printf( &logger, "Toggle LED2\r\n");
Delay_ms( 100 );
}
if ( 24 == button_select )
{
current_led_state = ~current_led_state;
log_printf( &logger, "Toggle both LEDs\r\n");
Delay_ms( 100 );
}
if ( slider_lvl )
{
bits_to_str( slider_lvl, output_lvl );
log_printf( &logger, "Slider level - channels [5-1]:\t%s \r\n", &output_lvl[ 3 ] );
Delay_ms( 100 );
}
}
int main ( void )
{
application_init( );
for ( ; ; )
{
application_task( );
}
return 0;
}
// ------------------------------------------------------------------------ END