Ultra-low power consumption, fast erase times, and the ability to execute code directly from memory makes it an excellent choice for developers looking to optimize their projects for the edge of IoT networks
A
A
Hardware Overview
How does it work?
Flash 12 Click is based on the AT25EU0041A, a 4Mbit serial flash memory from Renesas, known for its ultra-low power consumption. This memory is part of a series specifically developed for applications operating on the IoT network's fringes, where energy efficiency is paramount. The AT25EU0041A is particularly well-suited for battery-operated systems, offering a reliable solution for both program code storage, which is then loaded into embedded systems, and serving as external RAM for direct program execution from the NOR Flash memory. Based on these features, this Click board™ is ideal for various applications from low-power reading and rapid erasure to boot/code shadow memory and straightforward event/data logging. The AT25EU0041A sets a new standard in energy efficiency through its innovative erase architecture, which features short erase times while maintaining low power consumption across all
operations, including reading, programming, and erasing. The consistent erase times, irrespective of the memory block size, and a page-erase capability that allows for as little as 256 bytes to be erased enhance the efficiency of write operations. Moreover, the AT25EU0041A adheres to the JEDEC standard for manufacturer and device identification, and it includes a 128-bit unique serial number, further enhancing its utility and security features. Flash 12 Click communicates with MCU through a standard SPI interface supporting the two most common SPI modes, SPI Mode 0 and 3, and a maximum clock frequency of up to 108MHz. The AT25EU0041A enhances data transfer rates through Dual and Quad SPI operations, which double and quadruple the standard SPI speed, respectively. These enhanced speeds are achieved by re-purposing the SI and SO pins as bidirectional I/O pins during Dual and Quad SPI operations.
Furthermore, the board features a HOLD function, marked as HLD and routed on the default position of the INT pin of the mikroBUS™ socket. The hold function allows the suspension of serial communications without disrupting ongoing operations. The board also has a Write Protect feature, marked as WP and routed on the default position of the PWM of the mikroBUS™ socket, that safeguards all registers and memory from unintended write operations through both hardware and software mechanisms. This Click board™ can be operated only with a 3.3V logic voltage level. The board must perform appropriate logic voltage level conversion before using MCUs with different logic levels. Also, it comes equipped with a library containing functions and an example code that can be used as a reference for further development.
Features overview
Development board
EasyAVR v7 is the seventh generation of AVR development boards specially designed for the needs of rapid development of embedded applications. It supports a wide range of 16-bit AVR microcontrollers from Microchip and has a broad set of unique functions, such as a powerful onboard mikroProg programmer and In-Circuit debugger over USB. The development board is well organized and designed so that the end-user has all the necessary elements in one place, such as switches, buttons, indicators, connectors, and others. With four different connectors for each port, EasyAVR v7 allows you to connect accessory boards, sensors, and custom electronics more
efficiently than ever. Each part of the EasyAVR v7 development board contains the components necessary for the most efficient operation of the same board. An integrated mikroProg, a fast USB 2.0 programmer with mikroICD hardware In-Circuit Debugger, offers many valuable programming/debugging options and seamless integration with the Mikroe software environment. Besides it also includes a clean and regulated power supply block for the development board. It can use a wide range of external power sources, including an external 12V power supply, 7-12V AC or 9-15V DC via DC connector/screw terminals, and a power source via the USB Type-B (USB-B)
connector. Communication options such as USB-UART and RS-232 are also included, alongside the well-established mikroBUS™ standard, three display options (7-segment, graphical, and character-based LCD), and several different DIP sockets which cover a wide range of 16-bit AVR MCUs. EasyAVR v7 is an integral part of the Mikroe ecosystem for rapid development. Natively supported by Mikroe software tools, it covers many aspects of prototyping and development thanks to a considerable number of different Click boards™ (over a thousand boards), the number of which is growing every day.
Microcontroller Overview
MCU Card / MCU
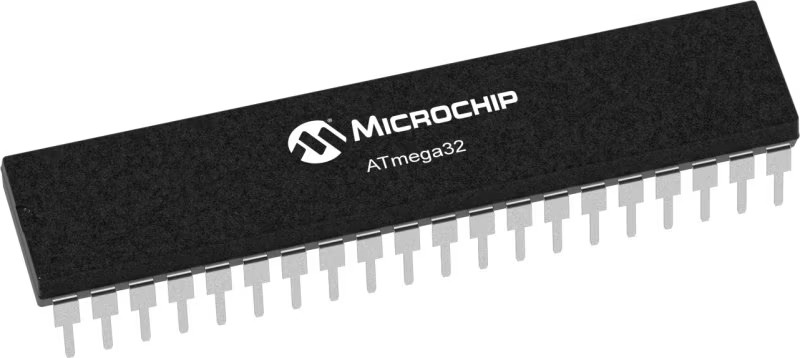
Architecture
AVR
MCU Memory (KB)
32
Silicon Vendor
Microchip
Pin count
40
RAM (Bytes)
2048
Used MCU Pins
mikroBUS™ mapper
Take a closer look
Click board™ Schematic
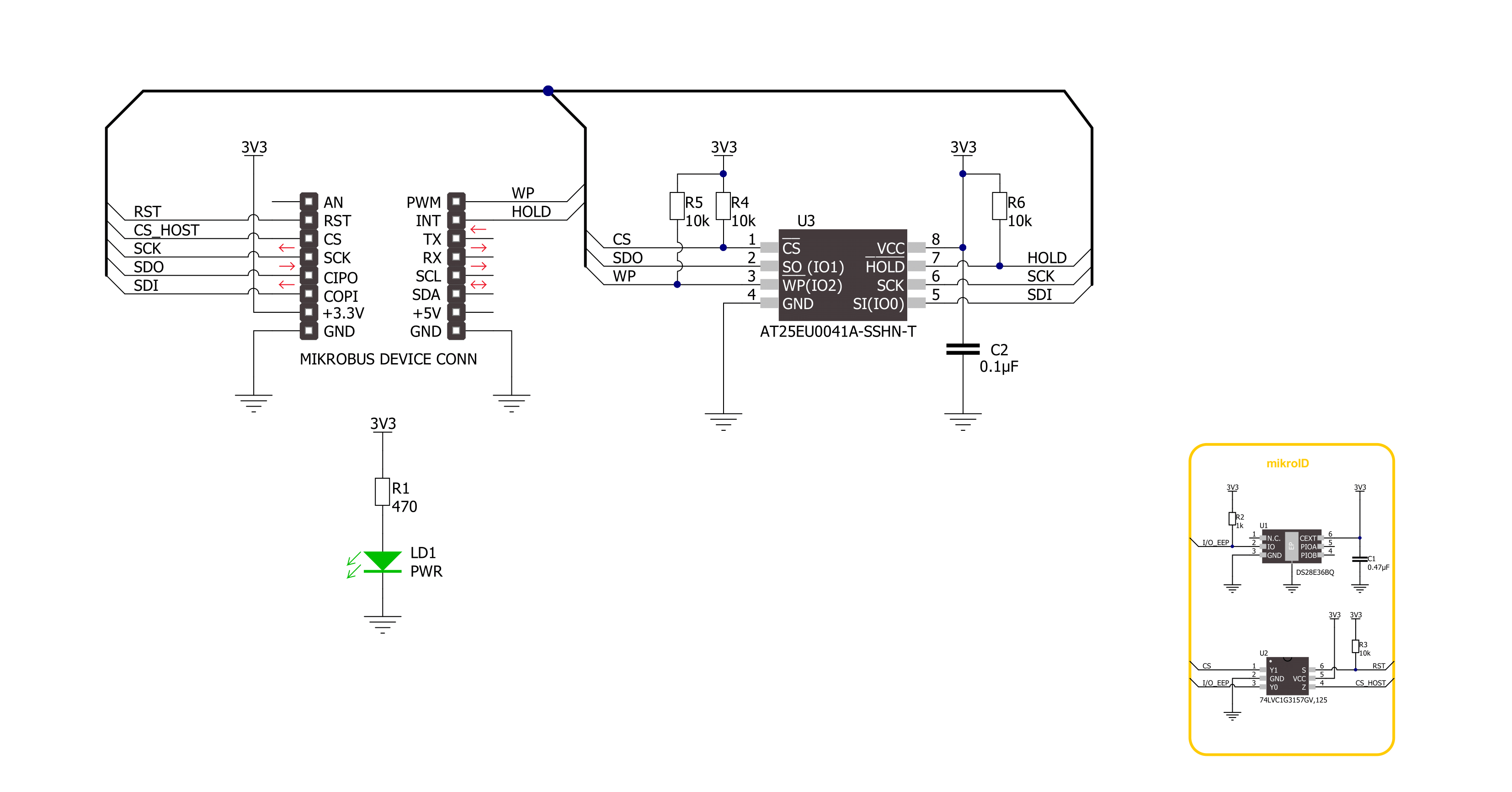
Step by step
Project assembly
Track your results in real time
Application Output
1. Application Output - In Debug mode, the 'Application Output' window enables real-time data monitoring, offering direct insight into execution results. Ensure proper data display by configuring the environment correctly using the provided tutorial.
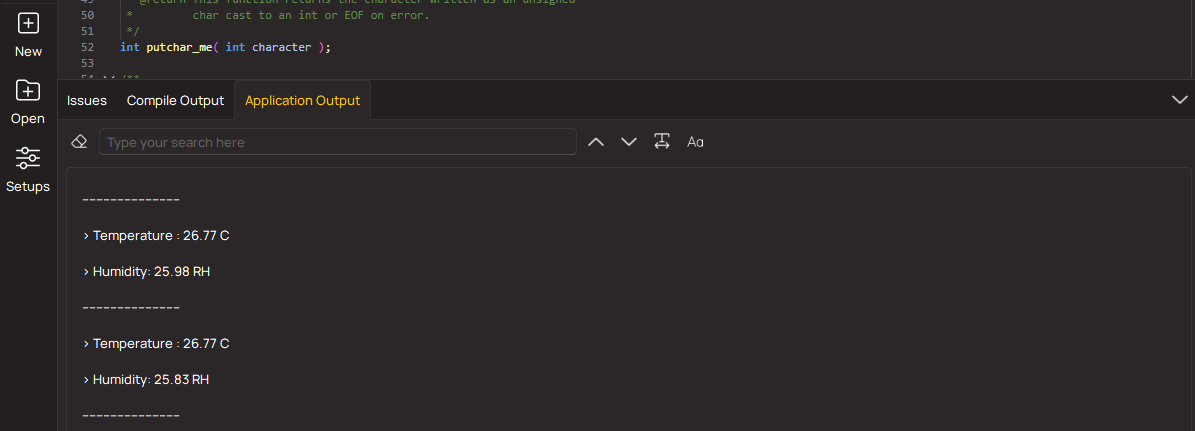
2. UART Terminal - Use the UART Terminal to monitor data transmission via a USB to UART converter, allowing direct communication between the Click board™ and your development system. Configure the baud rate and other serial settings according to your project's requirements to ensure proper functionality. For step-by-step setup instructions, refer to the provided tutorial.
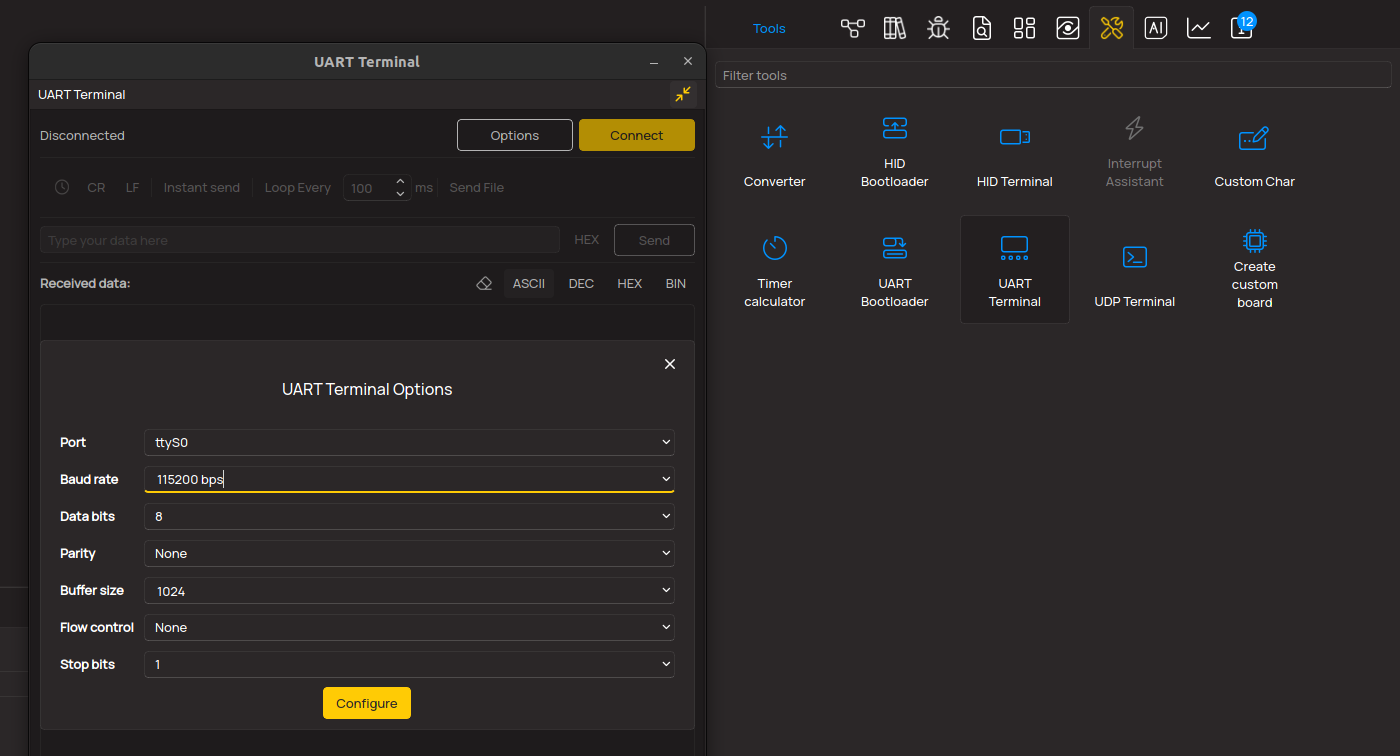
3. Plot Output - The Plot feature offers a powerful way to visualize real-time sensor data, enabling trend analysis, debugging, and comparison of multiple data points. To set it up correctly, follow the provided tutorial, which includes a step-by-step example of using the Plot feature to display Click board™ readings. To use the Plot feature in your code, use the function: plot(*insert_graph_name*, variable_name);. This is a general format, and it is up to the user to replace 'insert_graph_name' with the actual graph name and 'variable_name' with the parameter to be displayed.
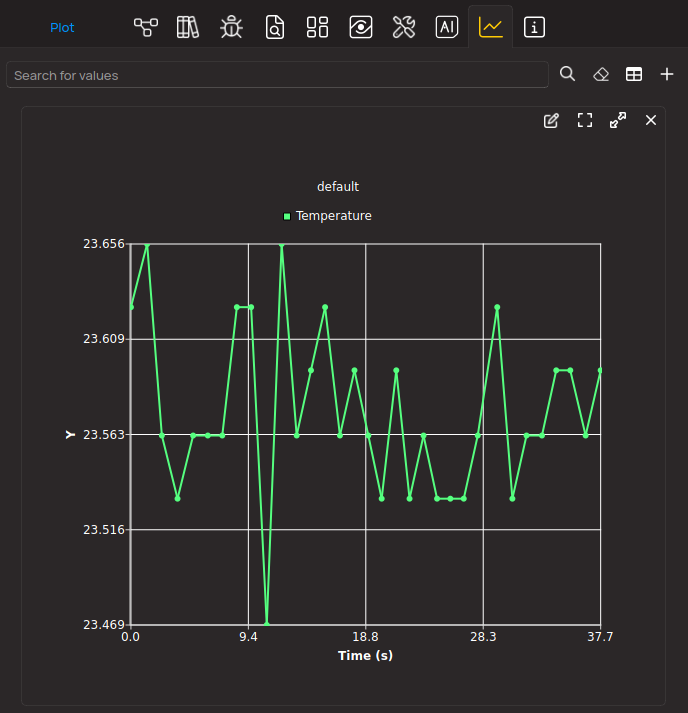
Software Support
Library Description
This library contains API for Flash 12 Click driver.
Key functions:
flash12_memory_write
- This function writes a desired number of data bytes starting to the selected memory address by using SPI serial interfaceflash12_memory_read
- This function reads a desired number of data bytes starting from the selected memory address by using SPI serial interfaceflash12_erase_memory
- This function erases the selected amount of memory which contains the selected address
Open Source
Code example
The complete application code and a ready-to-use project are available through the NECTO Studio Package Manager for direct installation in the NECTO Studio. The application code can also be found on the MIKROE GitHub account.
/*!
* @file main.c
* @brief Flash 12 Click example
*
* # Description
* This example demonstrates the use of Flash 12 click board
* by writing specified data to the memory and reading it back.
*
* The demo application is composed of two sections :
*
* ## Application Init
* The initialization of SPI module and log UART.
* After driver initialization, the app sets the default configuration.
*
* ## Application Task
* The demo application writes writes a desired number of bytes to the memory
* and then verifies if it is written correctly by reading
* from the same memory location and displaying the memory content.
* Results are being sent to the UART Terminal, where you can track their changes.
*
* @author Nenad Filipovic
*
*/
#include "board.h"
#include "log.h"
#include "flash12.h"
// Starting memory address
#define STARTING_ADDRESS 0x012345
// Demo text messages
#define DEMO_TEXT_MESSAGE_1 "MikroE"
#define DEMO_TEXT_MESSAGE_2 "Flash 12 Click"
static flash12_t flash12;
static log_t logger;
void application_init ( void )
{
log_cfg_t log_cfg; /**< Logger config object. */
flash12_cfg_t flash12_cfg; /**< Click config object. */
/**
* Logger initialization.
* Default baud rate: 115200
* Default log level: LOG_LEVEL_DEBUG
* @note If USB_UART_RX and USB_UART_TX
* are defined as HAL_PIN_NC, you will
* need to define them manually for log to work.
* See @b LOG_MAP_USB_UART macro definition for detailed explanation.
*/
LOG_MAP_USB_UART( log_cfg );
log_init( &logger, &log_cfg );
log_info( &logger, " Application Init " );
// Click initialization.
flash12_cfg_setup( &flash12_cfg );
FLASH12_MAP_MIKROBUS( flash12_cfg, MIKROBUS_1 );
if ( SPI_MASTER_ERROR == flash12_init( &flash12, &flash12_cfg ) )
{
log_error( &logger, " Communication init." );
for ( ; ; );
}
if ( FLASH12_ERROR == flash12_default_cfg ( &flash12 ) )
{
log_error( &logger, " Default configuration." );
for ( ; ; );
}
log_info( &logger, " Application Task\r\n" );
}
void application_task ( void )
{
uint8_t data_buf[ 128 ] = { 0 };
log_printf( &logger, " Memory address: 0x%.6LX\r\n", ( uint32_t ) STARTING_ADDRESS );
if ( FLASH12_OK == flash12_erase_memory( &flash12, FLASH12_CMD_BLOCK_ERASE_4KB, STARTING_ADDRESS ) )
{
log_printf( &logger, " Erase memory block (4KB)\r\n" );
}
memcpy( data_buf, DEMO_TEXT_MESSAGE_1, strlen( DEMO_TEXT_MESSAGE_1 ) );
if ( FLASH12_OK == flash12_memory_write( &flash12, STARTING_ADDRESS,
data_buf,
sizeof( data_buf ) ) )
{
log_printf( &logger, " Write data: %s\r\n", data_buf );
Delay_ms( 100 );
}
memset( data_buf, 0, sizeof( data_buf ) );
if ( FLASH12_OK == flash12_memory_read( &flash12, STARTING_ADDRESS,
data_buf,
sizeof( data_buf ) ) )
{
log_printf( &logger, " Read data: %s\r\n\n", data_buf );
Delay_ms( 3000 );
}
log_printf( &logger, " Memory address: 0x%.6LX\r\n", ( uint32_t ) STARTING_ADDRESS );
if ( FLASH12_OK == flash12_erase_memory( &flash12, FLASH12_CMD_BLOCK_ERASE_4KB, STARTING_ADDRESS ) )
{
log_printf( &logger, " Erase memory block (4KB)\r\n" );
}
memcpy( data_buf, DEMO_TEXT_MESSAGE_2, strlen( DEMO_TEXT_MESSAGE_2 ) );
if ( FLASH12_OK == flash12_memory_write( &flash12, STARTING_ADDRESS,
data_buf, sizeof( data_buf ) ) )
{
log_printf( &logger, " Write data: %s\r\n", data_buf );
Delay_ms( 100 );
}
memset( data_buf, 0, sizeof ( data_buf ) );
if ( FLASH12_OK == flash12_memory_read( &flash12, STARTING_ADDRESS,
data_buf, sizeof ( data_buf ) ) )
{
log_printf( &logger, " Read data: %s\r\n\n", data_buf );
Delay_ms( 3000 );
}
}
int main ( void )
{
application_init( );
for ( ; ; )
{
application_task( );
}
return 0;
}
// ------------------------------------------------------------------------ END