Dependable and long-lasting way to store information in electronic devices with enhanced write protection
A
A
Hardware Overview
How does it work?
EEPROM Click is based on the FT24C08A, 8Kb EEPROM with an I2C interface and Write Protection Mode from Fremont Micro Devices. The FT24C08A is organized as 1024 words of 8 bits (1 byte) each. The FT24C08A has 64 pages, respectively. Since each page has 16 bytes, random word addressing to FT24C08A will require 10 bits of data word addresses, respectively. It benefits from a wide power supply range and 100 years of data retention combining high reliability and lasting one million full-memory read/write/erase cycles. This Click board™ communicates with
MCU using the standard I2C 2-Wire interface with clock frequency that supports a Fast-Plus (1MHz) mode of operation. The FT24C08A also has a 7-bit slave address with the first five MSBs fixed to 1010. The address pins A0, A1, and A2 are programmed by the user and determine the value of the last three LSBs of the slave address, which can be selected by positioning onboard SMD jumpers labeled as ADDR SEL to an appropriate position marked as 0 or 1. Also, the configurable Write Protection function, labeled WP routed to the PWM pin of the mikroBUS™ socket, allows the
user to protect the whole EEPROM array from programming, thus protecting it from Write instructions. This Click board™ can operate with either 3.3V or 5V logic voltage levels selected via the VCC SEL jumper. This way, both 3.3V and 5V capable MCUs can use the communication lines properly. However, the Click board™ comes equipped with a library containing easy-to-use functions and an example code that can be used, as a reference, for further development.
Features overview
Development board
Curiosity PIC32 MZ EF development board is a fully integrated 32-bit development platform featuring the high-performance PIC32MZ EF Series (PIC32MZ2048EFM) that has a 2MB Flash, 512KB RAM, integrated FPU, Crypto accelerator, and excellent connectivity options. It includes an integrated programmer and debugger, requiring no additional hardware. Users can expand
functionality through MIKROE mikroBUS™ Click™ adapter boards, add Ethernet connectivity with the Microchip PHY daughter board, add WiFi connectivity capability using the Microchip expansions boards, and add audio input and output capability with Microchip audio daughter boards. These boards are fully integrated into PIC32’s powerful software framework, MPLAB Harmony,
which provides a flexible and modular interface to application development a rich set of inter-operable software stacks (TCP-IP, USB), and easy-to-use features. The Curiosity PIC32 MZ EF development board offers expansion capabilities making it an excellent choice for a rapid prototyping board in Connectivity, IOT, and general-purpose applications.
Microcontroller Overview
MCU Card / MCU
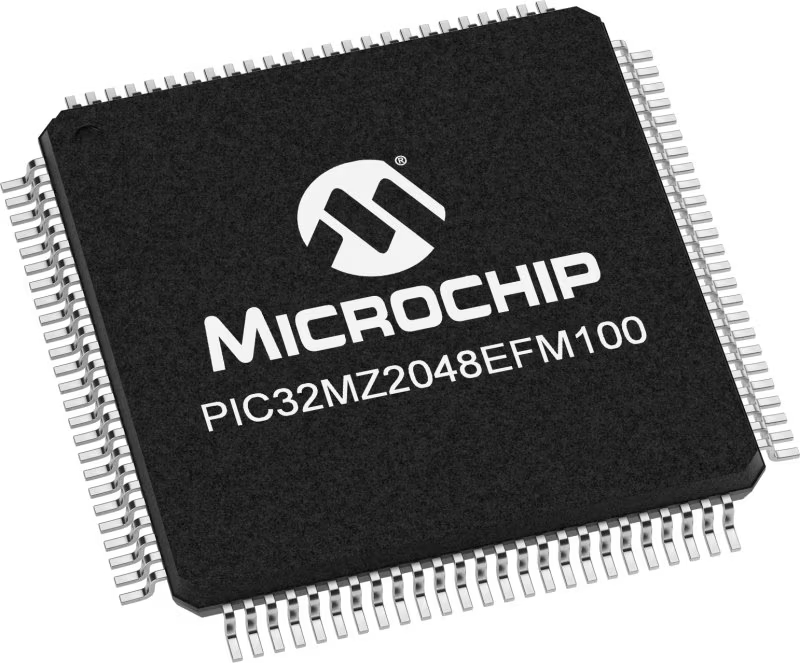
Architecture
PIC32
MCU Memory (KB)
2048
Silicon Vendor
Microchip
Pin count
100
RAM (Bytes)
524288
Used MCU Pins
mikroBUS™ mapper
Take a closer look
Schematic
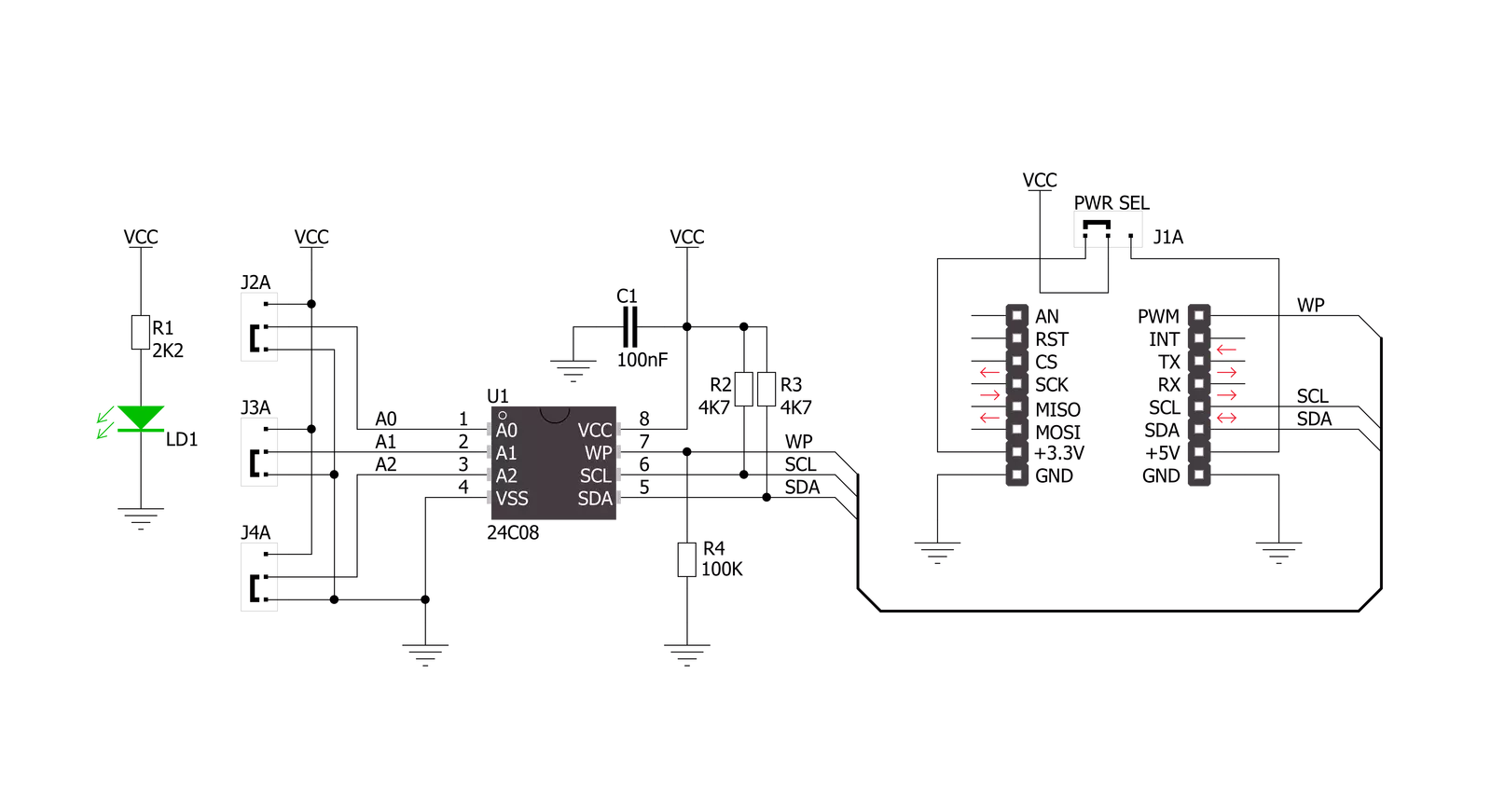
Step by step
Project assembly
Track your results in real time
Application Output via Debug Mode
1. Once the code example is loaded, pressing the "DEBUG" button initiates the build process, programs it on the created setup, and enters Debug mode.
2. After the programming is completed, a header with buttons for various actions within the IDE becomes visible. Clicking the green "PLAY" button starts reading the results achieved with the Click board™. The achieved results are displayed in the Application Output tab.
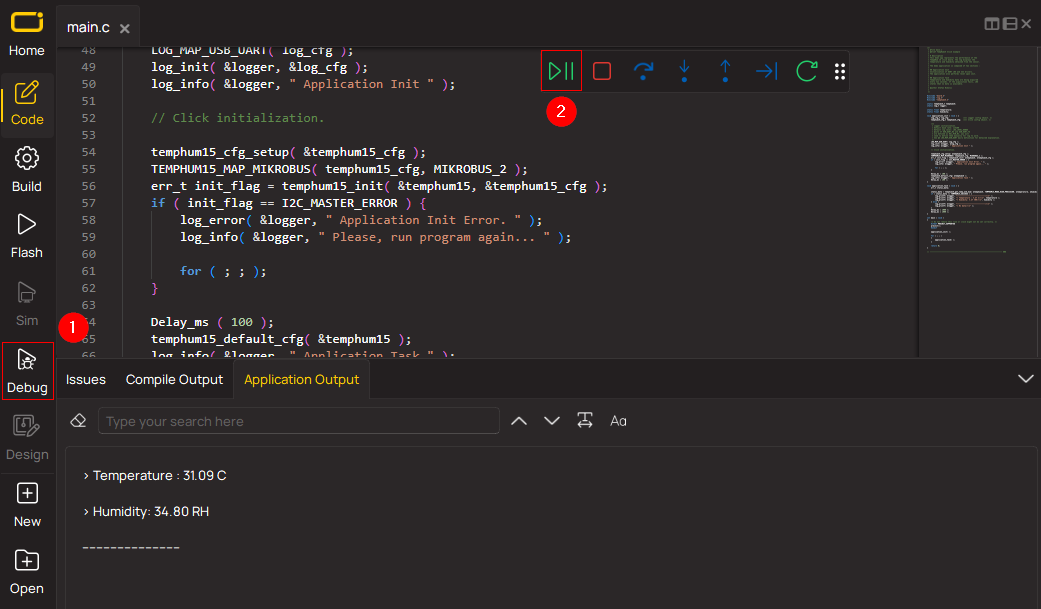
Software Support
Library Description
This library contains API for EEPROM Click driver.
Key functions:
eeprom_write_page
- Page Write functioneeprom_read_sequential
- Sequential Read functioneeprom_write_protect
- Write Protect function
Open Source
Code example
This example can be found in NECTO Studio. Feel free to download the code, or you can copy the code below.
/*!
* \file main.c
* \brief Eeprom Click example
*
* # Description
* This is a example which demonstrates the use of EEPROM Click board.
*
* The demo application is composed of two sections :
*
* ## Application Init
* Initializes peripherals and pins used by EEPROM Click.
* Initializes SPI serial interface and puts a device to the initial state.
*
* ## Application Task
* First page of memory block 1 will be written with data values starting from
* 1 to 16. This memory page will be read by the user, to verify successfully
* data writing. Data writing to memory will be protected upon memory writing,
* and before memory reading.
*
* \author Nemanja Medakovic
*
*/
// ------------------------------------------------------------------- INCLUDES
#include <string.h>
#include "board.h"
#include "log.h"
#include "eeprom.h"
// ------------------------------------------------------------------ VARIABLES
static eeprom_t eeprom;
static log_t logger;
// ------------------------------------------------------ APPLICATION FUNCTIONS
void application_init( void )
{
eeprom_cfg_t eeprom_cfg;
log_cfg_t log_cfg;
// Click initialization.
eeprom_cfg_setup( &eeprom_cfg );
EEPROM_MAP_MIKROBUS( eeprom_cfg, MIKROBUS_1 );
eeprom_init( &eeprom, &eeprom_cfg );
/**
* Logger initialization.
* Default baud rate: 115200
* Default log level: LOG_LEVEL_DEBUG
* @note If USB_UART_RX and USB_UART_TX
* are defined as HAL_PIN_NC, you will
* need to define them manually for log to work.
* See @b LOG_MAP_USB_UART macro definition for detailed explanation.
*/
LOG_MAP_USB_UART( log_cfg );
log_init( &logger, &log_cfg );
log_info( &logger, "---- Application Init ----" );
}
void application_task( void )
{
uint8_t transfer_data[ EEPROM_NBYTES_PAGE ];
uint8_t read_buff[ EEPROM_NBYTES_PAGE ] = { 0 };
uint8_t cnt;
uint8_t tmp = EEPROM_BLOCK_ADDR_START;
transfer_data[ EEPROM_BLOCK_ADDR_START ] = 1;
for (cnt = EEPROM_BLOCK_ADDR_START + 1; cnt < EEPROM_NBYTES_PAGE; cnt++)
{
transfer_data[ cnt ] = transfer_data[ cnt - 1 ] + 1;
}
eeprom_write_enable( &eeprom );
eeprom_write_page( &eeprom, tmp, transfer_data );
eeprom_write_protect( &eeprom );
Delay_ms( 1000 );
memset( transfer_data, 0, sizeof(transfer_data) );
eeprom_read_sequential( &eeprom, EEPROM_BLOCK_ADDR_START, EEPROM_NBYTES_PAGE, read_buff );
for (cnt = EEPROM_BLOCK_ADDR_START; cnt < EEPROM_NBYTES_PAGE; cnt++)
{
log_printf( &logger, " %u", ( uint16_t )read_buff[ cnt ] );
Delay_ms( 300 );
}
log_printf( &logger, "\r\n" );
}
void main( void )
{
application_init( );
for ( ; ; )
{
application_task( );
}
}
// ------------------------------------------------------------------------ END